published: 03 Jul 2022
2 min read
How to replace an element using JavaScript
To replace a DOM element with another element, you can use the replaceChild()
method. This method replaces a child node with a new node.
Let us say you've got the following list:
<ul>
<li>🍔</li>
<li>🍕</li>
<li>🍹</li>
<li>🍲</li>
<li>🍩</li>
</ul>
Now you want to replace the last list item with another item. Just follow the following steps:
- Select the target element that you want to replace.
- Create a new DOM element with all the content you need.
- Select the parent element of the target element and replace the target element with the new one by using the
replaceChild()
method.
Here is an example code snippet:
// select target target
const targetItem = document.querySelector('li:last-child');
// create a new element
const newItem = document.createElement('li');
newItem.innerHTML = '🍰';
// replace 'targetItem' with 'newItem'
targetItem.parentNode.replaceChild(newItem, targetItem);
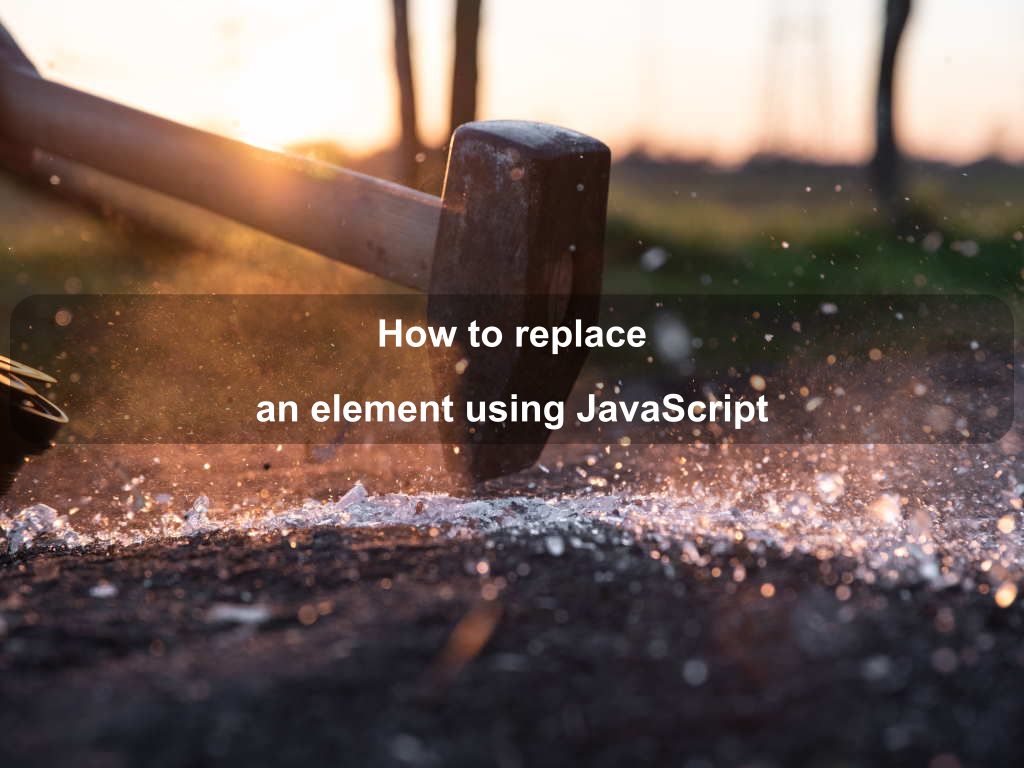
Are we missing something? Help us improve this article. Reach out to us.
How to replace an element using JavaScript
To replace a DOM element with another element, you can use the replaceChild()
method. This method replaces a child node with a new node.
Let us say you've got the following list:
<ul>
<li>🍔</li>
<li>🍕</li>
<li>🍹</li>
<li>🍲</li>
<li>🍩</li>
</ul>
Now you want to replace the last list item with another item. Just follow the following steps:
- Select the target element that you want to replace.
- Create a new DOM element with all the content you need.
- Select the parent element of the target element and replace the target element with the new one by using the
replaceChild()
method.
Here is an example code snippet:
// select target target
const targetItem = document.querySelector('li:last-child');
// create a new element
const newItem = document.createElement('li');
newItem.innerHTML = '🍰';
// replace 'targetItem' with 'newItem'
targetItem.parentNode.replaceChild(newItem, targetItem);
Are you looking for other code tips?
JS Nooby
Javascript connoisseur