published: 09 Jul 2022
2 min read
Iterating over all keys stored in local storage using JavaScript
There are many ways to iterate through all keys stored in a localStorage
object using JavaScript.
The quickest way is to use the for loop to iterate over all keys just like an array:
for (let i = 0; i < localStorage.length; i++) {
const key = localStorage.key(i)
console.log('${key}: ${localStorage.getItem(key)}')
}
Another way is to use the for...in loop to iterate over all keys of the localStorage
object:
for (const key in localStorage) {
console.log('${key}: ${localStorage.getItem(key)}')
}
The above code snippet iterates over all keys stored in localStorage
and prints them on the console. However, it also outputs the built-in localStorage
properties like length
, getItem
, setItem
, and so on.
To filter-out the object prototype's own properties, you can use the hasOwnProperty()
method as shown below:
for (const key in localStorage) {
// Skip built-in properties like length, setItem, etc.
if (localStorage.hasOwnProperty(key)) {
console.log('${key}: ${localStorage.getItem(key)}')
}
}
Finally, the last way is to use the Object.keys()
method to collect all 'own' keys of localStorage
and then loop over them by using the for...of loop:
const keys = Object.keys(localStorage)
for (let key of keys) {
console.log('${key}: ${localStorage.getItem(key)}')
}
Read this article to learn more about HTML web storage API and how to use localStorage
and sessionStorage
objects to store information in the user's browser.
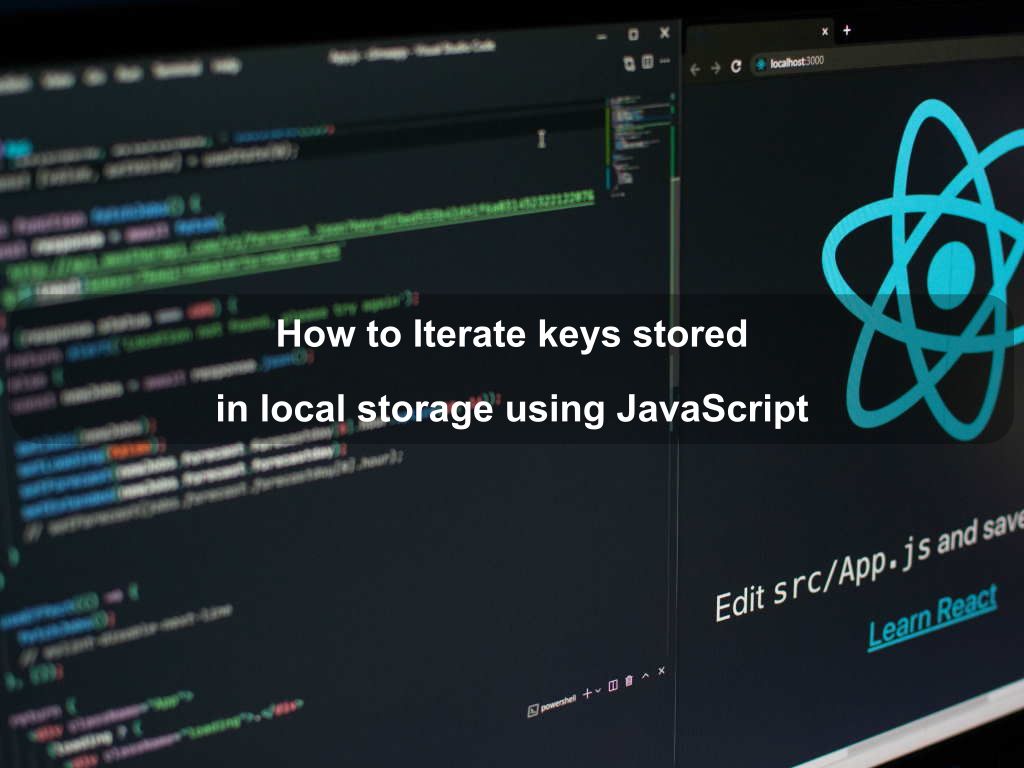
Are we missing something? Help us improve this article. Reach out to us.
Iterating over all keys stored in local storage using JavaScript
There are many ways to iterate through all keys stored in a localStorage
object using JavaScript.
The quickest way is to use the for loop to iterate over all keys just like an array:
for (let i = 0; i < localStorage.length; i++) {
const key = localStorage.key(i)
console.log('${key}: ${localStorage.getItem(key)}')
}
Another way is to use the for...in loop to iterate over all keys of the localStorage
object:
for (const key in localStorage) {
console.log('${key}: ${localStorage.getItem(key)}')
}
The above code snippet iterates over all keys stored in localStorage
and prints them on the console. However, it also outputs the built-in localStorage
properties like length
, getItem
, setItem
, and so on.
To filter-out the object prototype's own properties, you can use the hasOwnProperty()
method as shown below:
for (const key in localStorage) {
// Skip built-in properties like length, setItem, etc.
if (localStorage.hasOwnProperty(key)) {
console.log('${key}: ${localStorage.getItem(key)}')
}
}
Finally, the last way is to use the Object.keys()
method to collect all 'own' keys of localStorage
and then loop over them by using the for...of loop:
const keys = Object.keys(localStorage)
for (let key of keys) {
console.log('${key}: ${localStorage.getItem(key)}')
}
Read this article to learn more about HTML web storage API and how to use localStorage
and sessionStorage
objects to store information in the user's browser.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur