published: 17 Sep 2022
2 min read
Update the HTML markup of a DOM element with JavaScript
In JavaScript, the HTML element's innerHTML
property can be used to set or get the HTML markup within the element. This property returns a DOM string containing the HTML serialization of all child elements.
If you set a new value of innerHTML
, it will remove all the element's child nodes and replace them with HTML nodes created by parsing the HTML string.
Let us say we have the following <div>
element somewhere in the document:
<div id='hint'>
<p>Learn JavaScript for free!</p>
</div>
To get the current HTML markup of the above HTML, use the following JavaScript:
// grab element
const div = document.querySelector('#hint')
// get current HTML markup
console.log(div.innerHTML)
// <p>Learn JavaScript for free!</p>
To replace the existing HTML markup completely, simply set a new value of innerHTML
like below:
// replace existing markup
div.innerHTML = 'span>Hey, there!</span>'
To add new elements before the current HTML markup, do the following:
div.innerHTML = 'span>Hey, there!</span>' + div.innerHTML
To append HTML contents after the existing markup:
div.innerHTML += 'span>Hey, there!</span>'
The new markup can be any valid HTML string, including simple plain text:
div.innerHTML = 'This is plain text'
Read Next: How to create a DOM element using JavaScript
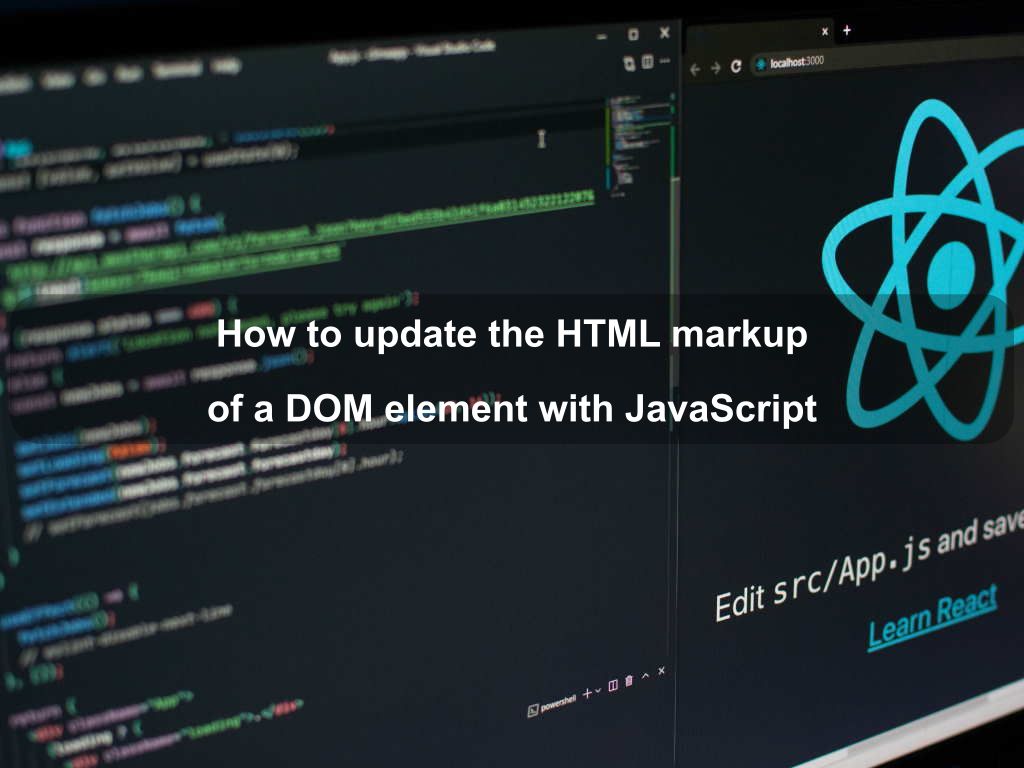
Are we missing something? Help us improve this article. Reach out to us.
Update the HTML markup of a DOM element with JavaScript
In JavaScript, the HTML element's innerHTML
property can be used to set or get the HTML markup within the element. This property returns a DOM string containing the HTML serialization of all child elements.
If you set a new value of innerHTML
, it will remove all the element's child nodes and replace them with HTML nodes created by parsing the HTML string.
Let us say we have the following <div>
element somewhere in the document:
<div id='hint'>
<p>Learn JavaScript for free!</p>
</div>
To get the current HTML markup of the above HTML, use the following JavaScript:
// grab element
const div = document.querySelector('#hint')
// get current HTML markup
console.log(div.innerHTML)
// <p>Learn JavaScript for free!</p>
To replace the existing HTML markup completely, simply set a new value of innerHTML
like below:
// replace existing markup
div.innerHTML = 'span>Hey, there!</span>'
To add new elements before the current HTML markup, do the following:
div.innerHTML = 'span>Hey, there!</span>' + div.innerHTML
To append HTML contents after the existing markup:
div.innerHTML += 'span>Hey, there!</span>'
The new markup can be any valid HTML string, including simple plain text:
div.innerHTML = 'This is plain text'
Read Next: How to create a DOM element using JavaScript
Are you looking for other code tips?
JS Nooby
Javascript connoisseur