published: 23 Aug 2022
2 min read
How to remove whitespace characters from a string in JavaScript
In JavaScript, you can use the trim()
method to remove whitespace characters from the beginning and end of the string. It returns a new string stripped of whitespace characters.
The whitespace characters are space, tab, no-break space, and all the line terminator characters (LF, CR, etc.).
let str = ' Hey there š ';
str = str.trim();
console.log(str); // 'Hey there š'
To remove whitespace characters from the beginning or from the end of a string only, you use the trimStart()
or trimEnd()
method:
str.trimStart(); // 'Hey there š '
str.trimEnd(); // ' Hey there š'
All trim methods return a new string leaving the original string intact.
Line-terminator characters
You can use the trim()
method to remove line terminator characters as well:
'Hey there š \n'.trim(); // 'Hey there š'
'Hey there š \r'.trim(); // 'Hey there š'
'Hey there š \t'.trim(); // 'Hey there š'
Multi-line strings
You can use Template Literals to easily create a mult-line string in JavaScript.
The trim()
method also works for multi-line strings and remove whitespace characters from both ends of the string:
let str = '
Hey
there
š
';
str = str.trim();
console.log(str);
// 'Hey
// there
// š'
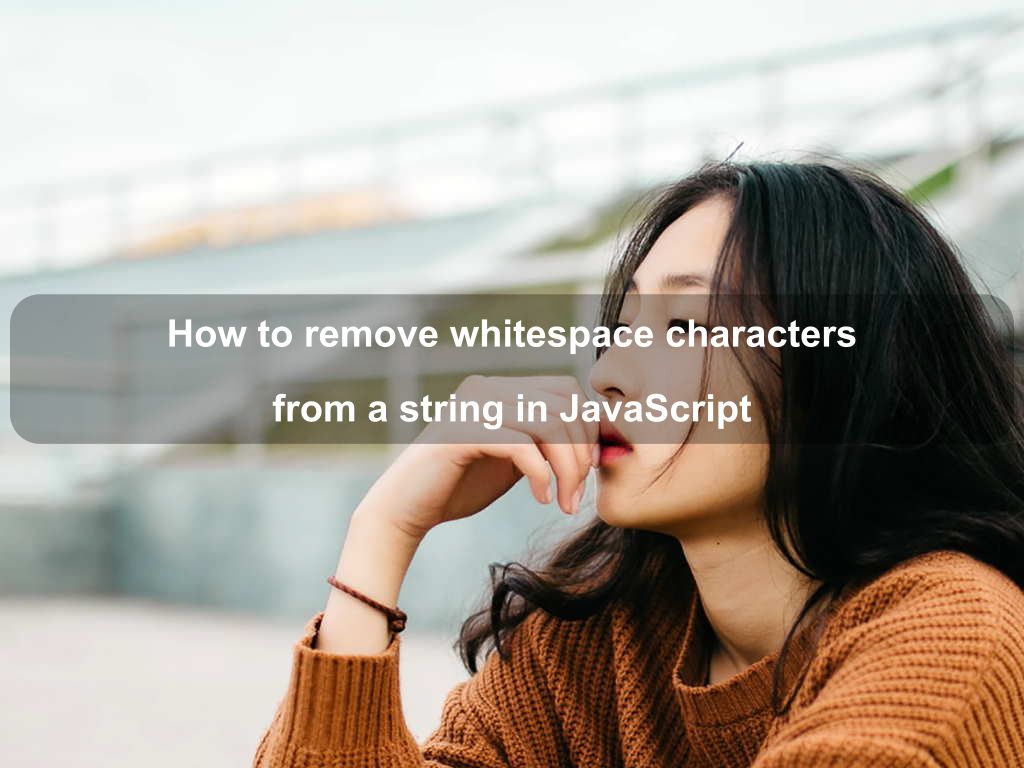
Are we missing something? Help us improve this article. Reach out to us.
How to remove whitespace characters from a string in JavaScript
In JavaScript, you can use the trim()
method to remove whitespace characters from the beginning and end of the string. It returns a new string stripped of whitespace characters.
The whitespace characters are space, tab, no-break space, and all the line terminator characters (LF, CR, etc.).
let str = ' Hey there š ';
str = str.trim();
console.log(str); // 'Hey there š'
To remove whitespace characters from the beginning or from the end of a string only, you use the trimStart()
or trimEnd()
method:
str.trimStart(); // 'Hey there š '
str.trimEnd(); // ' Hey there š'
All trim methods return a new string leaving the original string intact.
Line-terminator characters
You can use the trim()
method to remove line terminator characters as well:
'Hey there š \n'.trim(); // 'Hey there š'
'Hey there š \r'.trim(); // 'Hey there š'
'Hey there š \t'.trim(); // 'Hey there š'
Multi-line strings
You can use Template Literals to easily create a mult-line string in JavaScript.
The trim()
method also works for multi-line strings and remove whitespace characters from both ends of the string:
let str = '
Hey
there
š
';
str = str.trim();
console.log(str);
// 'Hey
// there
// š'
Are you looking for other code tips?
JS Nooby
Javascript connoisseur