published: 02 Sep 2022
2 min read
How to format a number as a currency string in JavaScript
The easiest and the most popular way to format numbers to currency strings in JavaScript is by using the Internationalization API. It provides methods for language-sensitive string comparison, number formatting, and the date and time formatting.
One of these methods is Intl.NumberFormat()
, which lets us format numbers using a locale of our own choice.
The Intl.NumberFormat()
constructor accepts two parameters. The first parameter is the locale string like en-US
or de-DE
. The second parameter is an object to specify the options you want to apply while formatting:
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2,
maximumFractionDigits: 2
})
console.log(formatter.format(100)) // $100.00
console.log(formatter.format(1255.786)) // $1,255.79
console.log(formatter.format(14567890)) // $14,567,890.00
In the options object, the style
property is used to specify the type of formatting. It has the following possible values:
decimal
for plain number formatting.currency
for currency formatting.unit
for unit formatting.percent
for percent formatting.
The default style
property value is decimal
. In the above example, we used currency
to format the number as a currency string.
The currency
property lets you define the currency to use in currency formatting, such as USD
, EUR
, or CAD
.
Finally, the maximumSignificantDigits
and maximumSignificantDigits
properties set minimum and maximum fraction digits to use while formatting.
That being said, let us write a generic function that accepts a number value, an optional currency, locale, minimum, and maximum fraction digits and returns a formatted currency string:
const currency = (amount, currency = 'USD', locale = 'en-US', minfd = 2, maxfd = 2) => {
return new Intl.NumberFormat(locale, {
style: 'currency',
currency: currency,
minimumFractionDigits: minfd,
maximumFractionDigits: maxfd
}).format(amount)
}
Now we can use the above currency()
method to format any number into a currency string:
console.log(currency(100)) // $100.00
console.log(currency(14567890, 'CAD')) // CA$14,567,890.00
console.log(currency(1255.786, 'EUR', 'de-DE')) // 1.255,79 €
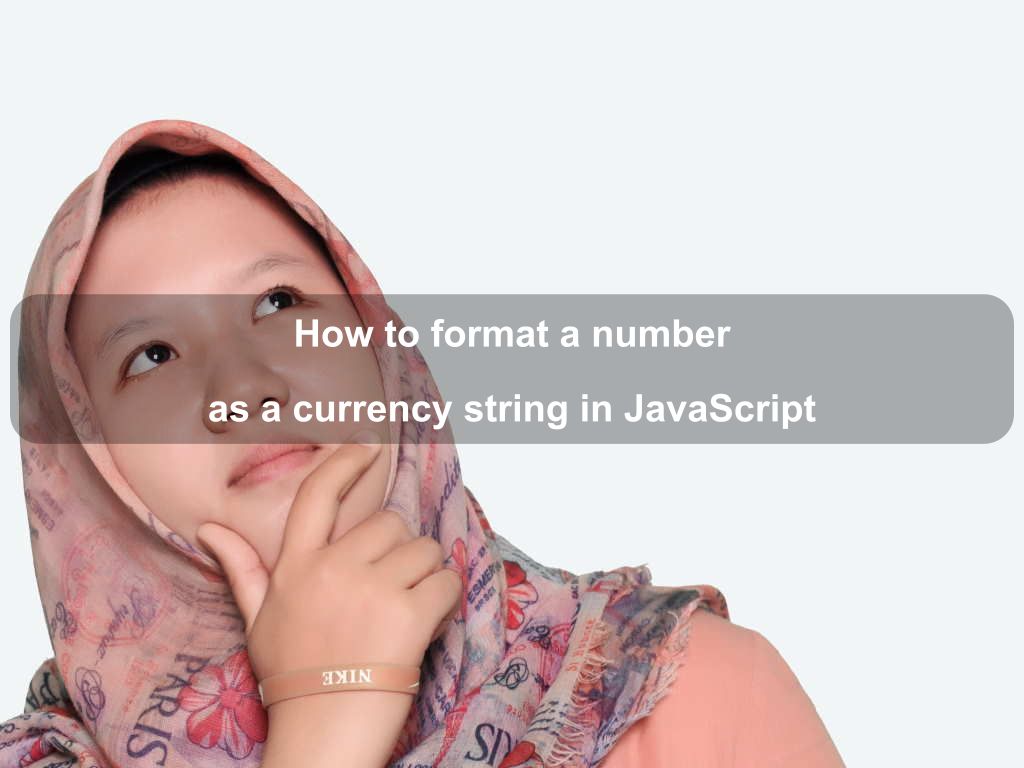
Are we missing something? Help us improve this article. Reach out to us.
How to format a number as a currency string in JavaScript
The easiest and the most popular way to format numbers to currency strings in JavaScript is by using the Internationalization API. It provides methods for language-sensitive string comparison, number formatting, and the date and time formatting.
One of these methods is Intl.NumberFormat()
, which lets us format numbers using a locale of our own choice.
The Intl.NumberFormat()
constructor accepts two parameters. The first parameter is the locale string like en-US
or de-DE
. The second parameter is an object to specify the options you want to apply while formatting:
const formatter = new Intl.NumberFormat('en-US', {
style: 'currency',
currency: 'USD',
minimumFractionDigits: 2,
maximumFractionDigits: 2
})
console.log(formatter.format(100)) // $100.00
console.log(formatter.format(1255.786)) // $1,255.79
console.log(formatter.format(14567890)) // $14,567,890.00
In the options object, the style
property is used to specify the type of formatting. It has the following possible values:
decimal
for plain number formatting.currency
for currency formatting.unit
for unit formatting.percent
for percent formatting.
The default style
property value is decimal
. In the above example, we used currency
to format the number as a currency string.
The currency
property lets you define the currency to use in currency formatting, such as USD
, EUR
, or CAD
.
Finally, the maximumSignificantDigits
and maximumSignificantDigits
properties set minimum and maximum fraction digits to use while formatting.
That being said, let us write a generic function that accepts a number value, an optional currency, locale, minimum, and maximum fraction digits and returns a formatted currency string:
const currency = (amount, currency = 'USD', locale = 'en-US', minfd = 2, maxfd = 2) => {
return new Intl.NumberFormat(locale, {
style: 'currency',
currency: currency,
minimumFractionDigits: minfd,
maximumFractionDigits: maxfd
}).format(amount)
}
Now we can use the above currency()
method to format any number into a currency string:
console.log(currency(100)) // $100.00
console.log(currency(14567890, 'CAD')) // CA$14,567,890.00
console.log(currency(1255.786, 'EUR', 'de-DE')) // 1.255,79 €
Are you looking for other code tips?
JS Nooby
Javascript connoisseur