published: 19 Aug 2022
2 min read
How to update the text content of a DOM element in JavaScript
In last article, we looked at how to update the HTML markup of a DOM element in vanilla JavaScript. In this brief tutorial, you'll learn how to get and set the text of an element.
JavaScript provides two properties, innerText
and textContent
, to get and set the text contents of an HTML element and all its child nodes.
If you set a new value of innerText
or textContent
, all child nodes will be removed and replaced with a single text node containing the specified string.
Let us say we have the following <p>
element:
<p id='intro'>My name is <b>John Doe</b>!</p>
The following example shows how to get the text content of the above element:
// grab element
const elem = document.querySelector('#intro')
// get text content
console.log(elem.innerText)
// OR
console.log(elem.textContent)
// My name is John Doe!
To completely replace the existing text contents, just set a new value for innerText
:
// replace existing text
elem.innerText = 'Hey there! I am Atta'
The innerText
property is very similar to the textContent
property. However, there are two differences:
innerText
returns the text contents all elements except<script>
and<style>
elements, whiletextContent
returns text contents of all elements.innerText
can not be used to get text contents of elements that are hidden with CSS, buttextContent
can be used.
Read Next: How to create a DOM element using JavaScript
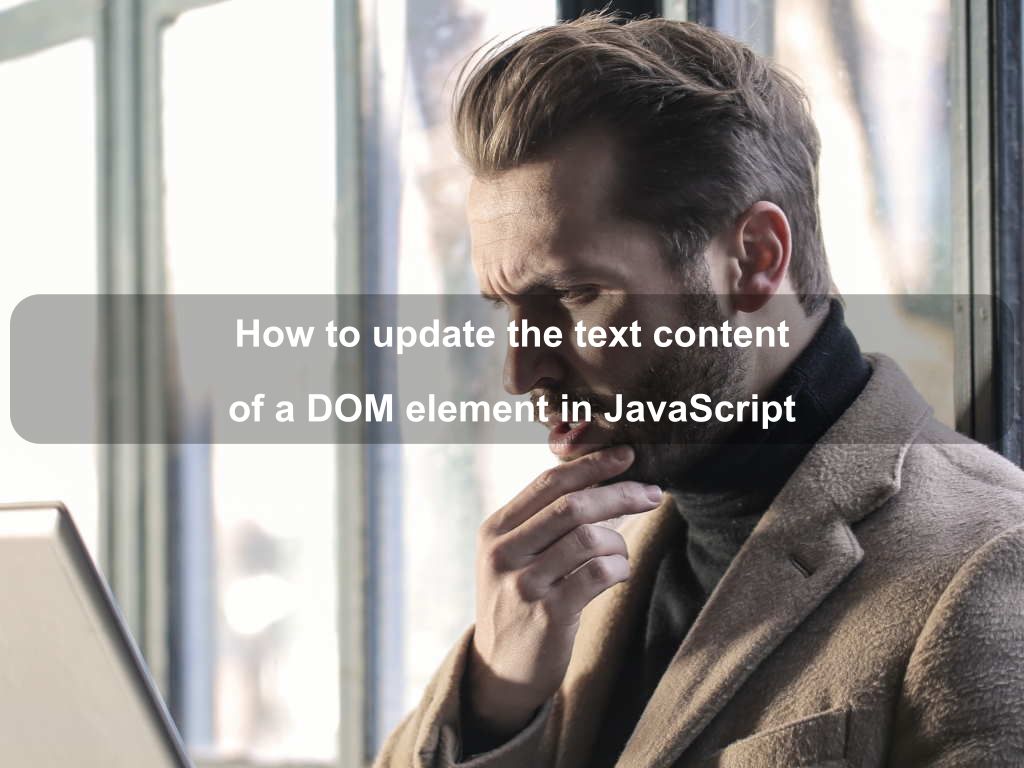
Are we missing something? Help us improve this article. Reach out to us.
How to update the text content of a DOM element in JavaScript
In last article, we looked at how to update the HTML markup of a DOM element in vanilla JavaScript. In this brief tutorial, you'll learn how to get and set the text of an element.
JavaScript provides two properties, innerText
and textContent
, to get and set the text contents of an HTML element and all its child nodes.
If you set a new value of innerText
or textContent
, all child nodes will be removed and replaced with a single text node containing the specified string.
Let us say we have the following <p>
element:
<p id='intro'>My name is <b>John Doe</b>!</p>
The following example shows how to get the text content of the above element:
// grab element
const elem = document.querySelector('#intro')
// get text content
console.log(elem.innerText)
// OR
console.log(elem.textContent)
// My name is John Doe!
To completely replace the existing text contents, just set a new value for innerText
:
// replace existing text
elem.innerText = 'Hey there! I am Atta'
The innerText
property is very similar to the textContent
property. However, there are two differences:
innerText
returns the text contents all elements except<script>
and<style>
elements, whiletextContent
returns text contents of all elements.innerText
can not be used to get text contents of elements that are hidden with CSS, buttextContent
can be used.
Read Next: How to create a DOM element using JavaScript
Are you looking for other code tips?
JS Nooby
Javascript connoisseur