published: 06 Sep 2022
2 min read
How to generate a random string in JavaScript
There are many ways available to generate a random string in JavaScript. The quickest way is to use the Math.random()
method.
The Math.random()
method returns a random number between 0 (inclusive), and 1 (exclusive). You can convert this random number to a string and then remove the trailing zeros:
const rand = Math.random().toString().substr(2, 8); // 60502138
The above code will generate a random string of 8 characters that will contain numbers only.
To generate an alpha-numeric string, you can pass an integer value between 2
and 36
to the toString()
method called radix
. It defines the base to use for representing a numeric value.
For a binary string (0-1), you can pass 2
as radix
to toString()
:
const binary = Math.random().toString(2).substr(2, 8); // 01100110
To generate a fully random string, you should pass 16
or greater as a radix
value to toString()
:
const rand = Math.random().toString(16).substr(2, 8); // 6de5ccda
Let us write a function by using the above code to generate a random string anywhere between 0 and 14 characters:
const random = (length = 8) => {
return Math.random().toString(16).substr(2, length);
};
console.log(random()); // bb325d9f
console.log(random(6)); // e51d83
console.log(random(10)); // e84c416cc7
console.log(random(14)); // ee16dfc68e361
Generate large random strings
To generate large random strings (14+ characters long), you have to write your own generator. The following example demonstrates how you can generate strings of any size by picking characters randomly from A-Z
, a-z
, and 0-9
:
const random = (length = 8) => {
// Declare all characters
let chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
// Pick characers randomly
let str = '';
for (let i = 0; i < length; i++) {
str += chars.charAt(Math.floor(Math.random() * chars.length));
}
return str;
};
console.log(random()); // JgKGQEUx
console.log(random(12)); // ttwbeshkYzaX
console.log(random(20)); // zZN7uH9pPjhJf30QNus5
In the above example, Math.random()
and Math.floor()
methods are used to generate a random index of the character in the specified characters (A-Z a-z 0-9).
The for loop is used to loop through the number passed into the random()
function. During each iteration of the loop, a random character is selected from the characters' list.
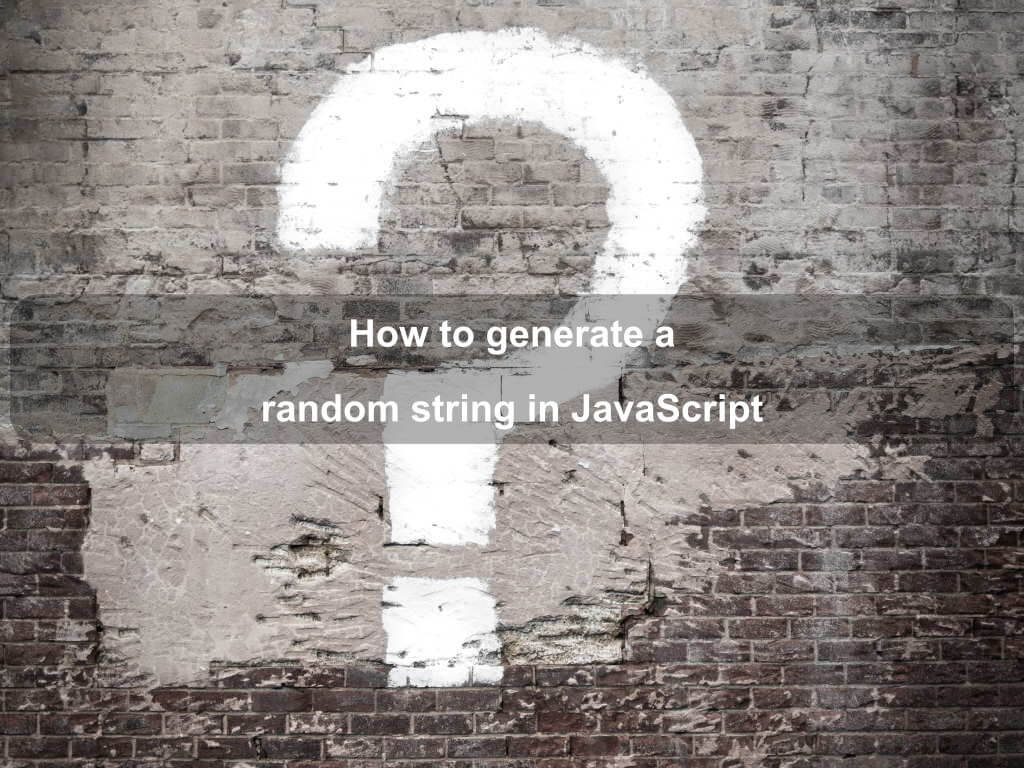
Are we missing something? Help us improve this article. Reach out to us.
How to generate a random string in JavaScript
There are many ways available to generate a random string in JavaScript. The quickest way is to use the Math.random()
method.
The Math.random()
method returns a random number between 0 (inclusive), and 1 (exclusive). You can convert this random number to a string and then remove the trailing zeros:
const rand = Math.random().toString().substr(2, 8); // 60502138
The above code will generate a random string of 8 characters that will contain numbers only.
To generate an alpha-numeric string, you can pass an integer value between 2
and 36
to the toString()
method called radix
. It defines the base to use for representing a numeric value.
For a binary string (0-1), you can pass 2
as radix
to toString()
:
const binary = Math.random().toString(2).substr(2, 8); // 01100110
To generate a fully random string, you should pass 16
or greater as a radix
value to toString()
:
const rand = Math.random().toString(16).substr(2, 8); // 6de5ccda
Let us write a function by using the above code to generate a random string anywhere between 0 and 14 characters:
const random = (length = 8) => {
return Math.random().toString(16).substr(2, length);
};
console.log(random()); // bb325d9f
console.log(random(6)); // e51d83
console.log(random(10)); // e84c416cc7
console.log(random(14)); // ee16dfc68e361
Generate large random strings
To generate large random strings (14+ characters long), you have to write your own generator. The following example demonstrates how you can generate strings of any size by picking characters randomly from A-Z
, a-z
, and 0-9
:
const random = (length = 8) => {
// Declare all characters
let chars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
// Pick characers randomly
let str = '';
for (let i = 0; i < length; i++) {
str += chars.charAt(Math.floor(Math.random() * chars.length));
}
return str;
};
console.log(random()); // JgKGQEUx
console.log(random(12)); // ttwbeshkYzaX
console.log(random(20)); // zZN7uH9pPjhJf30QNus5
In the above example, Math.random()
and Math.floor()
methods are used to generate a random index of the character in the specified characters (A-Z a-z 0-9).
The for loop is used to loop through the number passed into the random()
function. During each iteration of the loop, a random character is selected from the characters' list.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur