published: 13 Jun 2022
2 min read
How to get the length of an object in JavaScript
To get the length of an object in JavaScript:
- Pass the object to the
Object.keys()
method as a parameter. - The
Object.keys()
method returns an array of a given object's own enumerable property names. - Use the
length
property to get the number of elements in that array.
Here is an example:
const user = {
name: 'John Doe',
age: 23,
country: 'United States'
}
const length = Object.keys(user).length
console.log('Object length is ${length}')
// Object length is 3
In the above code, we used the Object.keys()
method to get an array of the object's keys. The length
property of the keys array returns the count of key-value pairs in the object.
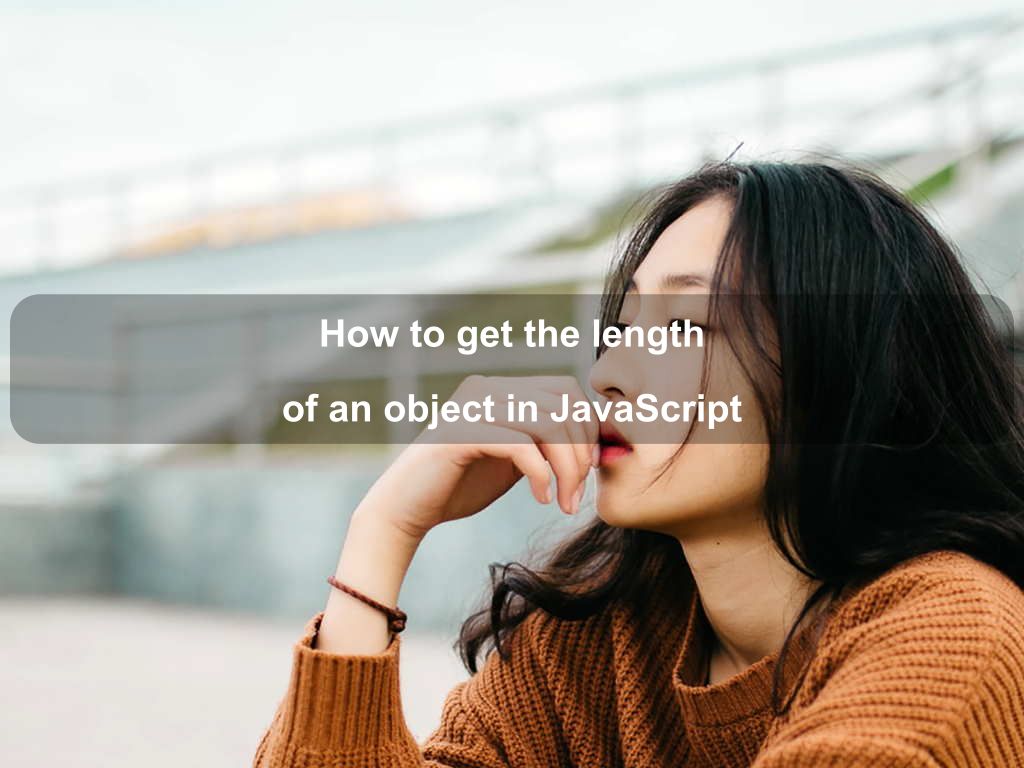
Are we missing something? Help us improve this article. Reach out to us.
How to get the length of an object in JavaScript
To get the length of an object in JavaScript:
- Pass the object to the
Object.keys()
method as a parameter. - The
Object.keys()
method returns an array of a given object's own enumerable property names. - Use the
length
property to get the number of elements in that array.
Here is an example:
const user = {
name: 'John Doe',
age: 23,
country: 'United States'
}
const length = Object.keys(user).length
console.log('Object length is ${length}')
// Object length is 3
In the above code, we used the Object.keys()
method to get an array of the object's keys. The length
property of the keys array returns the count of key-value pairs in the object.
Are you looking for other code tips?
Check out what's on in the category: javascript, programming
JS Nooby
Javascript connoisseur