published: 01 Jul 2022
2 min read
How to hide and show DOM elements using JavaScript
There are multiple ways to show or hide DOM elements in vanilla JavaScript. In this article, we'll look at two different ways to hide or show DOM elements using JavaScript.
Using Style display
Property
The style display
property is used to set as well as get the element's display type in JavaScript.
Majority of the HTML elements have the inline or block display type. The content of an inline element floats to its left and right sides. Block HTML elements are different because they * fill* the entire line, and do not show content on their sides.
To hide an element, set the display
property to none
:
document.querySelector('.btn').style.display = 'none';
To show an element, set the display
property to block
(or any other value except none
):
document.querySelector('.btn').style.display = 'block';
Using Style visibility
Property
Another way to show or hide DOM elements in JavaScript is by using the style visibility
property. It is similar to the above display
property. However, if you set display: none
, it hides the entire element from the DOM, while visibility:hidden
hides the contents of the element, but the element stays in its original position and size.
To hide an element, set the visibility
property to hidden
:
document.querySelector('.btn').style.visibility = 'hidden';
To shown the element again, simply set the visibility
property to visible
like below:
document.querySelector('.btn').style.visibility = 'visible';
The style visibility
property only hides the element but doesn't remove the space occupied by the element. If you want to remove the space too, you have to set display: none
using the display
property.
Creating show()
and hide()
Methods
jQuery provides hide()
, show()
, and toggle()
utility methods that use inline styles to update the display
property of the element.
Let us use the style
property to create the above jQuery methods in vanilla JavaScript:
// hide an element
const hide = (elem) => {
elem.style.display = 'none';
}
// show an element
const show = (elem) => {
elem.style.display = 'block';
}
// toggle the element visibility
const toggle = (elem) => {
// if the element is visible, hide it
if(window.getComputedStyle(elem).display !== 'none') {
hide(elem);
return;
}
// show the element
show(elem);
}
Now to hide or show any DOM element, just use the above methods like below:
// hide element
hide(document.querySelector('.btn'));
// show element
show(document.querySelector('.btn'));
// toggle visibility
toggle(document.querySelector('.btn'));
Notice the use of the getComputedStyle()
method, which we just learned the other day, to check if an element is already visible.
We used this method because the style
property only deals with inline styles specified using the element's style
attribute. But the element could be hidden through an embedded <style>
element or even an external stylesheet. The getComputedStyle()
method returns the actual CSS styles that were used to render an HTML element, regardless of how those styles were defined.
Another thing to notice is the getComputedStyle(elem).display !== 'none'
statement. We are not checking whether the display type is block
because block
is not the only way to show an element. You could use flex
, inline-block
, grid
, table
, etc. for the display
property to show an element. However, to hide an element, there is only one value which is display: none
.
If you prefer to use a CSS class to hide and show DOM elements instead of inline styles, take a look at this guide.
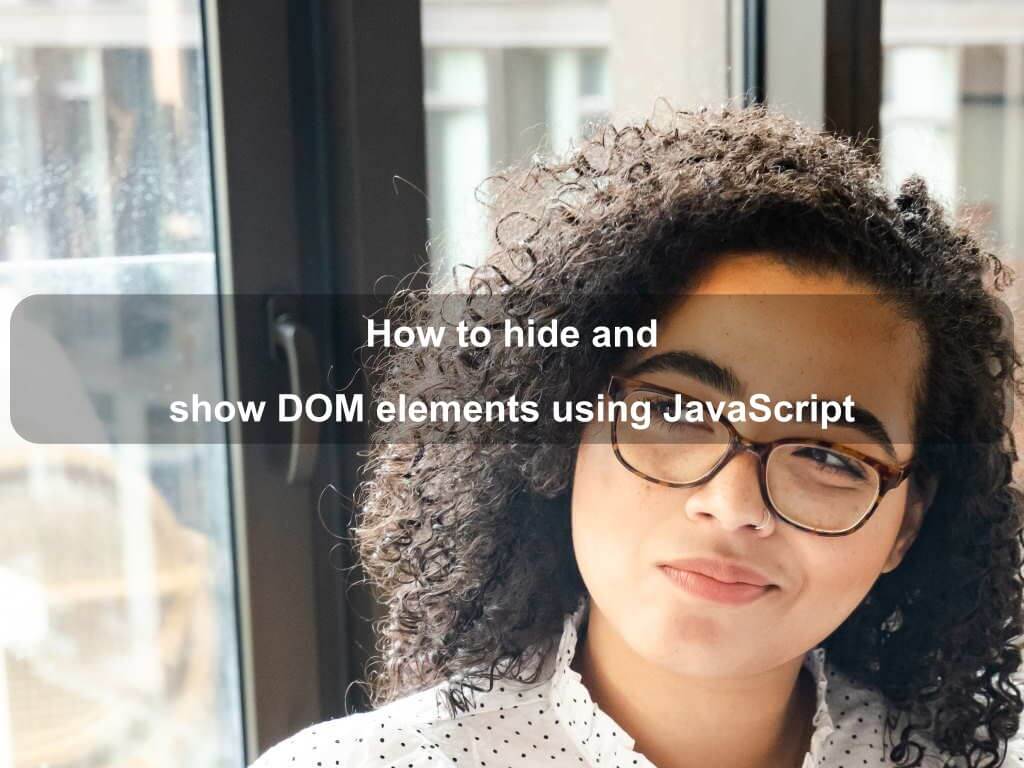
Are we missing something? Help us improve this article. Reach out to us.
How to hide and show DOM elements using JavaScript
There are multiple ways to show or hide DOM elements in vanilla JavaScript. In this article, we'll look at two different ways to hide or show DOM elements using JavaScript.
Using Style display
Property
The style display
property is used to set as well as get the element's display type in JavaScript.
Majority of the HTML elements have the inline or block display type. The content of an inline element floats to its left and right sides. Block HTML elements are different because they * fill* the entire line, and do not show content on their sides.
To hide an element, set the display
property to none
:
document.querySelector('.btn').style.display = 'none';
To show an element, set the display
property to block
(or any other value except none
):
document.querySelector('.btn').style.display = 'block';
Using Style visibility
Property
Another way to show or hide DOM elements in JavaScript is by using the style visibility
property. It is similar to the above display
property. However, if you set display: none
, it hides the entire element from the DOM, while visibility:hidden
hides the contents of the element, but the element stays in its original position and size.
To hide an element, set the visibility
property to hidden
:
document.querySelector('.btn').style.visibility = 'hidden';
To shown the element again, simply set the visibility
property to visible
like below:
document.querySelector('.btn').style.visibility = 'visible';
The style visibility
property only hides the element but doesn't remove the space occupied by the element. If you want to remove the space too, you have to set display: none
using the display
property.
Creating show()
and hide()
Methods
jQuery provides hide()
, show()
, and toggle()
utility methods that use inline styles to update the display
property of the element.
Let us use the style
property to create the above jQuery methods in vanilla JavaScript:
// hide an element
const hide = (elem) => {
elem.style.display = 'none';
}
// show an element
const show = (elem) => {
elem.style.display = 'block';
}
// toggle the element visibility
const toggle = (elem) => {
// if the element is visible, hide it
if(window.getComputedStyle(elem).display !== 'none') {
hide(elem);
return;
}
// show the element
show(elem);
}
Now to hide or show any DOM element, just use the above methods like below:
// hide element
hide(document.querySelector('.btn'));
// show element
show(document.querySelector('.btn'));
// toggle visibility
toggle(document.querySelector('.btn'));
Notice the use of the getComputedStyle()
method, which we just learned the other day, to check if an element is already visible.
We used this method because the style
property only deals with inline styles specified using the element's style
attribute. But the element could be hidden through an embedded <style>
element or even an external stylesheet. The getComputedStyle()
method returns the actual CSS styles that were used to render an HTML element, regardless of how those styles were defined.
Another thing to notice is the getComputedStyle(elem).display !== 'none'
statement. We are not checking whether the display type is block
because block
is not the only way to show an element. You could use flex
, inline-block
, grid
, table
, etc. for the display
property to show an element. However, to hide an element, there is only one value which is display: none
.
If you prefer to use a CSS class to hide and show DOM elements instead of inline styles, take a look at this guide.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur