published: 29 Aug 2022
2 min read
How to get a random value from an array with JavaScript
To select a random value from an array in JavaScript, you can use the built-in Math
object functions. Let us say we want to create a function that selects a random fruit from an array of fruits.
Here is how our fruits array looks like:
const fruits = [
'Apple',
'Orange',
'Mango',
'Banana',
'Cherry'
];
Next, let us write a randomValue()
function that randomly picks a value from the given list:
const randomValue = (list) => {
return list[Math.floor(Math.random() * list.length)];
};
Now you can use the above function to select a random value from an array, like below:
console.log(randomValue(fruits)); // Banana
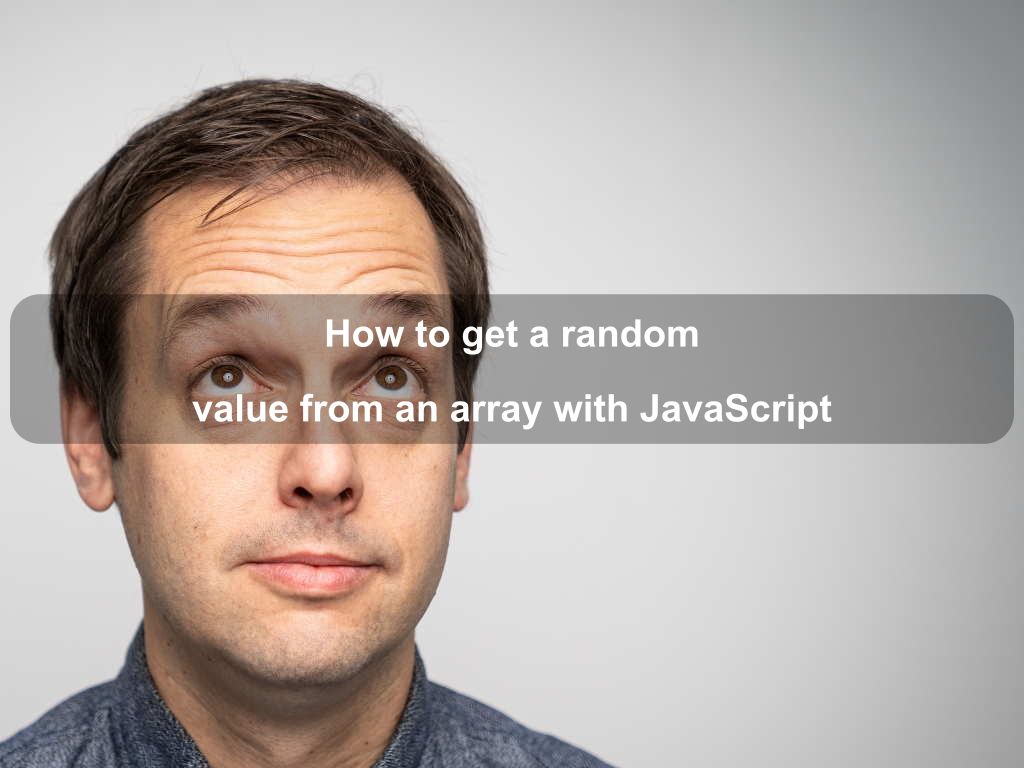
Are we missing something? Help us improve this article. Reach out to us.
How to get a random value from an array with JavaScript
To select a random value from an array in JavaScript, you can use the built-in Math
object functions. Let us say we want to create a function that selects a random fruit from an array of fruits.
Here is how our fruits array looks like:
const fruits = [
'Apple',
'Orange',
'Mango',
'Banana',
'Cherry'
];
Next, let us write a randomValue()
function that randomly picks a value from the given list:
const randomValue = (list) => {
return list[Math.floor(Math.random() * list.length)];
};
Now you can use the above function to select a random value from an array, like below:
console.log(randomValue(fruits)); // Banana
Are you looking for other code tips?
JS Nooby
Javascript connoisseur