published: 29 Aug 2022
2 min read
How to create a DOM element using JavaScript
In JavaScript, you can use the createElement()
method to create a new DOM element. This method takes the element name as input and creates a new HTML element with the given name.
Unlike jQuery's $(...)
method, which takes the element's opening and closing tag as input to create a new element:
const div = $('<div></div>')
The createElement()
method only needs the element name:
const anchor = document.createElement('a')
const div = document.createElement('div')
const article = document.createElement('article')
After the element is created, you can use the innerText
or innerHTML
properties of the element to add text as well as child HTML nodes:
// add text
div.innerText = 'Hey, there!'
// add child HTML nodes
div.innerHTML = '<p>Hello World!!!</p>'
This method is not just limited to HTML tags. You can even create custom tags of your own choice:
const unicorn = document.createElement('unicorn')
// <unicorn></unicorn>
To append the newly created HTML element to the document, JavaScript provides appendChild()
method:
// create a new element
const div = document.createElement('div')
div.innerText = 'Hey, there!'
// append element to body
document.body.appendChild(div)
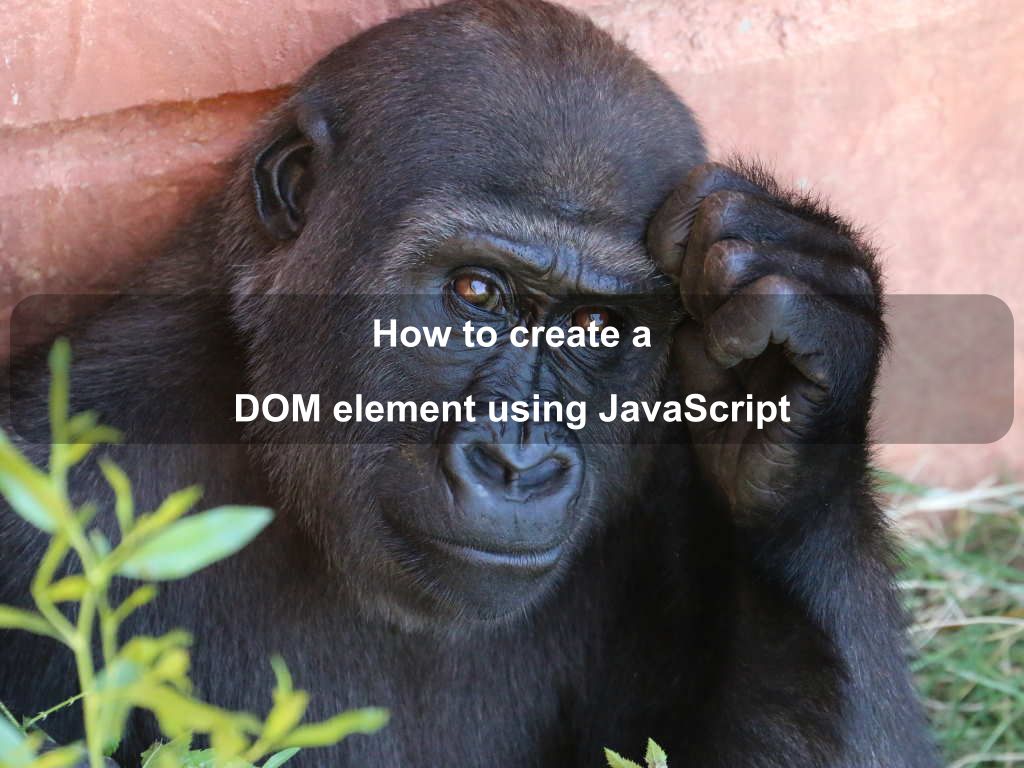
Are we missing something? Help us improve this article. Reach out to us.
How to create a DOM element using JavaScript
In JavaScript, you can use the createElement()
method to create a new DOM element. This method takes the element name as input and creates a new HTML element with the given name.
Unlike jQuery's $(...)
method, which takes the element's opening and closing tag as input to create a new element:
const div = $('<div></div>')
The createElement()
method only needs the element name:
const anchor = document.createElement('a')
const div = document.createElement('div')
const article = document.createElement('article')
After the element is created, you can use the innerText
or innerHTML
properties of the element to add text as well as child HTML nodes:
// add text
div.innerText = 'Hey, there!'
// add child HTML nodes
div.innerHTML = '<p>Hello World!!!</p>'
This method is not just limited to HTML tags. You can even create custom tags of your own choice:
const unicorn = document.createElement('unicorn')
// <unicorn></unicorn>
To append the newly created HTML element to the document, JavaScript provides appendChild()
method:
// create a new element
const div = document.createElement('div')
div.innerText = 'Hey, there!'
// append element to body
document.body.appendChild(div)
Are you looking for other code tips?
JS Nooby
Javascript connoisseur