published: 06 Sep 2022
2 min read
Storing and retrieving objects in local storage using JavaScript
The HTML web storage API offers a way to store a large amount of data (5MB+) in a user's browser without affecting the website performance.
By default, both web storage objects - localStorage
and sessionStorage
- allows us to store only string key-value pairs:
const user = { name: 'Alex', job: 'Software Engineer' };
localStorage.setItem('user', user);
console.log(localStorage.getItem('user')); // [object Object]
If you want to store an entire JavaScript object, you first need to serialize it into a string by using the JSON.stringify()
method:
const user = { name: 'Alex', job: 'Software Engineer' };
localStorage.setItem('user', JSON.stringify(user));
console.log(localStorage.getItem('user'));
// {'name':'Alex','job':'Software Engineer'}
To retrieve the JavaScript object from localStorage
, use the getItem()
method. You still need to use the JSON.parse()
method to parse the JSON string back to an object:
// Retrieve the JSON string
const userStr = localStorage.getItem('user');
// Parse JSON string to object
const userObj = JSON.parse(userStr);
// Print object attributes
console.log(userObj.name); // Alex
console.log(userObj.job); // Software Engineer
To learn more about localStorage
and sessionStorage
objects, take a look at this article.
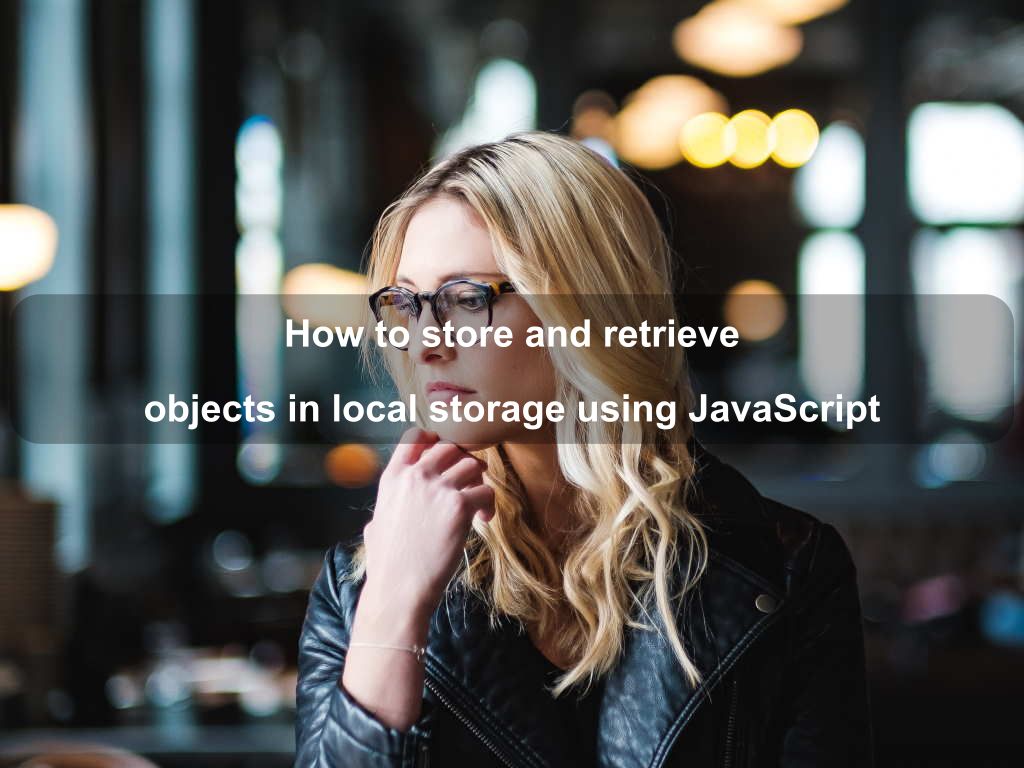
Are we missing something? Help us improve this article. Reach out to us.
Storing and retrieving objects in local storage using JavaScript
The HTML web storage API offers a way to store a large amount of data (5MB+) in a user's browser without affecting the website performance.
By default, both web storage objects - localStorage
and sessionStorage
- allows us to store only string key-value pairs:
const user = { name: 'Alex', job: 'Software Engineer' };
localStorage.setItem('user', user);
console.log(localStorage.getItem('user')); // [object Object]
If you want to store an entire JavaScript object, you first need to serialize it into a string by using the JSON.stringify()
method:
const user = { name: 'Alex', job: 'Software Engineer' };
localStorage.setItem('user', JSON.stringify(user));
console.log(localStorage.getItem('user'));
// {'name':'Alex','job':'Software Engineer'}
To retrieve the JavaScript object from localStorage
, use the getItem()
method. You still need to use the JSON.parse()
method to parse the JSON string back to an object:
// Retrieve the JSON string
const userStr = localStorage.getItem('user');
// Parse JSON string to object
const userObj = JSON.parse(userStr);
// Print object attributes
console.log(userObj.name); // Alex
console.log(userObj.job); // Software Engineer
To learn more about localStorage
and sessionStorage
objects, take a look at this article.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur