published: 28 Sep 2022
2 min read
How to sort an array of objects by a property value in JavaScript
In the previous article, we looked at how to sort an object's properties by their values in JavaScript. Let us find out how to sort an array of objects by a property value in JavaScript.
Suppose, we have an array of users with their name and age like this:
const users = [
{ name: 'John Deo', age: 23 },
{ name: 'Mike Datson', age: 21 },
{ name: 'Alex Kate', age: 45 }
]
We could sort these users by either their name
or age
. JavaScript arrays provide a sort()
method to logically sort data inside the array.
The sort()
method also accepts a callback function that allows us implement a custom comparator. The callback function accepts two parameters: the elements of array to be compared at any given time:
const sorted = users.sort((a, b) => (a.name > b.name ? 1 : a.name < b.name ? -1 : 0))
console.log(sorted)
During the comparison, we return 1
, 0
, or -1
, indicating the result of the comparison. If 1
is returned, a
is considered greater than b
. 0
indicates equality. -1
signifies that b
is lesser than a
.
You will see the following output:
[
{ name: 'Alex Kate', age: 45 },
{ name: 'John Deo', age: 23 },
{ name: 'Mike Datson', age: 21 }
]
Note: In strings comparison, both uppercase and lowercase letters have different weights. For instance, the lowercase letter
a
will take precedence over the uppercase letterA
. Therefore, it is considered good practice to either lowercase or uppercase strings before comparison.
To take into account both lowercase and uppercase letters, let us update our callback function:
const sorted = users.sort((a, b) => (a.name.toLowerCase() > b.name.toLowerCase() ? 1 :
a.name.toLowerCase() < b.name.toLowerCase() ? -1 : 0))
Similarly, we can also sort users by their age
. Since age
is an integer, the callback function can be simplified like below:
const sorted = users.sort((a, b) => a.age - b.age)
console.log(sorted)
After sorting by age
, the sorted
object will be:
[
{ name: 'Mike Datson', age: 21 },
{ name: 'John Deo', age: 23 },
{ name: 'Alex Kate', age: 45 }
]
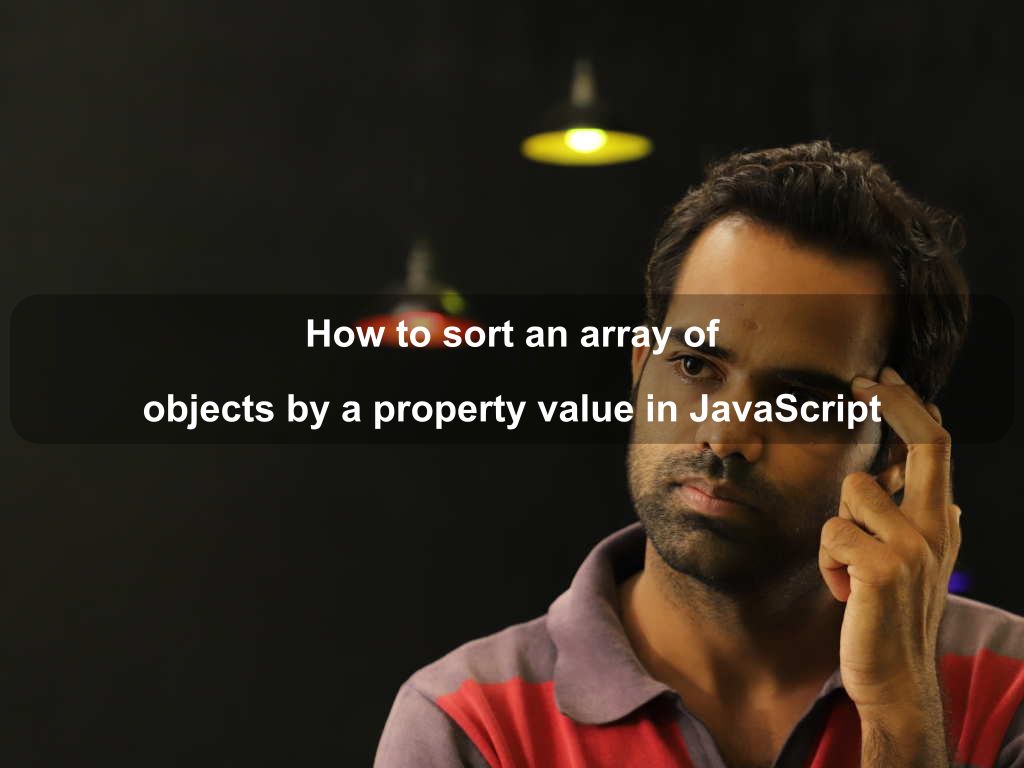
Are we missing something? Help us improve this article. Reach out to us.
How to sort an array of objects by a property value in JavaScript
In the previous article, we looked at how to sort an object's properties by their values in JavaScript. Let us find out how to sort an array of objects by a property value in JavaScript.
Suppose, we have an array of users with their name and age like this:
const users = [
{ name: 'John Deo', age: 23 },
{ name: 'Mike Datson', age: 21 },
{ name: 'Alex Kate', age: 45 }
]
We could sort these users by either their name
or age
. JavaScript arrays provide a sort()
method to logically sort data inside the array.
The sort()
method also accepts a callback function that allows us implement a custom comparator. The callback function accepts two parameters: the elements of array to be compared at any given time:
const sorted = users.sort((a, b) => (a.name > b.name ? 1 : a.name < b.name ? -1 : 0))
console.log(sorted)
During the comparison, we return 1
, 0
, or -1
, indicating the result of the comparison. If 1
is returned, a
is considered greater than b
. 0
indicates equality. -1
signifies that b
is lesser than a
.
You will see the following output:
[
{ name: 'Alex Kate', age: 45 },
{ name: 'John Deo', age: 23 },
{ name: 'Mike Datson', age: 21 }
]
Note: In strings comparison, both uppercase and lowercase letters have different weights. For instance, the lowercase letter
a
will take precedence over the uppercase letterA
. Therefore, it is considered good practice to either lowercase or uppercase strings before comparison.
To take into account both lowercase and uppercase letters, let us update our callback function:
const sorted = users.sort((a, b) => (a.name.toLowerCase() > b.name.toLowerCase() ? 1 :
a.name.toLowerCase() < b.name.toLowerCase() ? -1 : 0))
Similarly, we can also sort users by their age
. Since age
is an integer, the callback function can be simplified like below:
const sorted = users.sort((a, b) => a.age - b.age)
console.log(sorted)
After sorting by age
, the sorted
object will be:
[
{ name: 'Mike Datson', age: 21 },
{ name: 'John Deo', age: 23 },
{ name: 'Alex Kate', age: 45 }
]
Are you looking for other code tips?
JS Nooby
Javascript connoisseur