published: 28 Jun 2022
2 min read
How to loop through an array of objects in JavaScript
To iterate through an array of objects in JavaScript, you can use the forEach() method aong with the for...in loop.
Here is an example that demonstrates how you can loop over an array containing objects and print each object's properties in JavaScript:
const mobiles = [
{
brand: 'Samsung',
model: 'Galaxy Note 9'
},
{
brand: 'Google',
model: 'Pixel 3'
},
{
brand: 'Apple',
model: 'iPhone X'
}
]
mobiles.forEach(mobile => {
for (let key in mobile) {
console.log('${key}: ${mobile[key]}')
}
})
You should see the following output for the above code:
brand: Samsung
model: Galaxy Note 9
brand: Google
model: Pixel 3
brand: Apple
model: iPhone X
The outer forEach()
loop is used to iterate through the objects array. We then use the for...in
loop to iterate through the properties of an individual object.
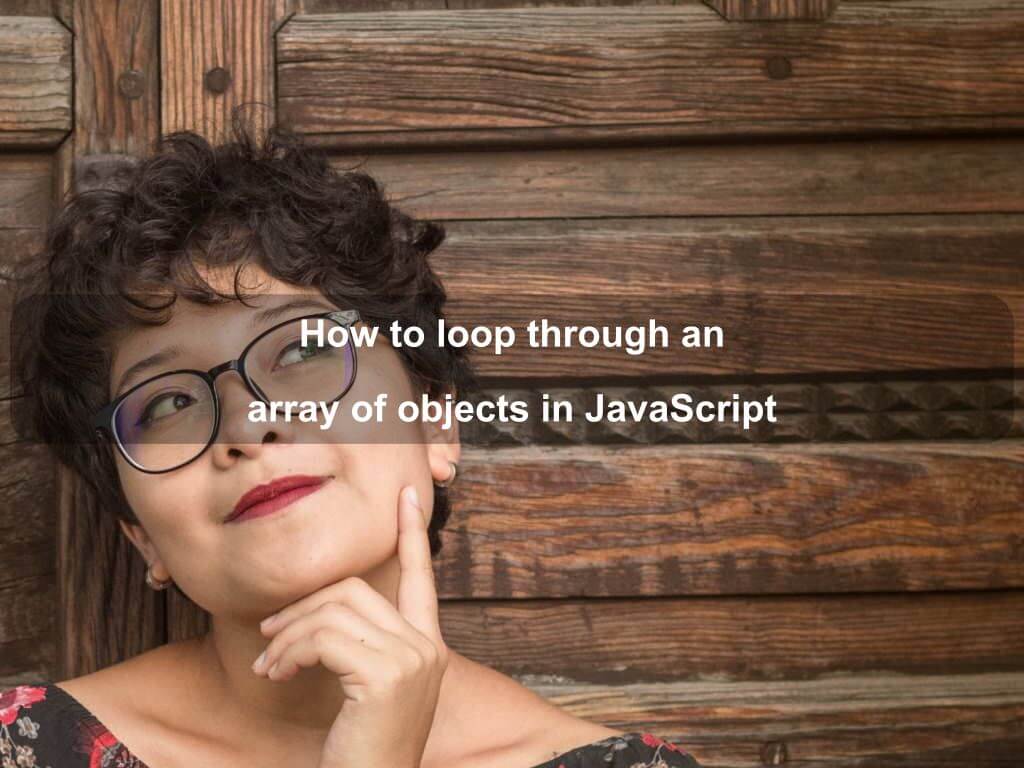
Are we missing something? Help us improve this article. Reach out to us.
How to loop through an array of objects in JavaScript
To iterate through an array of objects in JavaScript, you can use the forEach() method aong with the for...in loop.
Here is an example that demonstrates how you can loop over an array containing objects and print each object's properties in JavaScript:
const mobiles = [
{
brand: 'Samsung',
model: 'Galaxy Note 9'
},
{
brand: 'Google',
model: 'Pixel 3'
},
{
brand: 'Apple',
model: 'iPhone X'
}
]
mobiles.forEach(mobile => {
for (let key in mobile) {
console.log('${key}: ${mobile[key]}')
}
})
You should see the following output for the above code:
brand: Samsung
model: Galaxy Note 9
brand: Google
model: Pixel 3
brand: Apple
model: iPhone X
The outer forEach()
loop is used to iterate through the objects array. We then use the for...in
loop to iterate through the properties of an individual object.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur