published: 24 Jul 2022
2 min read
How to pretty-print a JSON object with JavaScript
In an earlier article, we looked at how to use the JSON.stringify()
method to serialize a JSON object into a JSON string. This is extremely useful when sending JON data from a client to a server.
In this article, you'll learn how to use the JSON.stringify()
method to pretty-print a JSON object in JavaScript.
The JSON.stringify()
method accepts up to three parameters: the JSON object, a replacer, and space. Only the JSON object is required. The remaining two parameters are optional.
If you skip the optional parameters when calling JSON.stringify()
, the output JSON string will not include any spaces or line breaks. This makes it hard to read the serialized JSON string especially when you write it in a file.
To improve the readability, what you can do is pass in a number as the 3rd argument representing the total white spaces to insert. The white space count must be between 0 and 10:
const obj = {
name: 'Atta',
profession: 'Software Engineer',
country: 'PK',
skills: ['Java', 'Spring Boot', 'Node.js', 'JavaScript']
};
// serialize JSON object
const str = JSON.stringify(obj, null, 4);
// print JSON string
console.log(str);
The above example will serialize the JSON object to the following string:
{
'name': 'Atta',
'profession': 'Software Engineer',
'country': 'PK',
'skills': [
'Java',
'Spring Boot',
'Node.js',
'JavaScript'
]
}
As you can see above, the serialized JSON string is properly formatted and is way more human-readable than the default behavior. Here is how the output JSON string looks like when you omit the space parameter:
{'name':'Atta','profession':'Software Engineer','country':'PK','skills':['Java','Spring Boot','Node.js','JavaScript']}
That's it. Take a look at this guide to learn more about JSON data parsing and serialization in JavaScript.
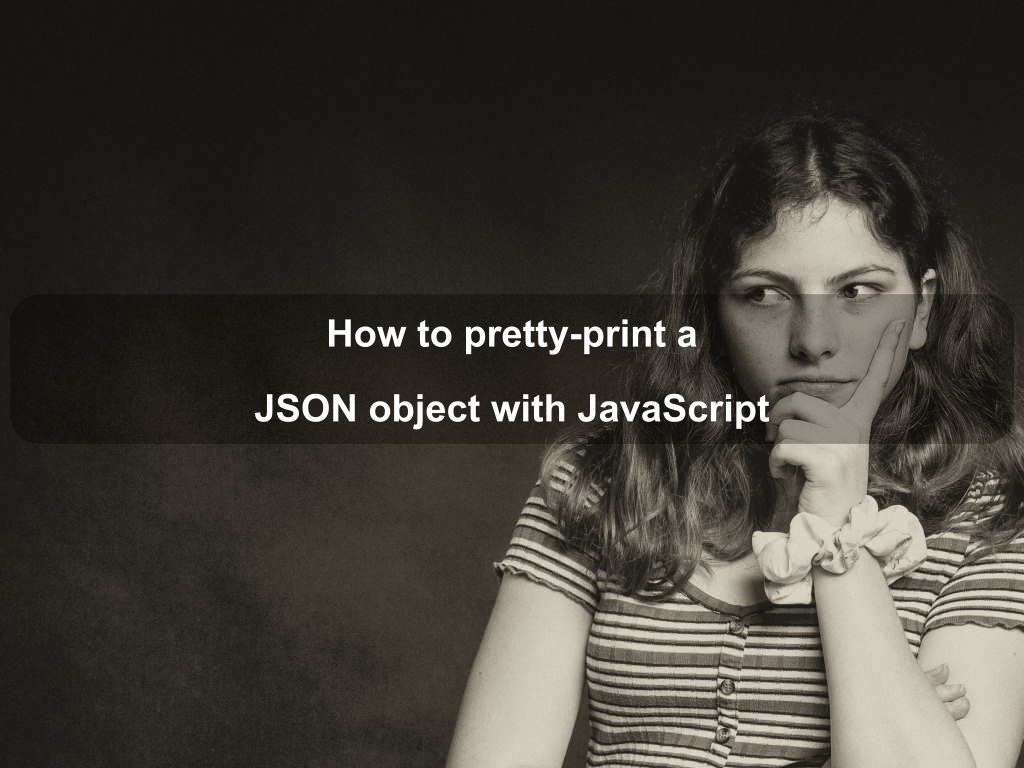
Are we missing something? Help us improve this article. Reach out to us.
How to pretty-print a JSON object with JavaScript
In an earlier article, we looked at how to use the JSON.stringify()
method to serialize a JSON object into a JSON string. This is extremely useful when sending JON data from a client to a server.
In this article, you'll learn how to use the JSON.stringify()
method to pretty-print a JSON object in JavaScript.
The JSON.stringify()
method accepts up to three parameters: the JSON object, a replacer, and space. Only the JSON object is required. The remaining two parameters are optional.
If you skip the optional parameters when calling JSON.stringify()
, the output JSON string will not include any spaces or line breaks. This makes it hard to read the serialized JSON string especially when you write it in a file.
To improve the readability, what you can do is pass in a number as the 3rd argument representing the total white spaces to insert. The white space count must be between 0 and 10:
const obj = {
name: 'Atta',
profession: 'Software Engineer',
country: 'PK',
skills: ['Java', 'Spring Boot', 'Node.js', 'JavaScript']
};
// serialize JSON object
const str = JSON.stringify(obj, null, 4);
// print JSON string
console.log(str);
The above example will serialize the JSON object to the following string:
{
'name': 'Atta',
'profession': 'Software Engineer',
'country': 'PK',
'skills': [
'Java',
'Spring Boot',
'Node.js',
'JavaScript'
]
}
As you can see above, the serialized JSON string is properly formatted and is way more human-readable than the default behavior. Here is how the output JSON string looks like when you omit the space parameter:
{'name':'Atta','profession':'Software Engineer','country':'PK','skills':['Java','Spring Boot','Node.js','JavaScript']}
That's it. Take a look at this guide to learn more about JSON data parsing and serialization in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur