published: 21 Aug 2022
2 min read
How to convert a NodeList to an array in JavaScript
A NodeList is an array-like object that represents a collection of DOM elements or more specifically nodes. It is just like an array, but you can not use the common array methods like map()
, slice()
, and filter()
on a NodeList
object.
Take a look this guide to understand the difference between a NodeList
and an array.
[...] a
NodeList
is a collection of nodes that can be used to access and manipulate DOM elements, while an array is a JavaScript object which can hold more than one value at a time.Both NodeLists and arrays have their own prototypes, methods, and properties. You can easily convert a
NodeList
to an array if you want to, but not the other way around.
In this article, we'll look at different ways to convert a NodeList
object to an array in JavaScript.
Array.from()
Method
In modern JavaScript, the simplest and easiest way to convert a NodeList
object to an array is by using the Array.from() method:
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = Array.from(divs);
The output of the above code is a normal Array
object containing all the nodes returned by the querySelectorAll()
method.
The Array.from()
method was introduced in ES6 and creates a new, shallow-copied Array
object from the NodeList
object. Unfortunately, it only works in modern browsers. To support old browsers like IE, you need a polyfill.
Spread Operator
Another way to create an Array
object from a NodeList
is by using the spread operator syntax ([...iterable]
):
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = [...divs];
The ES6's spread operator is a concise, and cool way of converting a NodeList
to an array in JavaScript. However, it only works in modern browsers.
Array.prototype.slice()
Method
Finally, the last way to convert a NodeList
to an Array
object is by using the call()
method to execute the Array.prototype.slice()
method on the NodeList
object as shown below:
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = Array.prototype.slice.call(divs);
You can also use a concise form of the above method:
const divsArr = [].slice.call(divs);
The Array.prototype.slice.call()
works well in all modern and old browsers including IE 6+. You should use this approach if you want to support the most number of browsers.
All of the above methods give a real JavaScript array, which you can iterate through using forEach() and use all your favorite array methods to do different things.
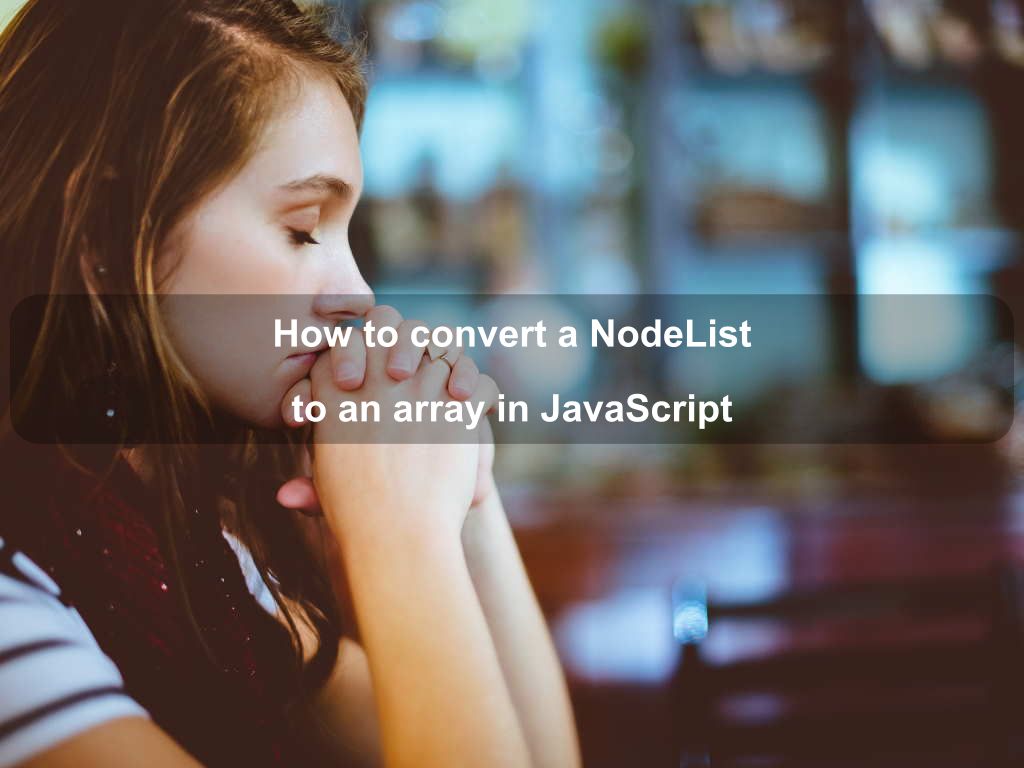
Are we missing something? Help us improve this article. Reach out to us.
How to convert a NodeList to an array in JavaScript
A NodeList is an array-like object that represents a collection of DOM elements or more specifically nodes. It is just like an array, but you can not use the common array methods like map()
, slice()
, and filter()
on a NodeList
object.
Take a look this guide to understand the difference between a NodeList
and an array.
[...] a
NodeList
is a collection of nodes that can be used to access and manipulate DOM elements, while an array is a JavaScript object which can hold more than one value at a time.Both NodeLists and arrays have their own prototypes, methods, and properties. You can easily convert a
NodeList
to an array if you want to, but not the other way around.
In this article, we'll look at different ways to convert a NodeList
object to an array in JavaScript.
Array.from()
Method
In modern JavaScript, the simplest and easiest way to convert a NodeList
object to an array is by using the Array.from() method:
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = Array.from(divs);
The output of the above code is a normal Array
object containing all the nodes returned by the querySelectorAll()
method.
The Array.from()
method was introduced in ES6 and creates a new, shallow-copied Array
object from the NodeList
object. Unfortunately, it only works in modern browsers. To support old browsers like IE, you need a polyfill.
Spread Operator
Another way to create an Array
object from a NodeList
is by using the spread operator syntax ([...iterable]
):
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = [...divs];
The ES6's spread operator is a concise, and cool way of converting a NodeList
to an array in JavaScript. However, it only works in modern browsers.
Array.prototype.slice()
Method
Finally, the last way to convert a NodeList
to an Array
object is by using the call()
method to execute the Array.prototype.slice()
method on the NodeList
object as shown below:
// create a 'NodeList' object
const divs = document.querySelectorAll('div');
// convert 'NodeList' to an array
const divsArr = Array.prototype.slice.call(divs);
You can also use a concise form of the above method:
const divsArr = [].slice.call(divs);
The Array.prototype.slice.call()
works well in all modern and old browsers including IE 6+. You should use this approach if you want to support the most number of browsers.
All of the above methods give a real JavaScript array, which you can iterate through using forEach() and use all your favorite array methods to do different things.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur