published: 16 Jul 2022
2 min read
How to generate random numbers in JavaScript
In JavaScript, you can use the Math. random()
function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive).
const random = Math.random();
console.log(random);
// 0.5362036769798451
If you want to get a random number between 0 and 20, just multiply the results of Math.random()
by 20:
const random = Math.random() * 20;
console.log(random);
// 15.40476356200032
To generate a random whole number, you can use the following Math
methods along with Math.random()
:
Math.ceil()
- Rounds a number upwards to the nearest integerMath.floor()
- Rounds a number downwards to the nearest integerMath.round()
- Rounds a number to the nearest integer
Let us use Math.floor()
to round the floating number generated by Math.random()
to a whole number:
const random = Math.floor(Math.random() * 20);
console.log(random);
// 12
Now we have learned that how to generate a whole random number, let us write a function that takes in an integer as input and returns a whole number between 0 and the integer itself:
const random = (max = 50) => {
return Math.floor(Math.random() * max);
};
console.log(random(100));
// 66
To generate a random number between two specific numbers (min
included, max
excluded), we have to update the random()
method like below:
const random = (min = 0, max = 50) => {
let num = Math.random() * (max - min) + min;
return Math.floor(num);
};
console.log(random(10, 40));
// 28
In the above code, we used (max - min) + min
to avoid cases where the max
number is less than the min
number.
To generate a random number that includes both min
and max
values, just change Math.floor()
to Math.round()
:
const random = (min = 0, max = 50) => {
let num = Math.random() * (max - min) + min;
return Math.round(num);
};
console.log(random(10, 70));
// 51
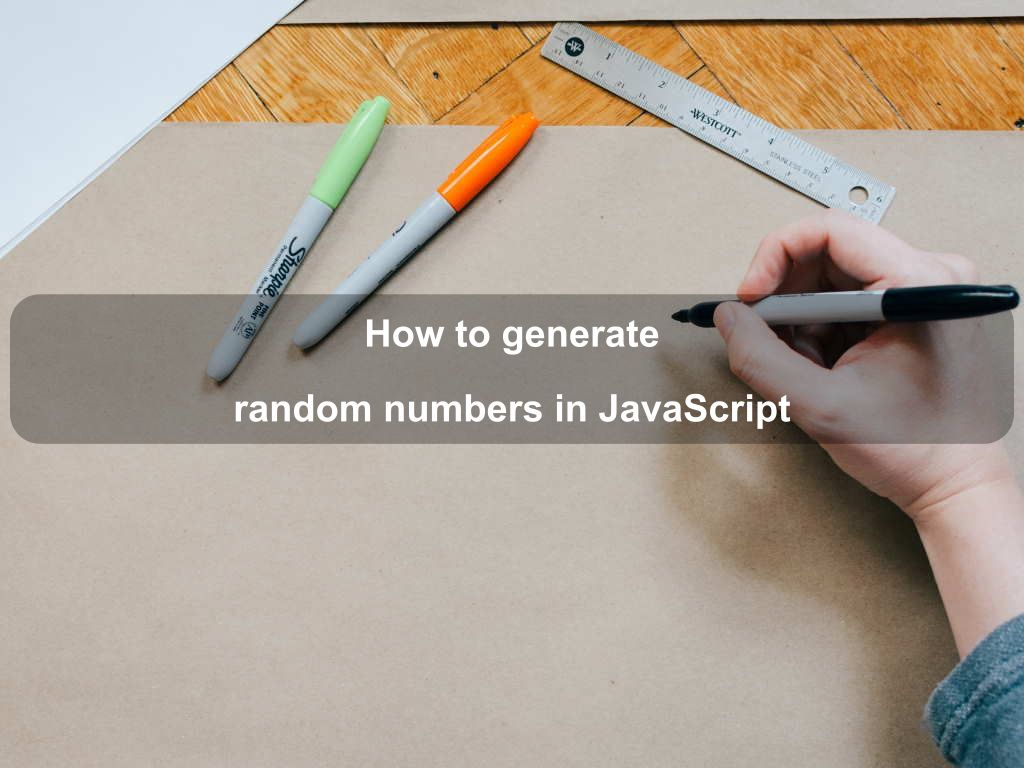
Are we missing something? Help us improve this article. Reach out to us.
How to generate random numbers in JavaScript
In JavaScript, you can use the Math. random()
function to generate a pseudo-random floating number between 0 (inclusive) and 1 (exclusive).
const random = Math.random();
console.log(random);
// 0.5362036769798451
If you want to get a random number between 0 and 20, just multiply the results of Math.random()
by 20:
const random = Math.random() * 20;
console.log(random);
// 15.40476356200032
To generate a random whole number, you can use the following Math
methods along with Math.random()
:
Math.ceil()
- Rounds a number upwards to the nearest integerMath.floor()
- Rounds a number downwards to the nearest integerMath.round()
- Rounds a number to the nearest integer
Let us use Math.floor()
to round the floating number generated by Math.random()
to a whole number:
const random = Math.floor(Math.random() * 20);
console.log(random);
// 12
Now we have learned that how to generate a whole random number, let us write a function that takes in an integer as input and returns a whole number between 0 and the integer itself:
const random = (max = 50) => {
return Math.floor(Math.random() * max);
};
console.log(random(100));
// 66
To generate a random number between two specific numbers (min
included, max
excluded), we have to update the random()
method like below:
const random = (min = 0, max = 50) => {
let num = Math.random() * (max - min) + min;
return Math.floor(num);
};
console.log(random(10, 40));
// 28
In the above code, we used (max - min) + min
to avoid cases where the max
number is less than the min
number.
To generate a random number that includes both min
and max
values, just change Math.floor()
to Math.round()
:
const random = (min = 0, max = 50) => {
let num = Math.random() * (max - min) + min;
return Math.round(num);
};
console.log(random(10, 70));
// 51
Are you looking for other code tips?
JS Nooby
Javascript connoisseur