published: 18 Jun 2022
2 min read
How to insert an element after another element in JavaScript
To append an HTML element to another element, you can use the appendChild()
method of the target element object.
The appendChild()
method appends a node as the last child of an existing node.
target.appendChild(elem);
Let us say that you have the following list element:
<ul id='drinks'>
<li>Coffee</li>
<li>Water</li>
</ul>
Now you want to append another element at the end of the list. You can use the following code:
const drinks = document.querySelector('#drinks');
// Create a <li> element
const elem = document.createElement('li');
// Create a new text node
const text = document.createTextNode('Tea');
// Append text node to <li> node
elem.appendChild(text);
// Finally, append <li> to <ul> node
drinks.appendChild(elem);
Take a look at this article to learn more about inserting elements into the DOM in JavaScript.
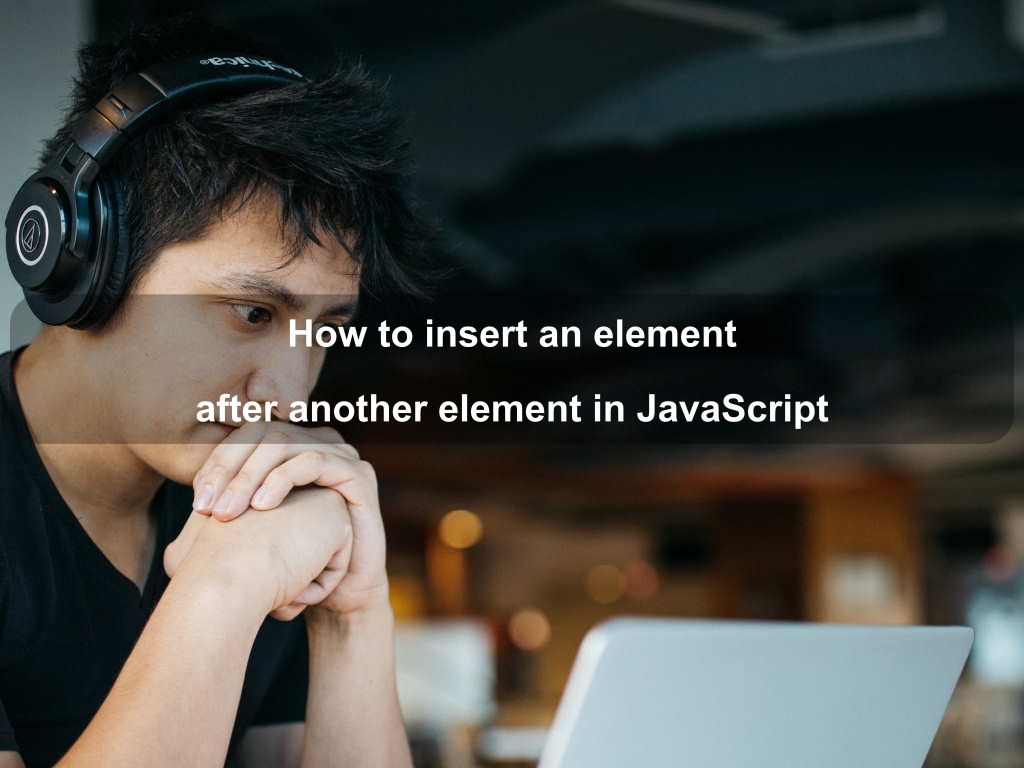
Are we missing something? Help us improve this article. Reach out to us.
How to insert an element after another element in JavaScript
To append an HTML element to another element, you can use the appendChild()
method of the target element object.
The appendChild()
method appends a node as the last child of an existing node.
target.appendChild(elem);
Let us say that you have the following list element:
<ul id='drinks'>
<li>Coffee</li>
<li>Water</li>
</ul>
Now you want to append another element at the end of the list. You can use the following code:
const drinks = document.querySelector('#drinks');
// Create a <li> element
const elem = document.createElement('li');
// Create a new text node
const text = document.createTextNode('Tea');
// Append text node to <li> node
elem.appendChild(text);
// Finally, append <li> to <ul> node
drinks.appendChild(elem);
Take a look at this article to learn more about inserting elements into the DOM in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur