published: 20 Sep 2022
2 min read
How to use Array.map() method in JavaScript
The Array.prototype.map()
method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each array's element.
The Array.map()
method accepts a callback function as a parameter you want to invoke for each item in the array. This function must return a value after performing all the modifications.
The callback accepts up to three operational parameters. The first is the current item in the iteration, the second is the index of the current item in the array, and the third is the array itself.
Let us look at the following example:
const prices = [45, 9.99, 33, 50]
const updatedPrices = prices.map(price => '$' + price)
console.log(updatedPrices)
// ['$45', '$9.99', '$33', '$50']
As you can see above, we used the map()
function to iterate over an array of numbers and convert its elements to strings by prepending a currency symbol. The updatedPrices
variable is a newly created array with the results of executing a function for each item in the parent array.
Keep in mind that the Array.map()
is a non-mutating function, which means that it doesn't change the original array and only relies on the results of the provided function to create a new array.
The range of elements processed by the map()
function is decided before the first invocation. If new items are added to the array after the map()
begins, they won't be processed by the callback.
Examples
In addition to iterating over all elements of an array and transforming them, the map()
method can be used to do more things. Let us look at a few more examples below.
Extracting values from an array of objects
The map()
method is extremely useful to extract values from an array of objects. Let us say you have the following JSON array that contains users' names and their ages:
const users = [
{
name: 'John Deo',
age: 35
},
{
name: 'Emma Kel',
age: 24
},
{
name: 'Kristy Adam',
age: 42
}
];
Here is an example that uses map()
to extract all ages and returns them as a new array:
const ages = users.map(user => user.age)
console.log(ages)
// [35, 24, 42]
Transforming an array of objects
The map()
method can be used to iterate over all objects in an array, transform the content of each individual object, and return a new array. Here is an example:
const modifiedUsers = users.map(user => {
// assign a random color to each user
user.color = '#' + ((Math.random() * 0xffffff) << 0).toString(16)
// return modified user
return user
})
console.log(modifiedUsers)
// [
// { name: 'John Deo', age: 35, color: '#f76f4b' },
// { name: 'Emma Kel', age: 24, color: '#e05341' },
// { name: 'Kristy Adam', age: 42, color: '#48242c' }
// ]
Conclusion
The Array.map()
method in JavaScript is used to iterate over all array elements and creates a new array. Here are a few things that you should remember about Array.map()
:
- It calls the provided function for each element in an array and returns a new array.
- It doesn't modify the original array contents and only creates a new array with the values returned by the invoked function.
- New elements added to the array after the
map()
function begins are not processed.
The Array.map()
method works in all modern browsers and Internet Explorer 9 and above. You can use a polyfill to support IE6 and higher.
Read this article to learn more about JavaScript arrays and their methods.
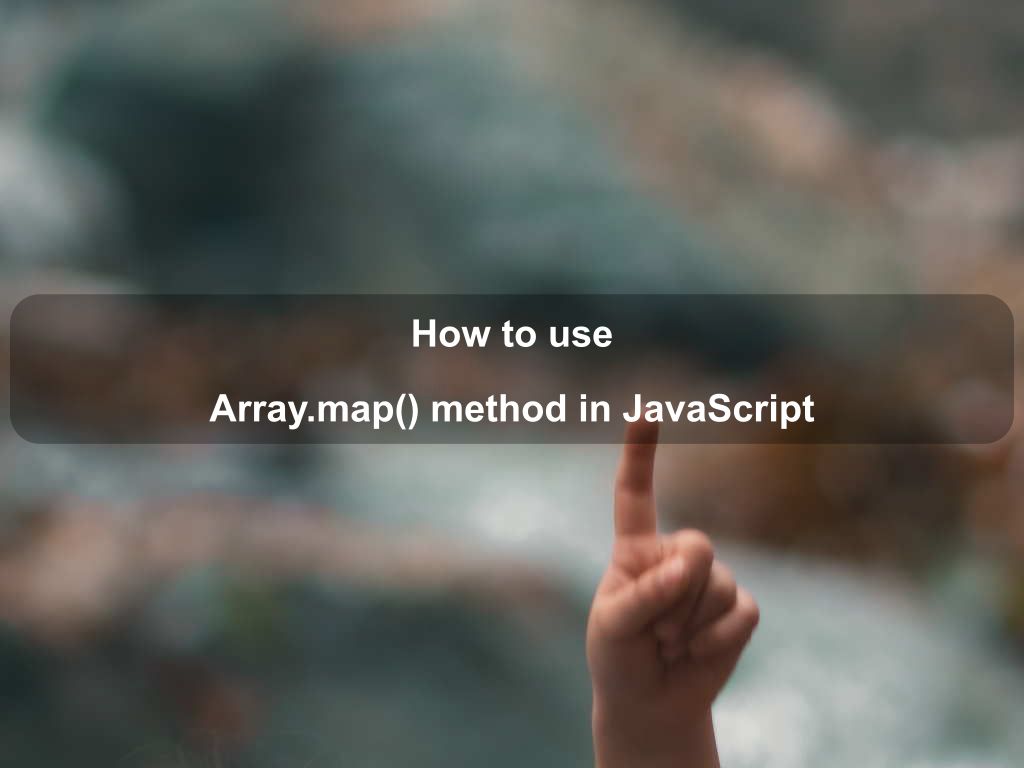
Are we missing something? Help us improve this article. Reach out to us.
How to use Array.map() method in JavaScript
The Array.prototype.map()
method was introduced in ES6 (ECMAScript 2015) for iterating and manipulating elements of an array in one go. This method creates a new array by executing the given function for each array's element.
The Array.map()
method accepts a callback function as a parameter you want to invoke for each item in the array. This function must return a value after performing all the modifications.
The callback accepts up to three operational parameters. The first is the current item in the iteration, the second is the index of the current item in the array, and the third is the array itself.
Let us look at the following example:
const prices = [45, 9.99, 33, 50]
const updatedPrices = prices.map(price => '$' + price)
console.log(updatedPrices)
// ['$45', '$9.99', '$33', '$50']
As you can see above, we used the map()
function to iterate over an array of numbers and convert its elements to strings by prepending a currency symbol. The updatedPrices
variable is a newly created array with the results of executing a function for each item in the parent array.
Keep in mind that the Array.map()
is a non-mutating function, which means that it doesn't change the original array and only relies on the results of the provided function to create a new array.
The range of elements processed by the map()
function is decided before the first invocation. If new items are added to the array after the map()
begins, they won't be processed by the callback.
Examples
In addition to iterating over all elements of an array and transforming them, the map()
method can be used to do more things. Let us look at a few more examples below.
Extracting values from an array of objects
The map()
method is extremely useful to extract values from an array of objects. Let us say you have the following JSON array that contains users' names and their ages:
const users = [
{
name: 'John Deo',
age: 35
},
{
name: 'Emma Kel',
age: 24
},
{
name: 'Kristy Adam',
age: 42
}
];
Here is an example that uses map()
to extract all ages and returns them as a new array:
const ages = users.map(user => user.age)
console.log(ages)
// [35, 24, 42]
Transforming an array of objects
The map()
method can be used to iterate over all objects in an array, transform the content of each individual object, and return a new array. Here is an example:
const modifiedUsers = users.map(user => {
// assign a random color to each user
user.color = '#' + ((Math.random() * 0xffffff) << 0).toString(16)
// return modified user
return user
})
console.log(modifiedUsers)
// [
// { name: 'John Deo', age: 35, color: '#f76f4b' },
// { name: 'Emma Kel', age: 24, color: '#e05341' },
// { name: 'Kristy Adam', age: 42, color: '#48242c' }
// ]
Conclusion
The Array.map()
method in JavaScript is used to iterate over all array elements and creates a new array. Here are a few things that you should remember about Array.map()
:
- It calls the provided function for each element in an array and returns a new array.
- It doesn't modify the original array contents and only creates a new array with the values returned by the invoked function.
- New elements added to the array after the
map()
function begins are not processed.
The Array.map()
method works in all modern browsers and Internet Explorer 9 and above. You can use a polyfill to support IE6 and higher.
Read this article to learn more about JavaScript arrays and their methods.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur