published: 31 Jul 2022
2 min read
How to select elements by name using JavaScript
HTML elements may have the optional name
attributes. For example, the following set of radio buttons have the name
attributes with the value animal
:
<input type='radio' name='animal' value='🦄'> Unicorn
<input type='radio' name='animal' value='🦁'> Lion
<input type='radio' name='animal' value='🦊'> Fox
<input type='radio' name='animal' value='🦌'> Deer
To select the above elements by the name
attribute, you can use the getElementsByName()
method.
The getElementsByName()
method returns a collection of all elements in the document that have the specified name as an HTMLCollection object.
The HTMLCollecton
object is an array-like collection of nodes that are accessible by their index numbers.
The following example demonstrates how you can use getElementsByName()
to select all radio buttons and return their values as an array:
const buttons = document.getElementsByName('animal');
const animals = Array.from(buttons).map(btn => btn.value);
console.log(animals);
You should see the following output:
['🦄', '🦁', '🦊', '🦌']
Here is how it works:
- First, select all radio buttons by name using the
getElementsByName()
method. - Then, use Array.from() method to convert the
HTMLCollection
object into an array. It is necessary becauseHTMLCollection
is not an actual JavaScript array. - Finally, the map() method is used to transform the values of radio buttons to an array.
In HTML5, the
name
attribute is replaced with theid
attribute for a lot of elements. You should consider using the getElementById() method when it is appropriate.
Take a look at this guide to learn more about different ways of getting DOM elements in JavaScript.
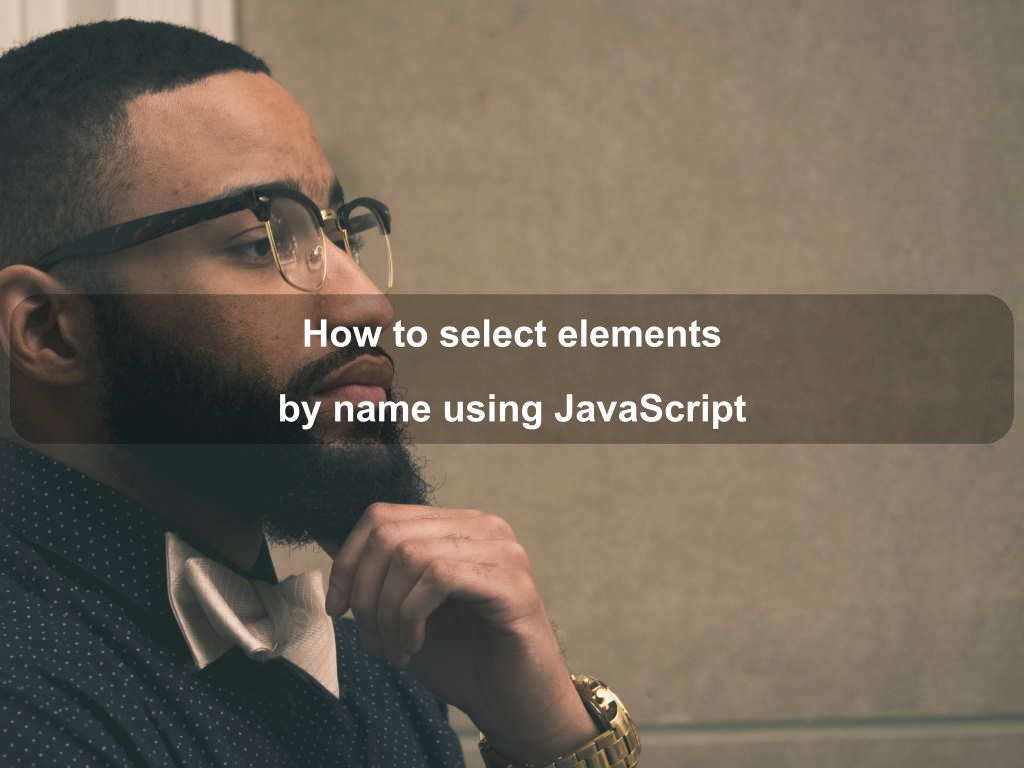
Are we missing something? Help us improve this article. Reach out to us.
How to select elements by name using JavaScript
HTML elements may have the optional name
attributes. For example, the following set of radio buttons have the name
attributes with the value animal
:
<input type='radio' name='animal' value='🦄'> Unicorn
<input type='radio' name='animal' value='🦁'> Lion
<input type='radio' name='animal' value='🦊'> Fox
<input type='radio' name='animal' value='🦌'> Deer
To select the above elements by the name
attribute, you can use the getElementsByName()
method.
The getElementsByName()
method returns a collection of all elements in the document that have the specified name as an HTMLCollection object.
The HTMLCollecton
object is an array-like collection of nodes that are accessible by their index numbers.
The following example demonstrates how you can use getElementsByName()
to select all radio buttons and return their values as an array:
const buttons = document.getElementsByName('animal');
const animals = Array.from(buttons).map(btn => btn.value);
console.log(animals);
You should see the following output:
['🦄', '🦁', '🦊', '🦌']
Here is how it works:
- First, select all radio buttons by name using the
getElementsByName()
method. - Then, use Array.from() method to convert the
HTMLCollection
object into an array. It is necessary becauseHTMLCollection
is not an actual JavaScript array. - Finally, the map() method is used to transform the values of radio buttons to an array.
In HTML5, the
name
attribute is replaced with theid
attribute for a lot of elements. You should consider using the getElementById() method when it is appropriate.
Take a look at this guide to learn more about different ways of getting DOM elements in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur