published: 05 May 2022
2 min read
How to decode a URL using JavaScript
In an earlier article, we looked at different ways to encode a URL in JavaScript. In this article, you'll learn how to decode an encoded URL in JavaScript.
URL decoding is the opposite of the encoding process. It converts the encoded URL strings and query parameters back to their normal formats. Most of the time, encoded query string parameters are automatically decoded by the underlying framework you're using like Express or Spring Boot. However, in standalone applications, you have to manually decode query strings.
Let us look at the JavaScript native functions that can be used for this purpose.
decodeURI()
The decodeURI()
function is used to decode a full URL in JavaScript. It performs the reverse operation of encodeURI()
. Here is an example:
const encodedUrl = 'http://example.com/!leearn%20javascript$/';
// decode complete URL
const url = decodeURI(encodedUrl);
// print decoded URL
console.log(url);
// output: http://example.com/!leearn javascript$/
decodeURIComponent()
The decodeURIComponent()
function is used to decode URL components encoding by encodeURIComponent()
in JavaScript. It uses UTF-8
encoding scheme to perform the decoding operation.
You should use decodeURIComponent()
to decode query string parameters and path segments instead of full URLs. Here is an example:
const query = 'Danke Schon';
// perofrm encode/decode
const encodedStr = encodeURIComponent(query);
const decodedStr = decodeURIComponent(encodedStr);
// print values
console.log('Encoded Query: ${encodedStr}');
console.log('Decoded Query: ${decodedStr}');
// Output
// Encoded Query: Danke%20Sch%C3%B6n
// Decoded Query: Danke Schon
Read Next: Base64 encoding and decoding in JavaScript
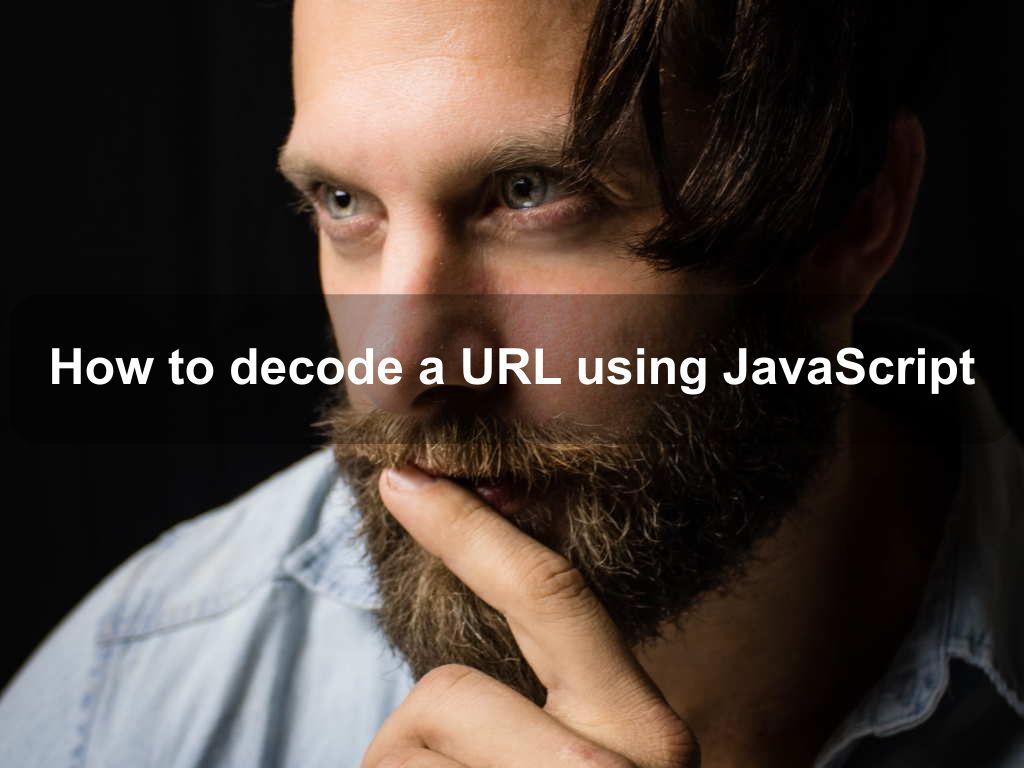
Are we missing something? Help us improve this article. Reach out to us.
How to decode a URL using JavaScript
In an earlier article, we looked at different ways to encode a URL in JavaScript. In this article, you'll learn how to decode an encoded URL in JavaScript.
URL decoding is the opposite of the encoding process. It converts the encoded URL strings and query parameters back to their normal formats. Most of the time, encoded query string parameters are automatically decoded by the underlying framework you're using like Express or Spring Boot. However, in standalone applications, you have to manually decode query strings.
Let us look at the JavaScript native functions that can be used for this purpose.
decodeURI()
The decodeURI()
function is used to decode a full URL in JavaScript. It performs the reverse operation of encodeURI()
. Here is an example:
const encodedUrl = 'http://example.com/!leearn%20javascript$/';
// decode complete URL
const url = decodeURI(encodedUrl);
// print decoded URL
console.log(url);
// output: http://example.com/!leearn javascript$/
decodeURIComponent()
The decodeURIComponent()
function is used to decode URL components encoding by encodeURIComponent()
in JavaScript. It uses UTF-8
encoding scheme to perform the decoding operation.
You should use decodeURIComponent()
to decode query string parameters and path segments instead of full URLs. Here is an example:
const query = 'Danke Schon';
// perofrm encode/decode
const encodedStr = encodeURIComponent(query);
const decodedStr = decodeURIComponent(encodedStr);
// print values
console.log('Encoded Query: ${encodedStr}');
console.log('Decoded Query: ${decodedStr}');
// Output
// Encoded Query: Danke%20Sch%C3%B6n
// Decoded Query: Danke Schon
Read Next: Base64 encoding and decoding in JavaScript
Are you looking for other code tips?
JS Nooby
Javascript connoisseur