published: 02 Sep 2022
2 min read
JavaScript String replace() and replaceAll() Methods
The replace()
method in JavaScript searches a string for a specified value or a regular expression and returns a new string with some or all matched occurrences replaced.
The replace()
method accepts two parameters:
const newStr = string.replace(substr|regexp, newSubstr|function);
The first parameter can be a string or a regular expression. If it is a string value, only the first instance of the value will be replaced.
To replace all occurrences of a specified value, you must use a regular expression with the global modifier (g
).
The second parameter can be a new string value or a function. If it is a function, it will be invoked after the match has been performed. The function's return value will be used as the replacement string.
The replace()
method does not change the original string object. It only returns a new string.
Examples
The following example demonstrates how you can specify a replacement string as a parameter:
const str = 'JavaScript Courses';
const newStr = str.replace('JavaScript', 'Java');
console.log(newStr); // Java Courses
To perform a global search to replace all occurrences of a string, use a regular expression with global modifier:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/g, 'blue');
console.log(newStr);
// Mr. Red owns a blue bike and a blue car.
For a global case-insensitive replacement, combine global modifier with the ignore case modifier:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, 'blue');
console.log(newStr);
// Mr. blue owns a blue bike and a blue car.
Finally, you could also use a function as second parameter to return the replacement text:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, (match) => {
return match.toUpperCase();
});
console.log(newStr);
// Mr. RED owns a RED bike and a RED car.
replaceAll()
Method
newest
The replaceAll()
method is the new addition to JavaScript (still at stage 4) that is scheduled to be included in ES2021.
This method replaces all appearances of the search string with the replacement text and returns a new string.
Just like the replace()
method, you can either pass a value or a regular expression as a search parameter:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replaceAll(/red/gi, 'blue');
console.log(newStr);
// Mr. blue owns a blue bike and a blue car.
Note that A RegExp
without the global (g
) modifier will throw a TypeError
: 'replaceAll must be called with a global RegExp'.
You can also pass a function (instead of a string) as the second parameter to the replaceAll()
method:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, (match) => {
return match.toLowerCase();
});
console.log(newStr);
// Mr. red owns a red bike and a red car.
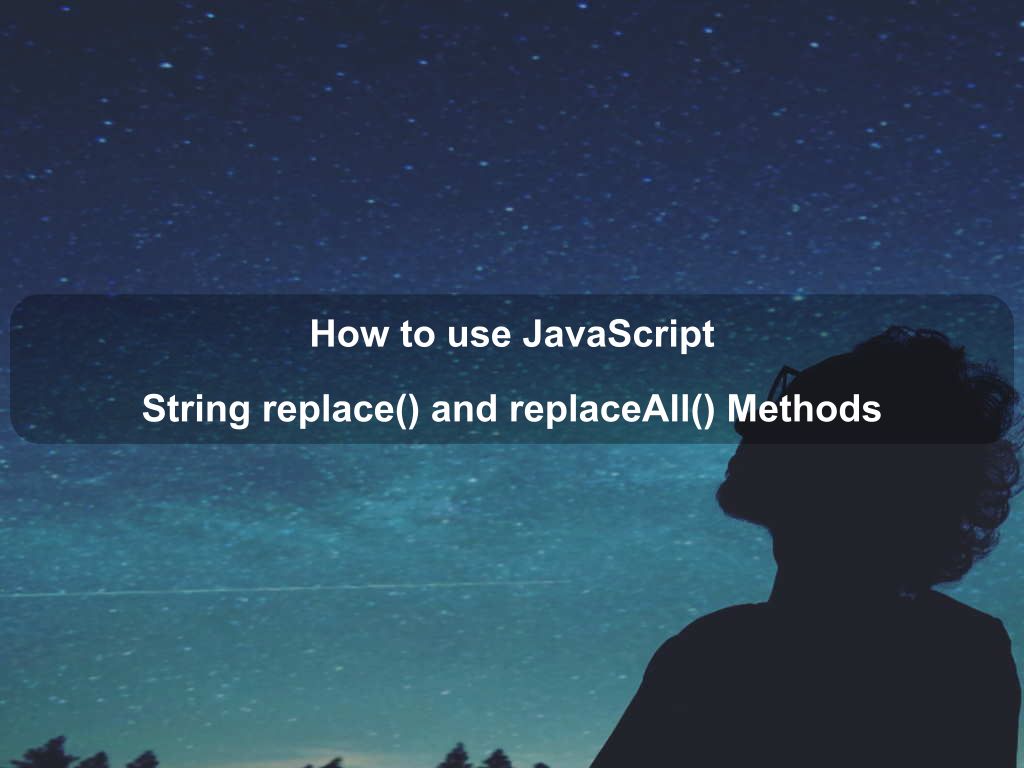
Are we missing something? Help us improve this article. Reach out to us.
JavaScript String replace() and replaceAll() Methods
The replace()
method in JavaScript searches a string for a specified value or a regular expression and returns a new string with some or all matched occurrences replaced.
The replace()
method accepts two parameters:
const newStr = string.replace(substr|regexp, newSubstr|function);
The first parameter can be a string or a regular expression. If it is a string value, only the first instance of the value will be replaced.
To replace all occurrences of a specified value, you must use a regular expression with the global modifier (g
).
The second parameter can be a new string value or a function. If it is a function, it will be invoked after the match has been performed. The function's return value will be used as the replacement string.
The replace()
method does not change the original string object. It only returns a new string.
Examples
The following example demonstrates how you can specify a replacement string as a parameter:
const str = 'JavaScript Courses';
const newStr = str.replace('JavaScript', 'Java');
console.log(newStr); // Java Courses
To perform a global search to replace all occurrences of a string, use a regular expression with global modifier:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/g, 'blue');
console.log(newStr);
// Mr. Red owns a blue bike and a blue car.
For a global case-insensitive replacement, combine global modifier with the ignore case modifier:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, 'blue');
console.log(newStr);
// Mr. blue owns a blue bike and a blue car.
Finally, you could also use a function as second parameter to return the replacement text:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, (match) => {
return match.toUpperCase();
});
console.log(newStr);
// Mr. RED owns a RED bike and a RED car.
replaceAll()
Method
newest
The replaceAll()
method is the new addition to JavaScript (still at stage 4) that is scheduled to be included in ES2021.
This method replaces all appearances of the search string with the replacement text and returns a new string.
Just like the replace()
method, you can either pass a value or a regular expression as a search parameter:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replaceAll(/red/gi, 'blue');
console.log(newStr);
// Mr. blue owns a blue bike and a blue car.
Note that A RegExp
without the global (g
) modifier will throw a TypeError
: 'replaceAll must be called with a global RegExp'.
You can also pass a function (instead of a string) as the second parameter to the replaceAll()
method:
const str = 'Mr. Red owns a red bike and a red car.';
const newStr = str.replace(/red/gi, (match) => {
return match.toLowerCase();
});
console.log(newStr);
// Mr. red owns a red bike and a red car.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur