published: 20 Apr 2022
2 min read
How to loop through an array in JavaScript
Loops are widely used for running a single piece of code repeatedly, every time with a different value, until a specific condition is met. They are commonly used to iterate through a bunch of values, calculate a sum of numbers, repeatedly call a function, and many other things.
In this article, you will learn how to use JavaScript loops to iterate through the elements of an array.
Modern JavaScript provides different kinds of loops:
for
- Repeats a block of code for a given number of timesforEach()
- Executes the given function for each element in the array or NodeListfor...in
- Loops through the properties of an objectfor...of
- Loops through the values of an iterable objectwhile
- Repeats a block of code while the specified condition is truedo...while
- Loops a block of code until a specific condition is true
for
Loop
The for
loop is used to iterate over arrays and NodeLists in JavaScript. It has the following syntax:
for (init; condition; expr) {
// code block to be executed
}
As you can see above, the for
loop has three statements:
init
is executed once before the code block execution starts. This is where you define whether to loop the entire array or start midway.condition
defines the condition until the loop continues executing the code block. It is a test that gets checked after each iteration of the loop. If it returnstrue
, the loop will keep executing. If it returnsfalse
, the loop ends.expr
gets executed every time after the code block completes the execution. You can use this statement to increment or decrement the counter variable.
Let us take a look at an example:
const birds = ['🐦', '🦅', '🦆', '🦉']
// loop all birds
for (let i = 0; i < birds.length; i++) {
console.log(birds[i]) // current value
console.log(i) // current index
}
In the above example, we use the init
statement to set a variable i
as a counter variable. In the condition
statement, we make sure that the counter variable is always smaller than the total number of array elements. Finally, the expr
statement just increments the counter variable by 1 every time after the code block has done with the execution.
Inside the loop body, we can use the counter variable i
to access the current item from the array.
forEach()
Loop
The Array.forEach() method was introduced in ES6 to execute the specified function once for each element of the array in ascending order.
Here is an example that demonstrates how to use forEach()
to iterate through array elements in JavaScript:
const birds = ['🐦', '🦅', '🦆', '🦉']
birds.forEach((bird, index) => {
console.log('${index} -> ${bird}')
})
// 0 -> 🐦
// 1 -> 🦅
// 2 -> 🦆
// 3 -> 🦉
The index
parameter is optional. You can skip it if not required:
birds.forEach(bird => console.log(bird))
Unfortunately, there is no way to terminate the forEach()
loop.
for...in
Loop
The for...in statement iterates through the properties of an object.
Here is an example:
const person = {
name: 'John Doe',
email: 'john.doe@example.com',
age: 25
}
for (const prop in person) {
console.log(prop) // property name
console.log(person[prop]) // property value
}
The for..in
statement is not just limited to objects. It should also work for an array (not recommended though):
const digits = [2, 3, 5]
for (const index in digits) {
console.log(digits[index])
}
// 2
// 3
// 5
for...of
Loop
The for...of statement was introduced in ES6. It loops through the values of iterable objects like arrays, strings, maps, sets, and much more.
Here is an example:
const birds = ['🐦', '🦅', '🦉']
// iterate over all values
for (const bird of birds) {
console.log('Hey ${bird}')
}
// Hey 🐦
// Hey 🦅
// Hey 🦉
The major difference between the for...in
and for...of
statements is that the former iterates over the property names whereas the latter iterates over the property values.
while
Loop
The while
loop iterates through a code block as long as a specified condition is true. Here is an example:
const cars = ['BMW', 'Porsche', 'Audi', 'Tesla']
let i = 0
while (i < cars.length) {
console.log(i) // index
console.log(cars[i]) // value
i++
}
Do not forget to increment the counter variable i
value. Otherwise, the loop will never end. You can terminate a while
loop using a break
statement:
while (i < cars.length) {
// terminate if index = 2
if (i === 2) {
break
}
// TODO: do whatever you want to here
}
To skip an iteration, just use the continue
statement:
while (i < cars.length) {
// skip 2nd iteration
if (i === 2) {
continue
}
// TODO: do whatever you want to here
}
do...while
Loop
The do...while
loop is similar to the while
loop. The only difference is that the do...while
loop executes the code block at least once before checking the condition. If it is true
, it will repeat the code block as long as the condition remains true
.
Here is an example of the do...while
loop:
const cars = ['BMW', 'Porsche', 'Audi', 'Tesla']
let i = 0
do {
console.log(i) // index
console.log(cars[i]) // value
i++
} while (i < cars.length)
Just like while
, you can use break
and continue
statements to terminate the loop or skip an iteration.
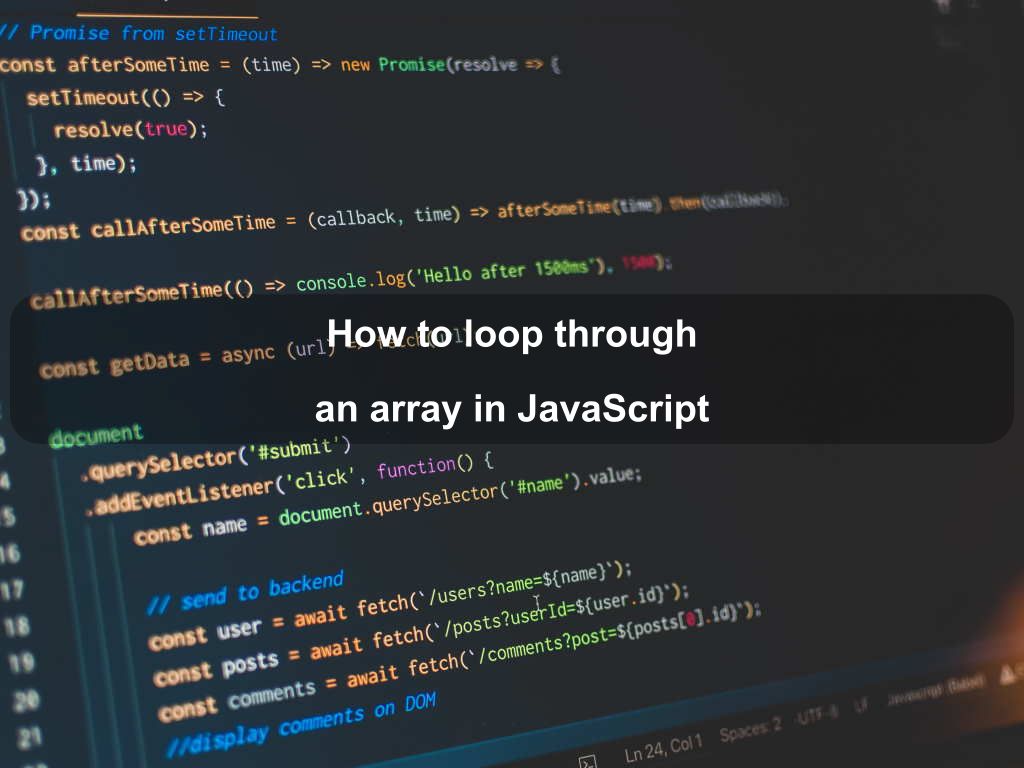
Are we missing something? Help us improve this article. Reach out to us.
How to loop through an array in JavaScript
Loops are widely used for running a single piece of code repeatedly, every time with a different value, until a specific condition is met. They are commonly used to iterate through a bunch of values, calculate a sum of numbers, repeatedly call a function, and many other things.
In this article, you will learn how to use JavaScript loops to iterate through the elements of an array.
Modern JavaScript provides different kinds of loops:
for
- Repeats a block of code for a given number of timesforEach()
- Executes the given function for each element in the array or NodeListfor...in
- Loops through the properties of an objectfor...of
- Loops through the values of an iterable objectwhile
- Repeats a block of code while the specified condition is truedo...while
- Loops a block of code until a specific condition is true
for
Loop
The for
loop is used to iterate over arrays and NodeLists in JavaScript. It has the following syntax:
for (init; condition; expr) {
// code block to be executed
}
As you can see above, the for
loop has three statements:
init
is executed once before the code block execution starts. This is where you define whether to loop the entire array or start midway.condition
defines the condition until the loop continues executing the code block. It is a test that gets checked after each iteration of the loop. If it returnstrue
, the loop will keep executing. If it returnsfalse
, the loop ends.expr
gets executed every time after the code block completes the execution. You can use this statement to increment or decrement the counter variable.
Let us take a look at an example:
const birds = ['🐦', '🦅', '🦆', '🦉']
// loop all birds
for (let i = 0; i < birds.length; i++) {
console.log(birds[i]) // current value
console.log(i) // current index
}
In the above example, we use the init
statement to set a variable i
as a counter variable. In the condition
statement, we make sure that the counter variable is always smaller than the total number of array elements. Finally, the expr
statement just increments the counter variable by 1 every time after the code block has done with the execution.
Inside the loop body, we can use the counter variable i
to access the current item from the array.
forEach()
Loop
The Array.forEach() method was introduced in ES6 to execute the specified function once for each element of the array in ascending order.
Here is an example that demonstrates how to use forEach()
to iterate through array elements in JavaScript:
const birds = ['🐦', '🦅', '🦆', '🦉']
birds.forEach((bird, index) => {
console.log('${index} -> ${bird}')
})
// 0 -> 🐦
// 1 -> 🦅
// 2 -> 🦆
// 3 -> 🦉
The index
parameter is optional. You can skip it if not required:
birds.forEach(bird => console.log(bird))
Unfortunately, there is no way to terminate the forEach()
loop.
for...in
Loop
The for...in statement iterates through the properties of an object.
Here is an example:
const person = {
name: 'John Doe',
email: 'john.doe@example.com',
age: 25
}
for (const prop in person) {
console.log(prop) // property name
console.log(person[prop]) // property value
}
The for..in
statement is not just limited to objects. It should also work for an array (not recommended though):
const digits = [2, 3, 5]
for (const index in digits) {
console.log(digits[index])
}
// 2
// 3
// 5
for...of
Loop
The for...of statement was introduced in ES6. It loops through the values of iterable objects like arrays, strings, maps, sets, and much more.
Here is an example:
const birds = ['🐦', '🦅', '🦉']
// iterate over all values
for (const bird of birds) {
console.log('Hey ${bird}')
}
// Hey 🐦
// Hey 🦅
// Hey 🦉
The major difference between the for...in
and for...of
statements is that the former iterates over the property names whereas the latter iterates over the property values.
while
Loop
The while
loop iterates through a code block as long as a specified condition is true. Here is an example:
const cars = ['BMW', 'Porsche', 'Audi', 'Tesla']
let i = 0
while (i < cars.length) {
console.log(i) // index
console.log(cars[i]) // value
i++
}
Do not forget to increment the counter variable i
value. Otherwise, the loop will never end. You can terminate a while
loop using a break
statement:
while (i < cars.length) {
// terminate if index = 2
if (i === 2) {
break
}
// TODO: do whatever you want to here
}
To skip an iteration, just use the continue
statement:
while (i < cars.length) {
// skip 2nd iteration
if (i === 2) {
continue
}
// TODO: do whatever you want to here
}
do...while
Loop
The do...while
loop is similar to the while
loop. The only difference is that the do...while
loop executes the code block at least once before checking the condition. If it is true
, it will repeat the code block as long as the condition remains true
.
Here is an example of the do...while
loop:
const cars = ['BMW', 'Porsche', 'Audi', 'Tesla']
let i = 0
do {
console.log(i) // index
console.log(cars[i]) // value
i++
} while (i < cars.length)
Just like while
, you can use break
and continue
statements to terminate the loop or skip an iteration.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur