published: 03 Sep 2022
2 min read
How to update all elements in an array using JavaScript
To update all elements in an array using JavaScript:
- Use the
Array.forEach()
method to iterate over the array. It accepts a callback function with the array element, its index, and the array itself. - The callback function is invoked for each element in an array in ascending order.
- Inside the callback function, use the current element index to modify the corresponding array element.
const birds = ['🐦', '🦅', '🦆', '🦉']
birds.forEach((bird, index) => {
birds[index] = '${bird} -> ${index}'
})
console.log(birds)
// [ '🐦 -> 0', '🦅 -> 1', '🦆 -> 2', '🦉 -> 3' ]
The Array.forEach()
method was introduced in ES6, and executes the given callback function once for each element in an array in ascending order. It doesn't invokes the callback function for empty array elements.
The above solution changes the original array in place. If you don't want to mutate the original array, use the Array.map()
method instead:
const original = ['zero', 'one', 'two']
const updated = original.map((item, index) => {
return '${item} -> ${index}'
})
console.log(updated)
// [ 'zero -> 0', 'one -> 1', 'two -> 2' ]
console.log(original)
// [ 'zero', 'one', 'two' ]
The Array.map()
method invokes the given callback function for each element in the array. It does not mutate the original array and relies on the results of the callback function to create a new array.
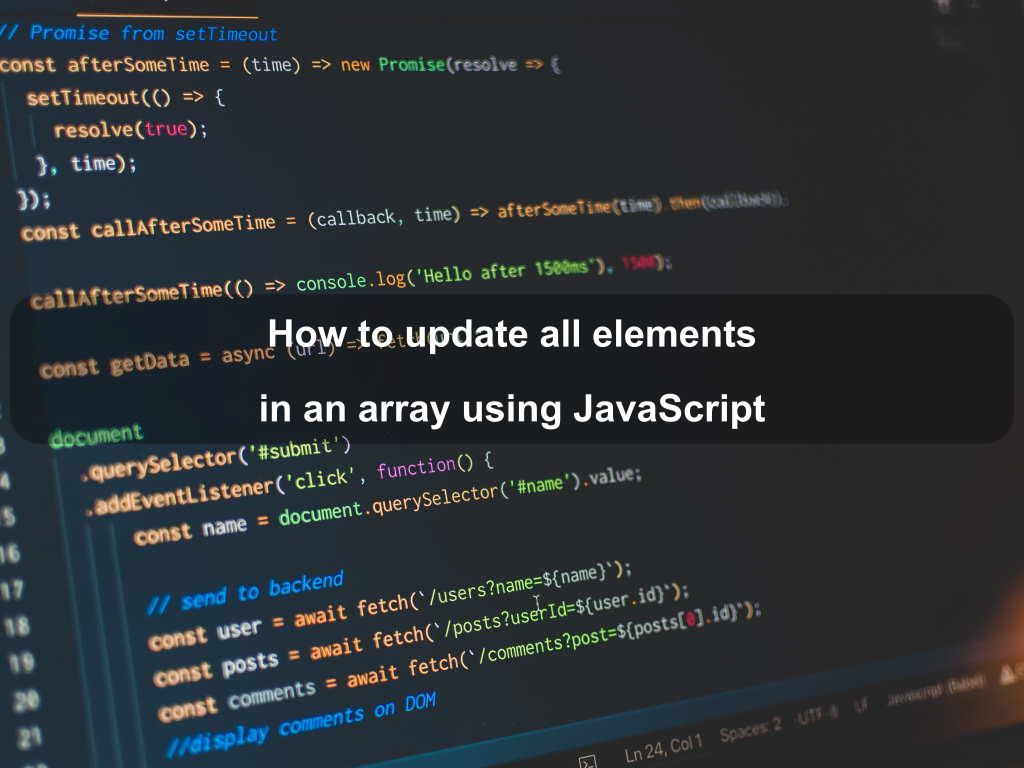
Are we missing something? Help us improve this article. Reach out to us.
How to update all elements in an array using JavaScript
To update all elements in an array using JavaScript:
- Use the
Array.forEach()
method to iterate over the array. It accepts a callback function with the array element, its index, and the array itself. - The callback function is invoked for each element in an array in ascending order.
- Inside the callback function, use the current element index to modify the corresponding array element.
const birds = ['🐦', '🦅', '🦆', '🦉']
birds.forEach((bird, index) => {
birds[index] = '${bird} -> ${index}'
})
console.log(birds)
// [ '🐦 -> 0', '🦅 -> 1', '🦆 -> 2', '🦉 -> 3' ]
The Array.forEach()
method was introduced in ES6, and executes the given callback function once for each element in an array in ascending order. It doesn't invokes the callback function for empty array elements.
The above solution changes the original array in place. If you don't want to mutate the original array, use the Array.map()
method instead:
const original = ['zero', 'one', 'two']
const updated = original.map((item, index) => {
return '${item} -> ${index}'
})
console.log(updated)
// [ 'zero -> 0', 'one -> 1', 'two -> 2' ]
console.log(original)
// [ 'zero', 'one', 'two' ]
The Array.map()
method invokes the given callback function for each element in the array. It does not mutate the original array and relies on the results of the callback function to create a new array.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur