published: 01 Aug 2022
2 min read
How to push an object to an array in JavaScript
To push an object to an array in JavaScript:
- Use the
Array.push()
method. - Pass one or more objects as parameters.
- This method adds one or more elements at the end of the array and returns the new length.
const users = []
const obj = {
name: 'John Doe',
age: 23
}
users.push(obj)
console.log(users)
// [ { name: 'John Doe', age: 23 } ]
You can also pass multiple objects as arguments to the Array.push()
method to add multiple objects to an array, as shown below:
const users = []
const obj1 = { name: 'John Doe', age: 23 }
const obj2 = { name: 'Jane Doe', age: 31 }
const obj3 = { name: 'Alex Lee', age: 18 }
users.push(obj1, obj2, obj3)
console.log(users)
// [
// { name: 'John Doe', age: 23 },
// { name: 'Jane Doe', age: 31 },
// { name: 'Alex Lee', age: 18 }
// ]
Read this article to learn more about JavaScript arrays and how to store multiple pieces of information into a single variable.
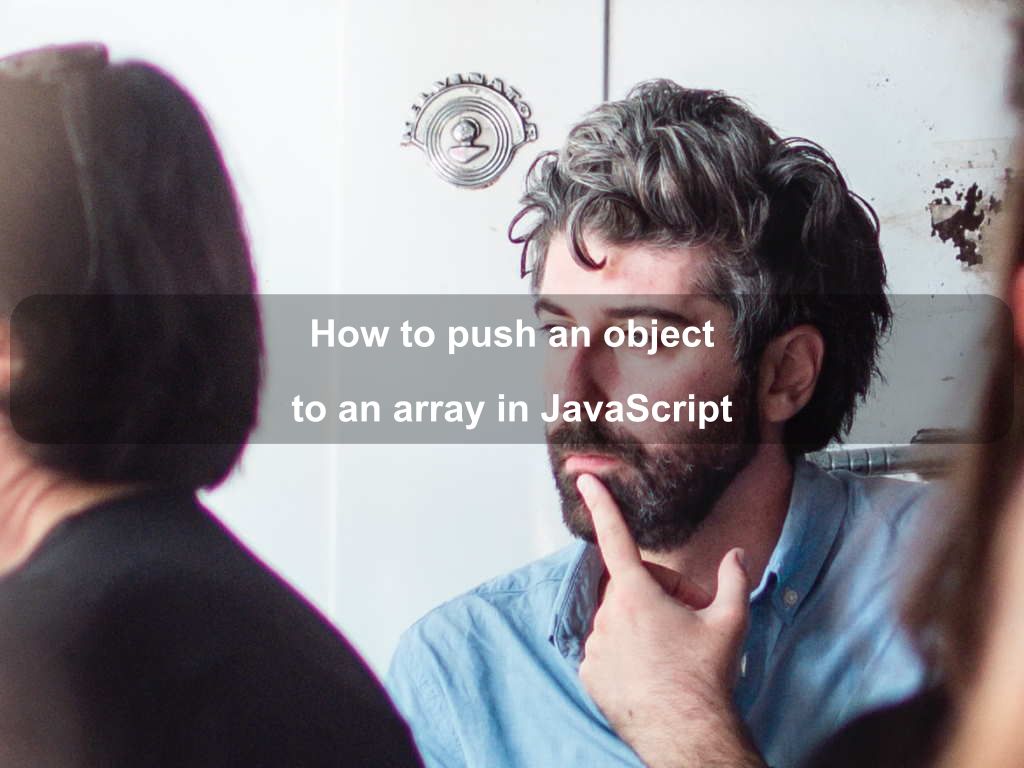
Are we missing something? Help us improve this article. Reach out to us.
How to push an object to an array in JavaScript
To push an object to an array in JavaScript:
- Use the
Array.push()
method. - Pass one or more objects as parameters.
- This method adds one or more elements at the end of the array and returns the new length.
const users = []
const obj = {
name: 'John Doe',
age: 23
}
users.push(obj)
console.log(users)
// [ { name: 'John Doe', age: 23 } ]
You can also pass multiple objects as arguments to the Array.push()
method to add multiple objects to an array, as shown below:
const users = []
const obj1 = { name: 'John Doe', age: 23 }
const obj2 = { name: 'Jane Doe', age: 31 }
const obj3 = { name: 'Alex Lee', age: 18 }
users.push(obj1, obj2, obj3)
console.log(users)
// [
// { name: 'John Doe', age: 23 },
// { name: 'Jane Doe', age: 31 },
// { name: 'Alex Lee', age: 18 }
// ]
Read this article to learn more about JavaScript arrays and how to store multiple pieces of information into a single variable.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur