published: 05 Jul 2022
2 min read
Object.entries() and Object.values() methods in JavaScript
The Object.entries()
and Object.values()
methods were added to the JavaScript object constructor with the release of ECMAScript 2017 (ES8).
Let us have a quick look at these useful methods.
Object.entries()
Method
The Object.entries()
method takes an object as argument and returns an array with arrays of key-value pairs:
const birds = {
owl: '🦉',
eagle: '🦅',
duck: '🦆'
}
const entries = Object.entries(birds)
console.log(entries)
// [['owl', '🦉'], ['eagle', '🦅'], ['duck', '🦆']]
The order of the array element does not depend on how the object was defined. The order is the same as that provided by a for...in
loop.
Iterate over an object
The Object.entries()
method can be used to iterate over an object:
// using 'for...of' loop
for (const [key, value] of Object.entries(birds)) {
console.log('${key}: ${value}')
}
// owl: 🦉
// eagle: 🦅
// duck: 🦆
// using array destructuring
Object.entries(birds).forEach(([key, value]) => console.log('${key}: ${value}'))
// owl: 🦉
// eagle: 🦅
// duck: 🦆
Convert an object to a Map
Since the Map
constructor takes an iterable to initialize a map object, the Object.entries()
method can be used to create a map from an object:
const map = new Map(Object.entries(birds))
console.log(map.size) // 3
console.log(map.has('owl')) // true
console.log(map.get('duck')) // 🦆
Object.values()
Method
The Object.values()
method, unlike Object.entries()
, only returns the values of the own enumerable string-keyed properties in the same order as provided by the for...in
loop:
const foods = {
cake: '🍰',
pizza: '🍕',
candy: '🍬',
icecream: '🍨'
}
const values = Object.values(foods)
console.log(values)
// ['🍰', '🍕', '🍬', '🍨']
Both Object.values()
and Object.entries()
do not follow the prototype chain and only iterate through the properties directly added to the given object.
They also ignore all non-enumerable properties:
Object.defineProperty(foods, 'sushi', {
value: '🍣',
writable: true,
configurable: true,
enumerable: false
})
console.log(Object.values(foods))
// ['🍰', '🍕', '🍬', '🍨']
Convert an object to a Set
Since the Set
constructor accepts an iterable, with Object.values()
, we can easily convert an object to a Set
:
const set = new Set(Object.values(foods))
console.log(set.size) // 4
console.log(set.has('🍨')) // true
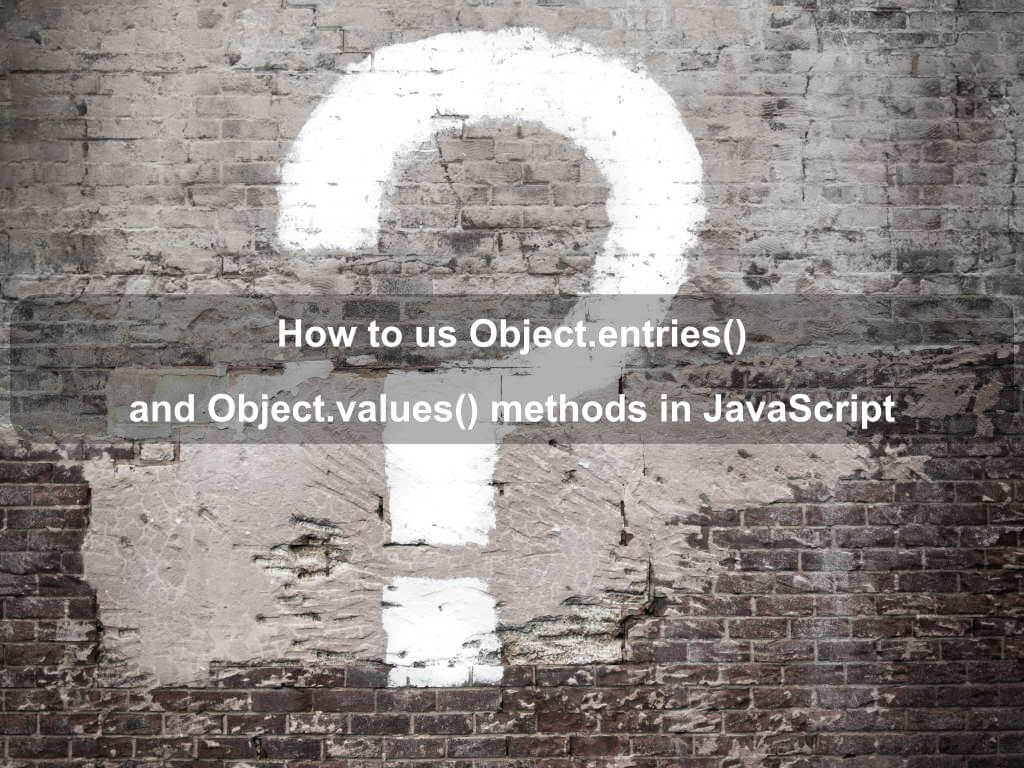
Are we missing something? Help us improve this article. Reach out to us.
Object.entries() and Object.values() methods in JavaScript
The Object.entries()
and Object.values()
methods were added to the JavaScript object constructor with the release of ECMAScript 2017 (ES8).
Let us have a quick look at these useful methods.
Object.entries()
Method
The Object.entries()
method takes an object as argument and returns an array with arrays of key-value pairs:
const birds = {
owl: '🦉',
eagle: '🦅',
duck: '🦆'
}
const entries = Object.entries(birds)
console.log(entries)
// [['owl', '🦉'], ['eagle', '🦅'], ['duck', '🦆']]
The order of the array element does not depend on how the object was defined. The order is the same as that provided by a for...in
loop.
Iterate over an object
The Object.entries()
method can be used to iterate over an object:
// using 'for...of' loop
for (const [key, value] of Object.entries(birds)) {
console.log('${key}: ${value}')
}
// owl: 🦉
// eagle: 🦅
// duck: 🦆
// using array destructuring
Object.entries(birds).forEach(([key, value]) => console.log('${key}: ${value}'))
// owl: 🦉
// eagle: 🦅
// duck: 🦆
Convert an object to a Map
Since the Map
constructor takes an iterable to initialize a map object, the Object.entries()
method can be used to create a map from an object:
const map = new Map(Object.entries(birds))
console.log(map.size) // 3
console.log(map.has('owl')) // true
console.log(map.get('duck')) // 🦆
Object.values()
Method
The Object.values()
method, unlike Object.entries()
, only returns the values of the own enumerable string-keyed properties in the same order as provided by the for...in
loop:
const foods = {
cake: '🍰',
pizza: '🍕',
candy: '🍬',
icecream: '🍨'
}
const values = Object.values(foods)
console.log(values)
// ['🍰', '🍕', '🍬', '🍨']
Both Object.values()
and Object.entries()
do not follow the prototype chain and only iterate through the properties directly added to the given object.
They also ignore all non-enumerable properties:
Object.defineProperty(foods, 'sushi', {
value: '🍣',
writable: true,
configurable: true,
enumerable: false
})
console.log(Object.values(foods))
// ['🍰', '🍕', '🍬', '🍨']
Convert an object to a Set
Since the Set
constructor accepts an iterable, with Object.values()
, we can easily convert an object to a Set
:
const set = new Set(Object.values(foods))
console.log(set.size) // 4
console.log(set.has('🍨')) // true
Are you looking for other code tips?
JS Nooby
Javascript connoisseur