published: 01 Apr 2022
2 min read
Making an HTML number input display 2 decimal places using JavaScript
If you are using an HTML number input field (<input type='number'>
) to collect a dollar amount, here is a little trick to make it always display 2 decimal places using JavaScript.
Let us say you have the following HTML number <input>
tag:
<input type='number' id='price' min='0' max='10' step='0.01' value='0.00'>
Now we can attach a change
event handler to listen for input changes:
const input = document.querySelector('#price')
input.addEventListener('change', e => {
// TODO: Format Number Here
})
Inside the event handler method, we can use the toFixed()
method to round the input string to a specified number of decimals:
input.addEventListener('change', e => {
e.currentTarget.value = parseFloat(e.currentTarget.value).toFixed(2)
})
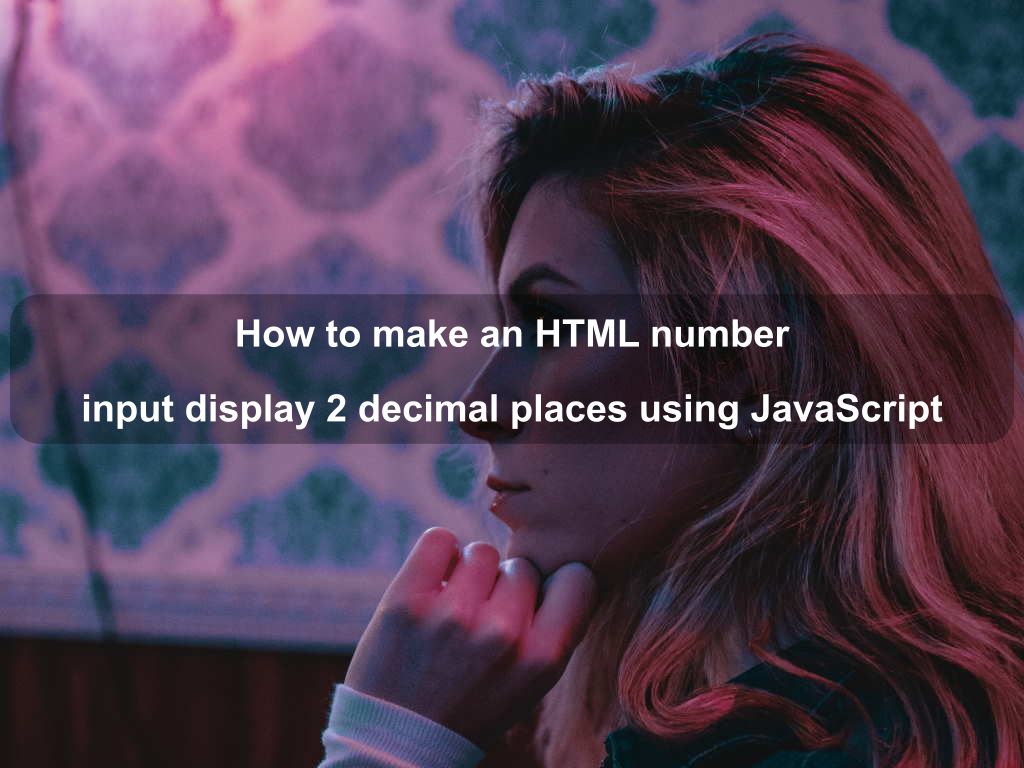
Are we missing something? Help us improve this article. Reach out to us.
Making an HTML number input display 2 decimal places using JavaScript
If you are using an HTML number input field (<input type='number'>
) to collect a dollar amount, here is a little trick to make it always display 2 decimal places using JavaScript.
Let us say you have the following HTML number <input>
tag:
<input type='number' id='price' min='0' max='10' step='0.01' value='0.00'>
Now we can attach a change
event handler to listen for input changes:
const input = document.querySelector('#price')
input.addEventListener('change', e => {
// TODO: Format Number Here
})
Inside the event handler method, we can use the toFixed()
method to round the input string to a specified number of decimals:
input.addEventListener('change', e => {
e.currentTarget.value = parseFloat(e.currentTarget.value).toFixed(2)
})
Are you looking for other code tips?
JS Nooby
Javascript connoisseur