published: 25 Aug 2022
2 min read
How to get all own properties of an object in JavaScript
To get all own properties of an object in JavaScript, you can use the Object.getOwnPropertyNames()
method.
This method returns an array containing all the names of the enumerable and non-enumerable own properties found directly on the object passed in as an argument.
The Object.getOwnPropertyNames()
method does not look for the inherited properties.
Here is an example:
const user = {
name: 'Alex',
age: 30
}
const props = Object.getOwnPropertyNames(user)
console.log(props) // [ 'name', 'age' ]
If you are interested in the own enumerable properties of the object, use the Object.keys() method instead:
const user = {
name: 'Alex',
age: 30
}
const props = Object.keys(user)
console.log(props) // [ 'name', 'age' ]
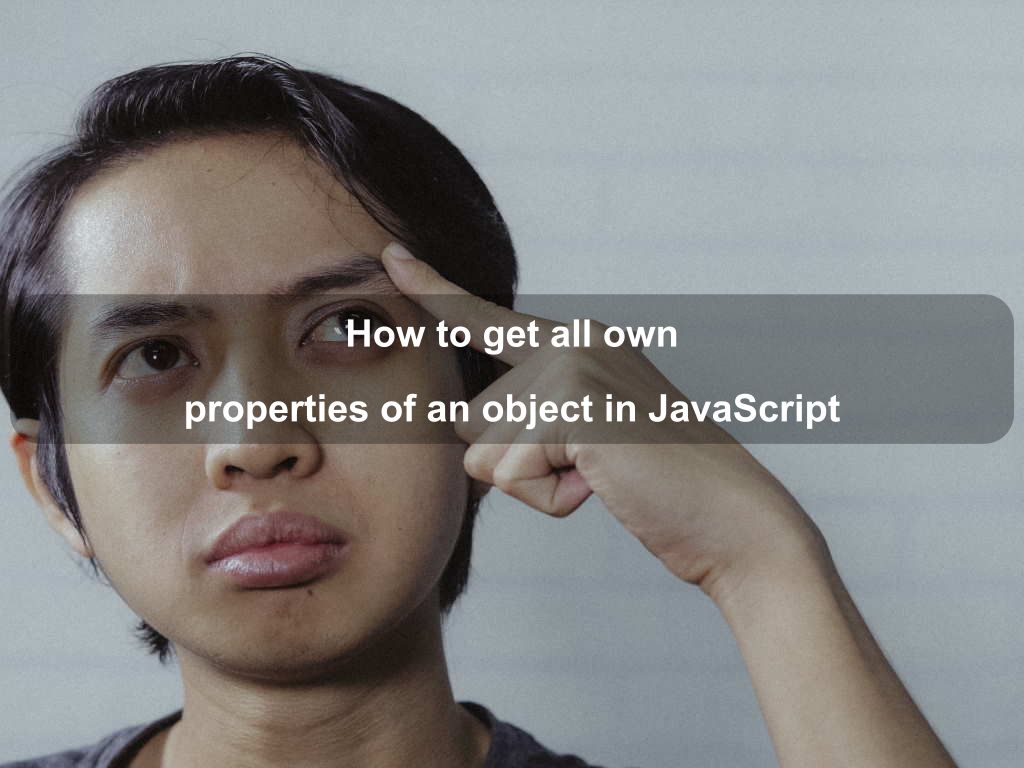
Are we missing something? Help us improve this article. Reach out to us.
How to get all own properties of an object in JavaScript
To get all own properties of an object in JavaScript, you can use the Object.getOwnPropertyNames()
method.
This method returns an array containing all the names of the enumerable and non-enumerable own properties found directly on the object passed in as an argument.
The Object.getOwnPropertyNames()
method does not look for the inherited properties.
Here is an example:
const user = {
name: 'Alex',
age: 30
}
const props = Object.getOwnPropertyNames(user)
console.log(props) // [ 'name', 'age' ]
If you are interested in the own enumerable properties of the object, use the Object.keys() method instead:
const user = {
name: 'Alex',
age: 30
}
const props = Object.keys(user)
console.log(props) // [ 'name', 'age' ]
Are you looking for other code tips?
JS Nooby
Javascript connoisseur