published: 01 Apr 2022
2 min read
Understanding Array.from() Method in JavaScript
The Array.from()
method in JavaScript creates a new, shallow-copied instance of Array
from an array-like or iterable object. You can use this method to convert array-like objects (objects with a length
property and indexed items) as well as iterable objects (objects such as Map and Set) to an array.
Syntax
Here is the syntax of Array.from()
method:
Array.from(arrayLikeOrIterable[, mapFunction[, thisArg]])
arrayLikeOrIterable
- An array-like or an iterable object that you want to convert to an array.mapFunction(item, index) {...}
- An optional map function to call on every element in the collection. This method is extremely useful when you want to convert and transform an array-like or iterable object to an array at the same time.thisArg
- The value ofthisArg
is used asthis
while invokingmapFunction
.
The Array.from()
method returns new Array
instance. When you pass mapFunction
to Array.from()
, it executes a map() function on each element of the newly created array.
In short, Array.from(obj, mapFunction, thisArg)
has the same result as Array.from(obj).map(mapFunction, thisArg)
, except that it does not create an intermediate array.
Array from a String
The Array.from()
can be used to convert a string to an array in JavaScript. Each alphabet of the string is converted to an element of the new array instance:
const str = 'Apple';
const arr = Array.from(str); // same as str.split('')
console.log(arr);
// ['A', 'p', 'p', 'l', 'e']
Array from a Set
A Set is a special type of object introduced in ES6 that allows us to create a collection of unique values. To convert a Set
to an array of unique values, you can use the Array.from()
method:
const set = new Set(['🐦', '🦉', '🦆', '🦅']);
const birds = Array.from(set);
console.log(birds);
// ['🐦', '🦉', '🦆', '🦅']
You can also use the above code to remove duplicates from an array:
function unique(arr) {
return Array.from(new Set(arr));
};
unique([1, 2, 2, 3, 4, 4]);
// [1, 2, 3, 4]
Array from a Map
A Map is another ES6 data structure that lets you create a collection of key-value pairs. Here is an example that converts an instance of Map
to an array by using Array.from()
method:
const map = new Map([
['🍌', 'Banana'],
['🍕', 'Pizza'],
['🥒', 'Cucumber'],
['🌽', 'Maize'],
]);
const foods = Array.from(map);
console.log(foods);
// [['🍌', 'Banana'], ['🍕', 'Pizza'], ['🥒', 'Cucumber'], ['🌽', 'Maize']]
Array from a NodeList
A NodeList is an array-like object that contains a collection of DOM elements or more specifically nodes. Without converting a NodeList
object to an array, you can not use the common array methods like map()
, slice()
, and filter()
on it.
To convert a NodeList
to an array, you can use the Array.from()
method:
const divs = document.querySelectorAll('div');
const divsArr = Array.from(divs);
// now you can use array methods
divsArr.map(div => console.log(div.innerText));
Take a look at this article to learn more about NodeList
to array conversion.
Array from an arguments
The arguments
object is an array-like object accessible inside all non-arrow functions that contains the values of the arguments passed to that function.
To convert the arguments
object to a truly array, you can use the Array.from()
method:
function numbers() {
const args = Array.from(arguments);
console.log(args);
}
numbers(1, 2, 3, 4, 5);
// [1, 2, 3, 4, 5]
Cloning an array
In an earlier article, we looked at several ways to create a clone of an array. One of them is Array.from()
that creates a shallow-copy of an existing array:
const fruits = ['🍑', '🍓', '🍉', '🍇'];
const moreFruits = Array.from(fruits);
console.log(moreFruits);
// ['🍑', '🍓', '🍉', '🍇']
To create a deep clone of an array, take a look at this guide.
Initializing an array
The Array.from()
method is also helpful when you want to initialize an array with the same values or objects:
const length = 4;
// init array with 0
Array.from({ length }, () => 0);
// [0, 0, 0, 0]
// init array with random values
Array.from({ length }, () => Math.floor(Math.random() * 10) + 1);
// [2, 4, 2, 10]
// init array with objects
Array.from({ length }, () => ({}));
// [{}, {}, {}, {}]
Generating a range of numbers
You can also use the Array.from()
method to generate a sequence of numbers in JavaScript:
const range = (stop) => {
return Array.from({ length: stop }, (item, index) => index);
}
range(5); // [0, 1, 2, 3, 5]
Browser compatibility
Since Array.from()
is part of ES6, it only works in modern browsers. To support older browsers like IE, you can use a polyfill available on MDN.
To learn more about JavaScript arrays and how to use them to store multiple pieces of information in one single variable, take a look at this article.
Read Next: Understanding Object.assign() Method in JavaScript
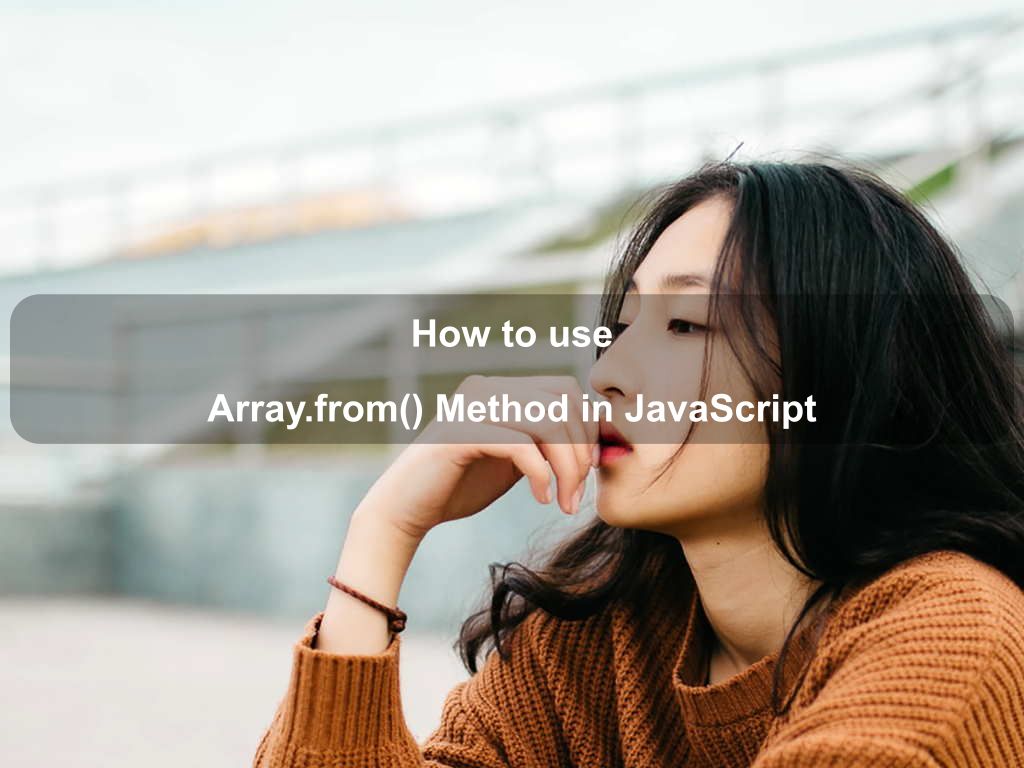
Are we missing something? Help us improve this article. Reach out to us.
Understanding Array.from() Method in JavaScript
The Array.from()
method in JavaScript creates a new, shallow-copied instance of Array
from an array-like or iterable object. You can use this method to convert array-like objects (objects with a length
property and indexed items) as well as iterable objects (objects such as Map and Set) to an array.
Syntax
Here is the syntax of Array.from()
method:
Array.from(arrayLikeOrIterable[, mapFunction[, thisArg]])
arrayLikeOrIterable
- An array-like or an iterable object that you want to convert to an array.mapFunction(item, index) {...}
- An optional map function to call on every element in the collection. This method is extremely useful when you want to convert and transform an array-like or iterable object to an array at the same time.thisArg
- The value ofthisArg
is used asthis
while invokingmapFunction
.
The Array.from()
method returns new Array
instance. When you pass mapFunction
to Array.from()
, it executes a map() function on each element of the newly created array.
In short, Array.from(obj, mapFunction, thisArg)
has the same result as Array.from(obj).map(mapFunction, thisArg)
, except that it does not create an intermediate array.
Array from a String
The Array.from()
can be used to convert a string to an array in JavaScript. Each alphabet of the string is converted to an element of the new array instance:
const str = 'Apple';
const arr = Array.from(str); // same as str.split('')
console.log(arr);
// ['A', 'p', 'p', 'l', 'e']
Array from a Set
A Set is a special type of object introduced in ES6 that allows us to create a collection of unique values. To convert a Set
to an array of unique values, you can use the Array.from()
method:
const set = new Set(['🐦', '🦉', '🦆', '🦅']);
const birds = Array.from(set);
console.log(birds);
// ['🐦', '🦉', '🦆', '🦅']
You can also use the above code to remove duplicates from an array:
function unique(arr) {
return Array.from(new Set(arr));
};
unique([1, 2, 2, 3, 4, 4]);
// [1, 2, 3, 4]
Array from a Map
A Map is another ES6 data structure that lets you create a collection of key-value pairs. Here is an example that converts an instance of Map
to an array by using Array.from()
method:
const map = new Map([
['🍌', 'Banana'],
['🍕', 'Pizza'],
['🥒', 'Cucumber'],
['🌽', 'Maize'],
]);
const foods = Array.from(map);
console.log(foods);
// [['🍌', 'Banana'], ['🍕', 'Pizza'], ['🥒', 'Cucumber'], ['🌽', 'Maize']]
Array from a NodeList
A NodeList is an array-like object that contains a collection of DOM elements or more specifically nodes. Without converting a NodeList
object to an array, you can not use the common array methods like map()
, slice()
, and filter()
on it.
To convert a NodeList
to an array, you can use the Array.from()
method:
const divs = document.querySelectorAll('div');
const divsArr = Array.from(divs);
// now you can use array methods
divsArr.map(div => console.log(div.innerText));
Take a look at this article to learn more about NodeList
to array conversion.
Array from an arguments
The arguments
object is an array-like object accessible inside all non-arrow functions that contains the values of the arguments passed to that function.
To convert the arguments
object to a truly array, you can use the Array.from()
method:
function numbers() {
const args = Array.from(arguments);
console.log(args);
}
numbers(1, 2, 3, 4, 5);
// [1, 2, 3, 4, 5]
Cloning an array
In an earlier article, we looked at several ways to create a clone of an array. One of them is Array.from()
that creates a shallow-copy of an existing array:
const fruits = ['🍑', '🍓', '🍉', '🍇'];
const moreFruits = Array.from(fruits);
console.log(moreFruits);
// ['🍑', '🍓', '🍉', '🍇']
To create a deep clone of an array, take a look at this guide.
Initializing an array
The Array.from()
method is also helpful when you want to initialize an array with the same values or objects:
const length = 4;
// init array with 0
Array.from({ length }, () => 0);
// [0, 0, 0, 0]
// init array with random values
Array.from({ length }, () => Math.floor(Math.random() * 10) + 1);
// [2, 4, 2, 10]
// init array with objects
Array.from({ length }, () => ({}));
// [{}, {}, {}, {}]
Generating a range of numbers
You can also use the Array.from()
method to generate a sequence of numbers in JavaScript:
const range = (stop) => {
return Array.from({ length: stop }, (item, index) => index);
}
range(5); // [0, 1, 2, 3, 5]
Browser compatibility
Since Array.from()
is part of ES6, it only works in modern browsers. To support older browsers like IE, you can use a polyfill available on MDN.
To learn more about JavaScript arrays and how to use them to store multiple pieces of information in one single variable, take a look at this article.
Read Next: Understanding Object.assign() Method in JavaScript
Are you looking for other code tips?
JS Nooby
Javascript connoisseur