published: 03 Sep 2022
2 min read
How to trim all strings in an array using JavaScript
To trim all strings in an array using JavaScript:
- Use the
Array.map()
method to iterate over all elements in an array. - Use the
trim()
method to remove whitespace from both sides of a string in each iteration. - The
map()
method will return a new array containing strings with no whitespace.
const arr = [' zero ', ' one ', ' two ']
const updated = arr.map(item => item.trim())
console.log(updated)
// [ 'zero', 'one', 'two' ]
The Array.map()
method invokes the given callback function for each element in the array. It does not mutate the original array. Instead, it creates a new array using the results of the callback function.
The trim()
function removes leading and trailing whitespace from a string. It also does not mutate the original string.
If you need to update the array in place, use the Array.forEach()
method instead:
const arr = [' zero ', ' one ', ' two ']
arr.forEach((item, index) => {
arr[index] = item.trim()
})
console.log(arr)
// [ 'zero', 'one', 'two' ]
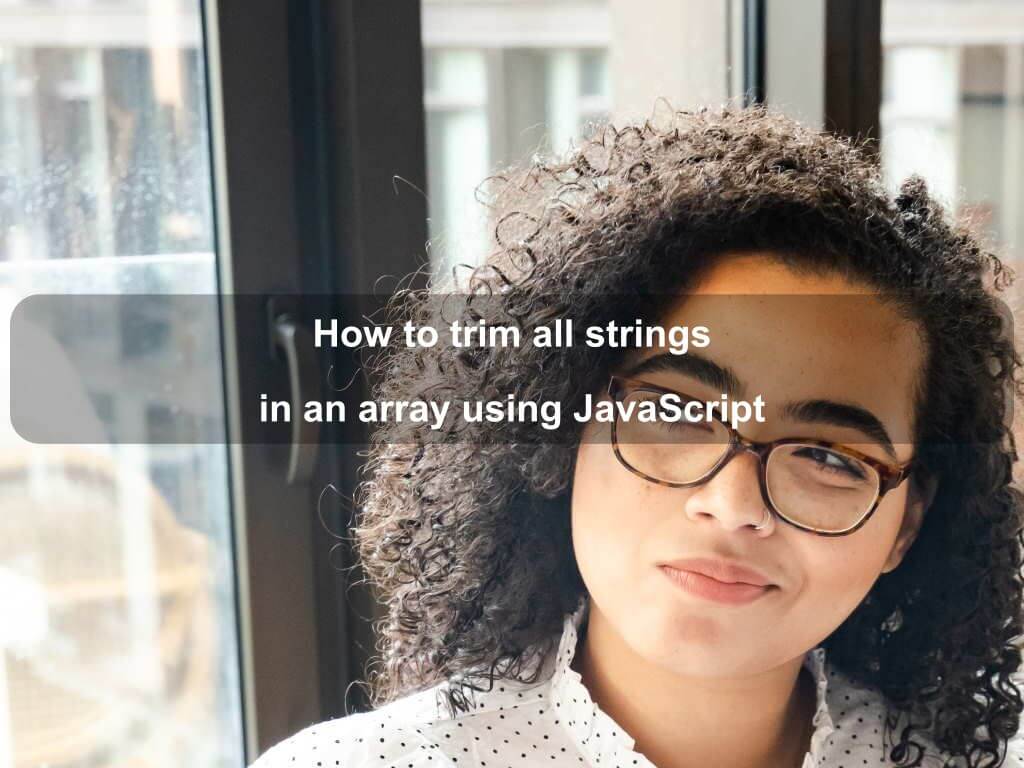
Are we missing something? Help us improve this article. Reach out to us.
How to trim all strings in an array using JavaScript
To trim all strings in an array using JavaScript:
- Use the
Array.map()
method to iterate over all elements in an array. - Use the
trim()
method to remove whitespace from both sides of a string in each iteration. - The
map()
method will return a new array containing strings with no whitespace.
const arr = [' zero ', ' one ', ' two ']
const updated = arr.map(item => item.trim())
console.log(updated)
// [ 'zero', 'one', 'two' ]
The Array.map()
method invokes the given callback function for each element in the array. It does not mutate the original array. Instead, it creates a new array using the results of the callback function.
The trim()
function removes leading and trailing whitespace from a string. It also does not mutate the original string.
If you need to update the array in place, use the Array.forEach()
method instead:
const arr = [' zero ', ' one ', ' two ']
arr.forEach((item, index) => {
arr[index] = item.trim()
})
console.log(arr)
// [ 'zero', 'one', 'two' ]
Are you looking for other code tips?
JS Nooby
Javascript connoisseur