published: 28 May 2022
2 min read
Introduction to Map object in JavaScript
A Map
is a new data structure in JavaScript that allows you to create collections of key-value pairs. They were introduced with ES6 (also called ES2015) along with the Set
object in JavaScript. A Map
object can store both objects and primitives as keys and values.
JavaScript maps are similar to objects used for storing key-value data. But the main difference is that map keys can be of any type and are not limited to just strings and symbols. Unlike objects, it is also easier to get the size of the map.
Creating a Map
object
Just like sets, you can use the Map()
constructor to create an empty map:
const items = new Map()
You can also pass an iterable (such as an array) to the constructor to initialize the map:
const items = new Map([['🦅', 'Eagle'], ['🐶', 'Dog']])
Map
methods
The main methods and properties are set()
, get()
, has()
, size
, delete()
, and clear()
. Here is a simple example of a map showing the use of these methods:
const items = new Map()
// add items
items.set('🐶', 'Dog')
items.set('🦅', 'Eagle')
items.set('🚄', 'Train')
items.set(45, 'Number')
items.set(true, 'Boolean')
// get item
items.get(45) // Number
items.get('🐶') // Dog
items.get('🐺') // undefined
// check if the key exists
items.has('🚄') // true
items.has('🐺') // false
// get items count
items.size // 5
// delete item
items.delete('🦅') // true
items.delete('🦅') // false - already removed
// delete all items
items.clear()
Just like Set
, Map
keys are also unique. Calling set()
more than once with the same key won't add multiple key-value pairs. Instead, the value part is replaced with the newest value:
const animals = new Map()
animals.set('🐺', 'Wolf')
animals.set('🐺', 'Wolf Face')
console.log(animals) // Map(1) {'🐺' => 'Wolf Face'}
Adding objects in Map
Since Map
allows us to store any data type as a key or value, we can store complex objects like object literals, arrays, and even functions:
const props = {
browser: 'Chrome',
os: 'Ubuntu 19.04'
}
const hamburger = () => '🍔'
const things = new Map()
things.set('birds', ['🦉', '🦅'])
things.set('user', { name: 'John Doe', planet: 'Earth' })
things.set(props, 59)
things.set(hamburger, 'What is the food?')
things.get(props) // 59
things.get(hamburger) // What is the food?
Iterating over a Map
object
Unlike objects, when we iterate over the map, the key-value pairs are returned in the same order as they were inserted. We can use the for...of
loop to iterate over all key-value pairs:
const foods = new Map([
['🍌', 'Banana'],
['🍕', 'Pizza'],
['🥒', 'Cucumber'],
['🌽', 'Maize']
])
for (const [key, value] of foods) {
console.log('${key}: ${value}')
}
// 🍌: Banana
// 🍕: Pizza
// 🥒: Cucumber
// 🌽: Maize
Alternatively, we can use the forEach()
method to iterate over all elements:
foods.forEach((key, value) => {
console.log('${key}: ${value}')
})
Keys and values
A Map
object provides keys()
and values()
methods to access the keys and values only. These methods return a new iterable object which can also be used to iterate over all keys or values:
for (const key of foods.keys()) {
console.log(key)
}
for (const value of foods.values()) {
console.log(value)
}
The Map
object also has the entries()
method that returns an iterable for entries [key, value]
. This method is used by default in the for...of
loop. Here's an example:
for (const [key, value] of foods.entries()) {
console.log('${key}: ${value}')
}
Similar to Set
, you can call the next()
method on the iterable returned by the entries()
method to traverse the key-value pairs one by one:
const entries = foods.entries()
console.log(entries.next()) // {value: ['🍌', 'Banana'], done: false}
Conclusion
A map is a collection of key-value pairs, which allows us to store both objects and primitives as keys and values. Unlike an object, map keys can be of any type: objects, arrays, functions, or even another map. Similar to sets, the keys are unique; they can only occur once in the entire map. When we iterate over a map object, the key-value pairs are returned in the same order they were inserted in the map.
If you are interested in learning more, check out our guide on the Set
object in JavaScript.
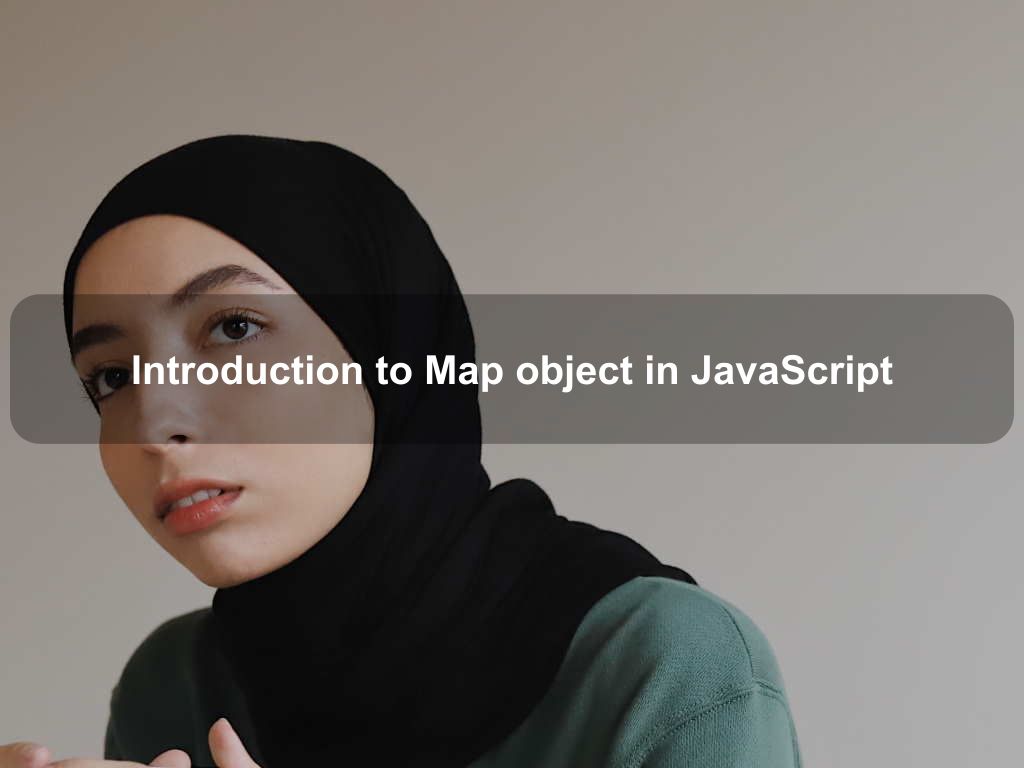
Are we missing something? Help us improve this article. Reach out to us.
Introduction to Map object in JavaScript
A Map
is a new data structure in JavaScript that allows you to create collections of key-value pairs. They were introduced with ES6 (also called ES2015) along with the Set
object in JavaScript. A Map
object can store both objects and primitives as keys and values.
JavaScript maps are similar to objects used for storing key-value data. But the main difference is that map keys can be of any type and are not limited to just strings and symbols. Unlike objects, it is also easier to get the size of the map.
Creating a Map
object
Just like sets, you can use the Map()
constructor to create an empty map:
const items = new Map()
You can also pass an iterable (such as an array) to the constructor to initialize the map:
const items = new Map([['🦅', 'Eagle'], ['🐶', 'Dog']])
Map
methods
The main methods and properties are set()
, get()
, has()
, size
, delete()
, and clear()
. Here is a simple example of a map showing the use of these methods:
const items = new Map()
// add items
items.set('🐶', 'Dog')
items.set('🦅', 'Eagle')
items.set('🚄', 'Train')
items.set(45, 'Number')
items.set(true, 'Boolean')
// get item
items.get(45) // Number
items.get('🐶') // Dog
items.get('🐺') // undefined
// check if the key exists
items.has('🚄') // true
items.has('🐺') // false
// get items count
items.size // 5
// delete item
items.delete('🦅') // true
items.delete('🦅') // false - already removed
// delete all items
items.clear()
Just like Set
, Map
keys are also unique. Calling set()
more than once with the same key won't add multiple key-value pairs. Instead, the value part is replaced with the newest value:
const animals = new Map()
animals.set('🐺', 'Wolf')
animals.set('🐺', 'Wolf Face')
console.log(animals) // Map(1) {'🐺' => 'Wolf Face'}
Adding objects in Map
Since Map
allows us to store any data type as a key or value, we can store complex objects like object literals, arrays, and even functions:
const props = {
browser: 'Chrome',
os: 'Ubuntu 19.04'
}
const hamburger = () => '🍔'
const things = new Map()
things.set('birds', ['🦉', '🦅'])
things.set('user', { name: 'John Doe', planet: 'Earth' })
things.set(props, 59)
things.set(hamburger, 'What is the food?')
things.get(props) // 59
things.get(hamburger) // What is the food?
Iterating over a Map
object
Unlike objects, when we iterate over the map, the key-value pairs are returned in the same order as they were inserted. We can use the for...of
loop to iterate over all key-value pairs:
const foods = new Map([
['🍌', 'Banana'],
['🍕', 'Pizza'],
['🥒', 'Cucumber'],
['🌽', 'Maize']
])
for (const [key, value] of foods) {
console.log('${key}: ${value}')
}
// 🍌: Banana
// 🍕: Pizza
// 🥒: Cucumber
// 🌽: Maize
Alternatively, we can use the forEach()
method to iterate over all elements:
foods.forEach((key, value) => {
console.log('${key}: ${value}')
})
Keys and values
A Map
object provides keys()
and values()
methods to access the keys and values only. These methods return a new iterable object which can also be used to iterate over all keys or values:
for (const key of foods.keys()) {
console.log(key)
}
for (const value of foods.values()) {
console.log(value)
}
The Map
object also has the entries()
method that returns an iterable for entries [key, value]
. This method is used by default in the for...of
loop. Here's an example:
for (const [key, value] of foods.entries()) {
console.log('${key}: ${value}')
}
Similar to Set
, you can call the next()
method on the iterable returned by the entries()
method to traverse the key-value pairs one by one:
const entries = foods.entries()
console.log(entries.next()) // {value: ['🍌', 'Banana'], done: false}
Conclusion
A map is a collection of key-value pairs, which allows us to store both objects and primitives as keys and values. Unlike an object, map keys can be of any type: objects, arrays, functions, or even another map. Similar to sets, the keys are unique; they can only occur once in the entire map. When we iterate over a map object, the key-value pairs are returned in the same order they were inserted in the map.
If you are interested in learning more, check out our guide on the Set
object in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur