published: 10 Apr 2022
2 min read
How to get query string parameters in JavaScript
In an earlier article, we looked at how to add and update query string parameters using URLSearchParams
in JavaScript. Today, you'll learn how to get query string parameters from the URL in JavaScript.
To get the full query string from the current browser URL, you can use window.location.search
:
// URL: http://example.com?size=M&size=XL&price=29&sort=desc
console.log(window.location.search);
// ?size=M&size=XL&price=29&sort=desc
To create an instance of URLSearchParams
, just pass the full query string to its constructor:
const params = new URLSearchParams(window.location.search);
URLSearchParams
provides several methods that you can use to manipulate query string parameters:
// get parameter
params.get('price'); // 29
// get multi-valued parameter
params.getAll('size'); // [ 'M', 'XL' ]
// check if parameter exists
params.has('sort'); // true
// iterate over all parameters
for (const p of params) {
console.log(p);
}
// [ 'size', 'M' ]
// [ 'size', 'XL' ]
// [ 'price', '29' ]
// [ 'sort', 'desc' ]
While URLSearchParams
is an ideal solution to work with query strings, it is not supported by all browsers. If you want to support old browsers like Internet Explorer, there is a polyfill available.
Alternatively, you could write a small JavaScript function that takes in a query string parameter name and returns its value:
function queryParam(name) {
name = name.replace(/[\[]/, '\\[').replace(/[\]]/, '\\]');
var regex = new RegExp('[\\?&]' + name + '=([^&#]*)');
var results = regex.exec(window.location.search);
return results === null ? '' : decodeURIComponent(results[1].replace(/\+/g, ' '));
}
queryParam('price'); // 29
queryParam('sort'); // desc
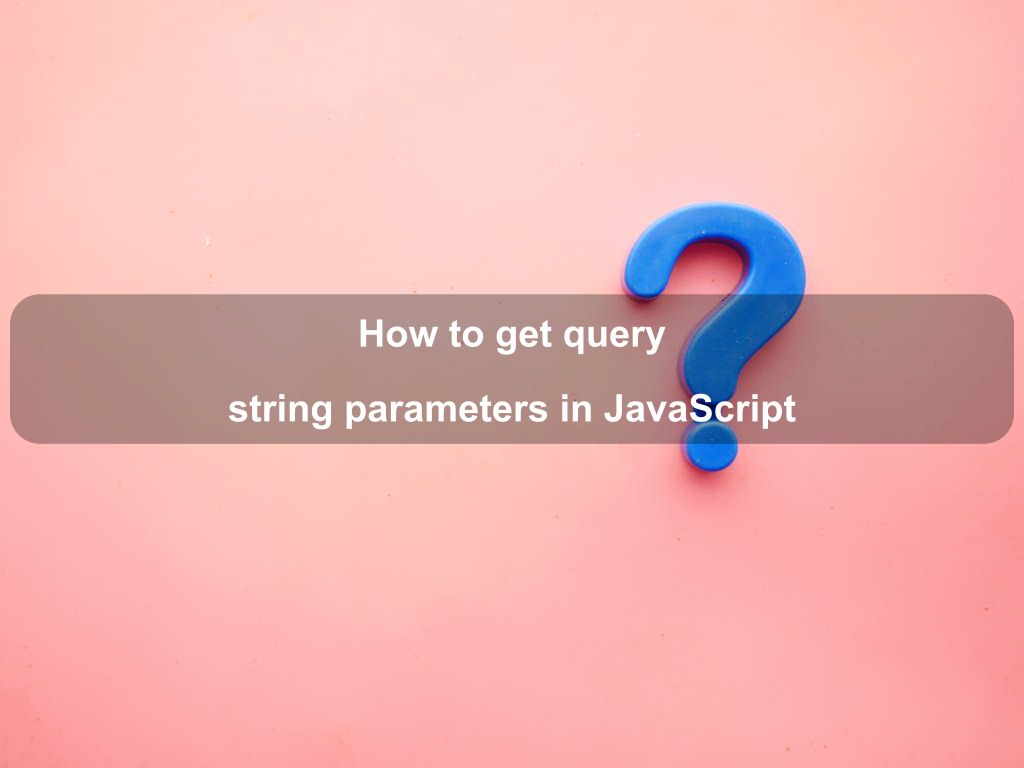
Are we missing something? Help us improve this article. Reach out to us.
How to get query string parameters in JavaScript
In an earlier article, we looked at how to add and update query string parameters using URLSearchParams
in JavaScript. Today, you'll learn how to get query string parameters from the URL in JavaScript.
To get the full query string from the current browser URL, you can use window.location.search
:
// URL: http://example.com?size=M&size=XL&price=29&sort=desc
console.log(window.location.search);
// ?size=M&size=XL&price=29&sort=desc
To create an instance of URLSearchParams
, just pass the full query string to its constructor:
const params = new URLSearchParams(window.location.search);
URLSearchParams
provides several methods that you can use to manipulate query string parameters:
// get parameter
params.get('price'); // 29
// get multi-valued parameter
params.getAll('size'); // [ 'M', 'XL' ]
// check if parameter exists
params.has('sort'); // true
// iterate over all parameters
for (const p of params) {
console.log(p);
}
// [ 'size', 'M' ]
// [ 'size', 'XL' ]
// [ 'price', '29' ]
// [ 'sort', 'desc' ]
While URLSearchParams
is an ideal solution to work with query strings, it is not supported by all browsers. If you want to support old browsers like Internet Explorer, there is a polyfill available.
Alternatively, you could write a small JavaScript function that takes in a query string parameter name and returns its value:
function queryParam(name) {
name = name.replace(/[\[]/, '\\[').replace(/[\]]/, '\\]');
var regex = new RegExp('[\\?&]' + name + '=([^&#]*)');
var results = regex.exec(window.location.search);
return results === null ? '' : decodeURIComponent(results[1].replace(/\+/g, ' '));
}
queryParam('price'); // 29
queryParam('sort'); // desc
Are you looking for other code tips?
JS Nooby
Javascript connoisseur