published: 16 Jul 2022
2 min read
How to select elements by tag name using JavaScript
In JavaScript, the getElementsByTagName()
method can be used to select HTML elements from the DOM by their tag names. This method returns an array-like HTMLCollection object that contains a collection of HTML elements.
The following example demonstrates how to use the getElementsByTagName()
method to extract a list of HTML elements from the DOM, and then iterate through them by using the for...of statement:
const elems = document.getElementsByTagName('div');
// iterate using for...of loop
for (const div of elems) {
console.log(div.innerText);
}
The HTMLCollection
object is different than the NodeList and an array. Therefore, you can not use the ES6's forEach() loop over HTMLcollection
elements.
The getElementsByTagName()
method only works in all modern browsers, and is not supported by old browsers like Internet Explorer. Also, it is only limited to selecting elements by their tag name.
For more browsers support and to select DOM elements by any arbitrary CSS selector, just use the querySelectorAll() method:
const elems = document.querySelectorAll('div');
Check out how to select DOM elements tutorial to learn more about different ways of getting DOM elements in JavaScript.
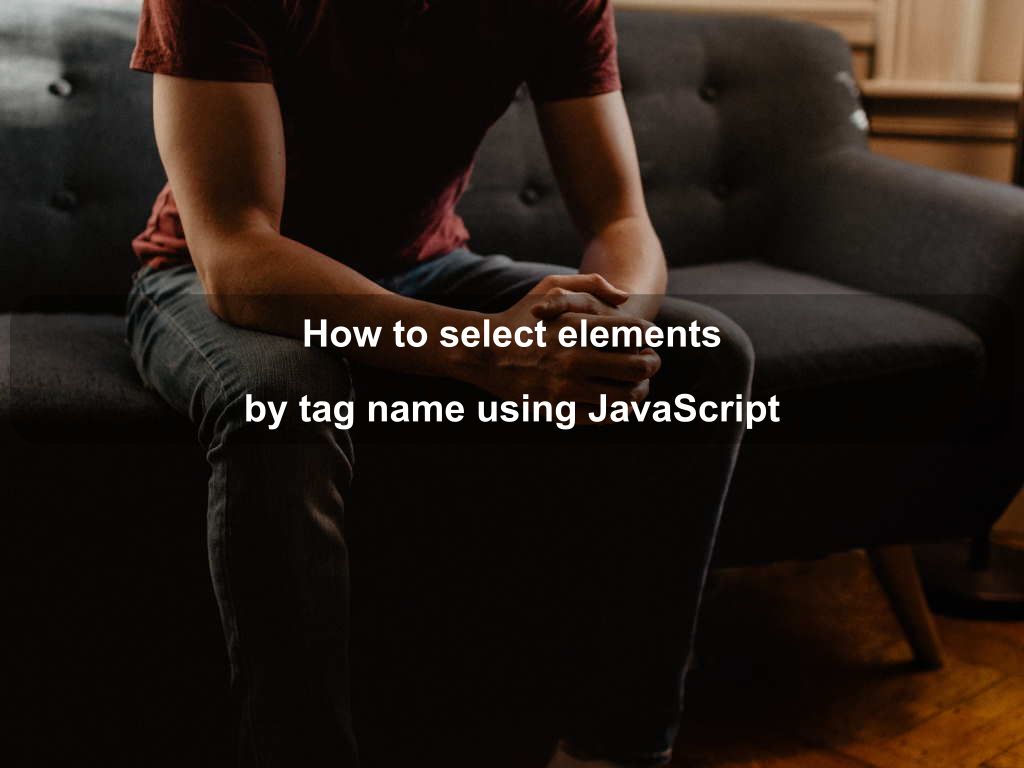
Are we missing something? Help us improve this article. Reach out to us.
How to select elements by tag name using JavaScript
In JavaScript, the getElementsByTagName()
method can be used to select HTML elements from the DOM by their tag names. This method returns an array-like HTMLCollection object that contains a collection of HTML elements.
The following example demonstrates how to use the getElementsByTagName()
method to extract a list of HTML elements from the DOM, and then iterate through them by using the for...of statement:
const elems = document.getElementsByTagName('div');
// iterate using for...of loop
for (const div of elems) {
console.log(div.innerText);
}
The HTMLCollection
object is different than the NodeList and an array. Therefore, you can not use the ES6's forEach() loop over HTMLcollection
elements.
The getElementsByTagName()
method only works in all modern browsers, and is not supported by old browsers like Internet Explorer. Also, it is only limited to selecting elements by their tag name.
For more browsers support and to select DOM elements by any arbitrary CSS selector, just use the querySelectorAll() method:
const elems = document.querySelectorAll('div');
Check out how to select DOM elements tutorial to learn more about different ways of getting DOM elements in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur