published: 13 Jul 2022
2 min read
How to get all query string parameters as an object in JavaScript
In JavaScript, you can use the URLSearchParams
interface to convert a query string into an object. It provides utility methods to work with the query string of a URL.
- Pass the query string to the
URLSearchParams
constructor to turn it into an object instance. - Use the
get()
method to access query string parameters. - To get a native JavaScript object, pass the object instance to the
Object.fromEntries()
method.
const qs = '?size=M&price=29&sort=desc'
const params = new URLSearchParams(qs)
console.log(params.get('size')) // M
console.log(params.get('price')) // 29
console.log(params.get('sort')) // desc
// Convert to native JS object
const obj = Object.fromEntries(params)
console.log(obj)
// { size: 'M', price: '29', sort: 'desc' }
In the web browser, just pass window.location.search
to the URLSearchParams
constructor to turn the query string into an object:
const params = new URLSearchParams(window.location.search)
If you have an entire URL, create a new URL object to retrieve the query string, and then pass it to the URLSearchParams
constructor:
const url = new URL('http://example.com?size=M&price=29&sort=desc')
const params = new URLSearchParams(url.search)
The Object.fromEntries()
function works fine as long as there are no duplicate query string parameters. If you have something like ?size=M&size=XL
, you'll only get { size: 'XL' }
.
To handle duplicate query string parameters, use the following approach instead:
const qs = '?size=M&size=XL&price=29&sort=desc'
const params = new URLSearchParams(qs)
const obj = {}
for (const key of params.keys()) {
if (params.getAll(key).length > 1) {
obj[key] = params.getAll(key)
} else {
obj[key] = params.get(key)
}
}
console.log(obj)
// { size: [ 'M', 'XL' ], price: '29', sort: 'desc' }
Read this article to learn how to convert an object to a query string in JavaScript.
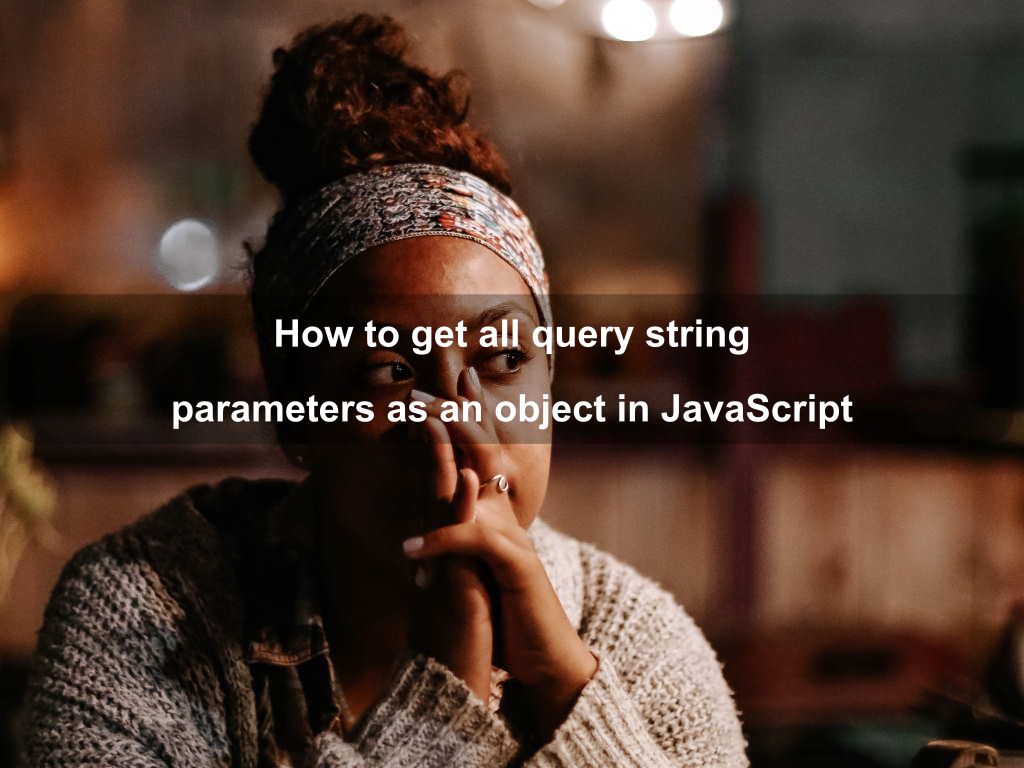
Are we missing something? Help us improve this article. Reach out to us.
How to get all query string parameters as an object in JavaScript
In JavaScript, you can use the URLSearchParams
interface to convert a query string into an object. It provides utility methods to work with the query string of a URL.
- Pass the query string to the
URLSearchParams
constructor to turn it into an object instance. - Use the
get()
method to access query string parameters. - To get a native JavaScript object, pass the object instance to the
Object.fromEntries()
method.
const qs = '?size=M&price=29&sort=desc'
const params = new URLSearchParams(qs)
console.log(params.get('size')) // M
console.log(params.get('price')) // 29
console.log(params.get('sort')) // desc
// Convert to native JS object
const obj = Object.fromEntries(params)
console.log(obj)
// { size: 'M', price: '29', sort: 'desc' }
In the web browser, just pass window.location.search
to the URLSearchParams
constructor to turn the query string into an object:
const params = new URLSearchParams(window.location.search)
If you have an entire URL, create a new URL object to retrieve the query string, and then pass it to the URLSearchParams
constructor:
const url = new URL('http://example.com?size=M&price=29&sort=desc')
const params = new URLSearchParams(url.search)
The Object.fromEntries()
function works fine as long as there are no duplicate query string parameters. If you have something like ?size=M&size=XL
, you'll only get { size: 'XL' }
.
To handle duplicate query string parameters, use the following approach instead:
const qs = '?size=M&size=XL&price=29&sort=desc'
const params = new URLSearchParams(qs)
const obj = {}
for (const key of params.keys()) {
if (params.getAll(key).length > 1) {
obj[key] = params.getAll(key)
} else {
obj[key] = params.get(key)
}
}
console.log(obj)
// { size: [ 'M', 'XL' ], price: '29', sort: 'desc' }
Read this article to learn how to convert an object to a query string in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur