published: 03 Apr 2023
5 min read | website: https://gabrieleromanato.name/nodejs-renaming-files-recursively
Node.js is a popular JavaScript runtime environment that has transformed the way we build server-side applications. One of its many strengths is the ability to manipulate the file system with ease. In this article, we will discuss how to use Node.js to rename files and folders. We will provide step-by-step instructions and code snippets to help you understand and implement these operations in your projects.
Before we begin, make sure you have Node.js installed on your system. If you don't have it yet, visit the official Node.js website (https://nodejs.org/) to download and install the latest version. Once installed, you can proceed with the following examples.
To rename files with Node.js, we will use the fs module and the path module, which is built-in and provides an API for interacting with the file system.
The requirement were:
* spaces and underscores was to be replaced with a dash
* parentheses to be removed.
* recursively replace the filenames
'use strict'; const path = require('path'); const fs = require('fs'); const listDir = (dir, fileList = []) => { let files = fs.readdirSync(dir); files.forEach(file => { if (fs.statSync(path.join(dir, file)).isDirectory()) { fileList = listDir(path.join(dir, file), fileList); } else { let ext = path.parse(file).ext; let name = path.parse(file).name; name = name.replace(/[\s_]/g, '-').replace(/[()]/g, '') + "" + ext; name = name.toLowerCase(); let src = path.join(dir, file); let newSrc = path.join(dir, name); fileList.push({ oldSrc: src, newSrc: newSrc }); } }); return fileList; }; let foundFiles = listDir('./parentFolder'); foundFiles.forEach(f => { fs.renameSync(f.oldSrc, f.newSrc); console.log('renaming from', f.oldSrc, "to", f.newSrc); });
Instructions:
1. Replace the ./parentFolder with the name of the folder containing the files.
2. Paste the code into a file named renameFiles.cjs
3. Run the code at the command line "node renameFiles.cjs"
Credit is given to Gabriele Romanato since he provided the basis of this code. See https://gabrieleromanato.name/nodejs-renaming-files-recursively
The code was slightly altered to accommodate folders.
'use strict'; const path = require('path'); const fs = require('fs'); function renameFoldersRecursively(dirPath) { fs.readdir(dirPath, { withFileTypes: true }, (error, items) => { if (error) { console.error('Error reading directory:', error); return; } items.forEach(item => { if (item.isDirectory()) { const oldFolderPath = path.join(dirPath, item.name); const newFolderPath = path.join(dirPath, item.name.replace(/[\s_]/g, '-').replace(/[()]/g, '')); fs.rename(oldFolderPath, newFolderPath, error => { if (error) { console.error('Error renaming folder:', error); return; } console.log(`Renamed folder: ${oldFolderPath} -> ${newFolderPath}`); renameFoldersRecursively(newFolderPath); }); } }); }); } renameFoldersRecursively('./parentFolder');
Instructions:
1. Replace the ./parentFolder with the name of the folder containing the files.
2. Paste the code into a file named renameFolders.cjs
3. Run the code at the command line "node renameFolders.cjs"
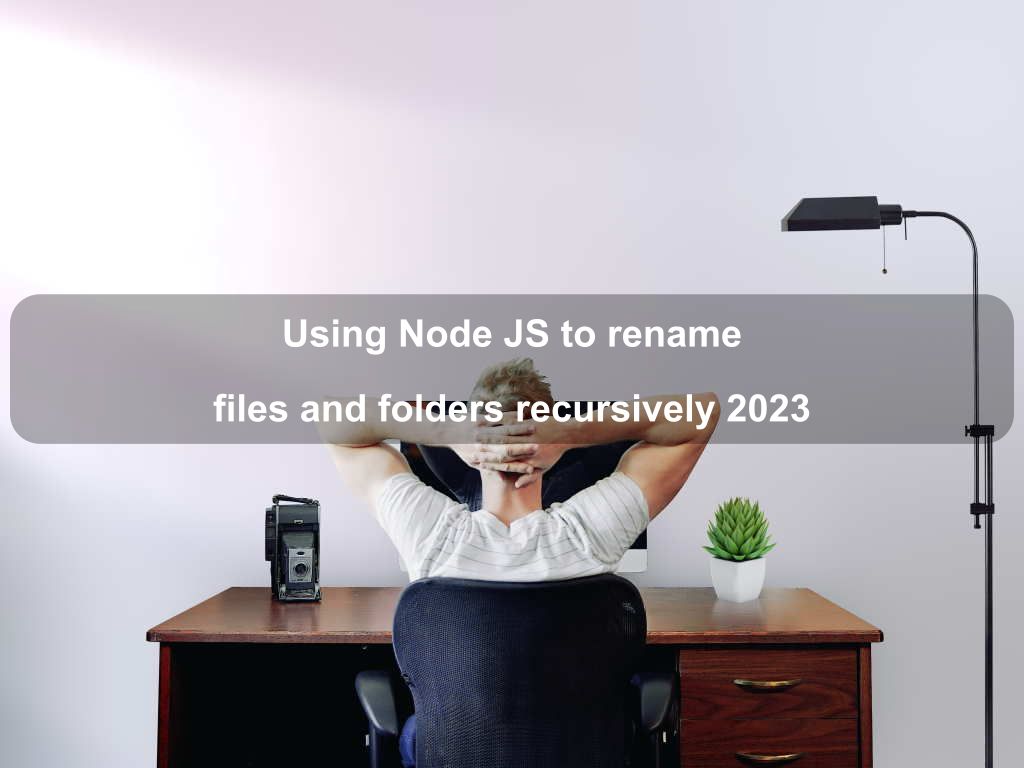
Are we missing something? Help us improve this article. Reach out to us.
Node.js is a popular JavaScript runtime environment that has transformed the way we build server-side applications. One of its many strengths is the ability to manipulate the file system with ease. In this article, we will discuss how to use Node.js to rename files and folders. We will provide step-by-step instructions and code snippets to help you understand and implement these operations in your projects.
Before we begin, make sure you have Node.js installed on your system. If you don't have it yet, visit the official Node.js website (https://nodejs.org/) to download and install the latest version. Once installed, you can proceed with the following examples.
To rename files with Node.js, we will use the fs module and the path module, which is built-in and provides an API for interacting with the file system.
The requirement were:
* spaces and underscores was to be replaced with a dash
* parentheses to be removed.
* recursively replace the filenames
'use strict'; const path = require('path'); const fs = require('fs'); const listDir = (dir, fileList = []) => { let files = fs.readdirSync(dir); files.forEach(file => { if (fs.statSync(path.join(dir, file)).isDirectory()) { fileList = listDir(path.join(dir, file), fileList); } else { let ext = path.parse(file).ext; let name = path.parse(file).name; name = name.replace(/[\s_]/g, '-').replace(/[()]/g, '') + "" + ext; name = name.toLowerCase(); let src = path.join(dir, file); let newSrc = path.join(dir, name); fileList.push({ oldSrc: src, newSrc: newSrc }); } }); return fileList; }; let foundFiles = listDir('./parentFolder'); foundFiles.forEach(f => { fs.renameSync(f.oldSrc, f.newSrc); console.log('renaming from', f.oldSrc, "to", f.newSrc); });
Instructions:
1. Replace the ./parentFolder with the name of the folder containing the files.
2. Paste the code into a file named renameFiles.cjs
3. Run the code at the command line "node renameFiles.cjs"
Credit is given to Gabriele Romanato since he provided the basis of this code. See https://gabrieleromanato.name/nodejs-renaming-files-recursively
The code was slightly altered to accommodate folders.
'use strict'; const path = require('path'); const fs = require('fs'); function renameFoldersRecursively(dirPath) { fs.readdir(dirPath, { withFileTypes: true }, (error, items) => { if (error) { console.error('Error reading directory:', error); return; } items.forEach(item => { if (item.isDirectory()) { const oldFolderPath = path.join(dirPath, item.name); const newFolderPath = path.join(dirPath, item.name.replace(/[\s_]/g, '-').replace(/[()]/g, '')); fs.rename(oldFolderPath, newFolderPath, error => { if (error) { console.error('Error renaming folder:', error); return; } console.log(`Renamed folder: ${oldFolderPath} -> ${newFolderPath}`); renameFoldersRecursively(newFolderPath); }); } }); }); } renameFoldersRecursively('./parentFolder');
Instructions:
1. Replace the ./parentFolder with the name of the folder containing the files.
2. Paste the code into a file named renameFolders.cjs
3. Run the code at the command line "node renameFolders.cjs"
Are you looking for other code tips?
TipsAndTricksta
I love coding, I love coding tips & tricks, code snippets and javascript