published: 09 May 2022
2 min read
How to convert a date to UTC string in JavaScript
To convert a JavaScript date object to a UTC string:
- Use the
toUTCString()
method of theDate
object. - The
toUTCString()
method converts a date to a string using the universal time zone.
const date = new Date()
// Date in the local time zone
console.log(date.toString())
// Sun Jun 20 2021 16:36:21 GMT+0500 (Pakistan Standard Time)
// Date in UTC time zone
console.log(date.toUTCString())
// Sun, 20 Jun 2021 11:36:56 GMT
Alternatively, you could use the Date.UTC()
method to create a new Date
object directly in the UTC time zone. By default, the Date.UTC()
method returns the number of milliseconds since January 1, 1970, 00:00:00 UTC.
In the following example, we use new Date()
to convert the milliseconds into a JavaScript Date
object.
const date = new Date(Date.UTC(2021, 5, 20, 12, 44, 20))
const utc = date.toUTCString()
console.log(utc)
// Sun, 20 Jun 2021 12:44:20 GMT
If you need to have the returned UTC string in ISO-8601 format, use the toISOString()
method instead:
console.log(new Date().toISOString())
// 2021-06-20T11:40:39.937Z
The toISOString()
method returns a string in ISO-8601 format (YYYY-MM-DDTHH:mm:ss.sssZ
). The time zone is always UTC, as denoted by the suffix Z
.
Read Next: How to get current time zone in JavaScript
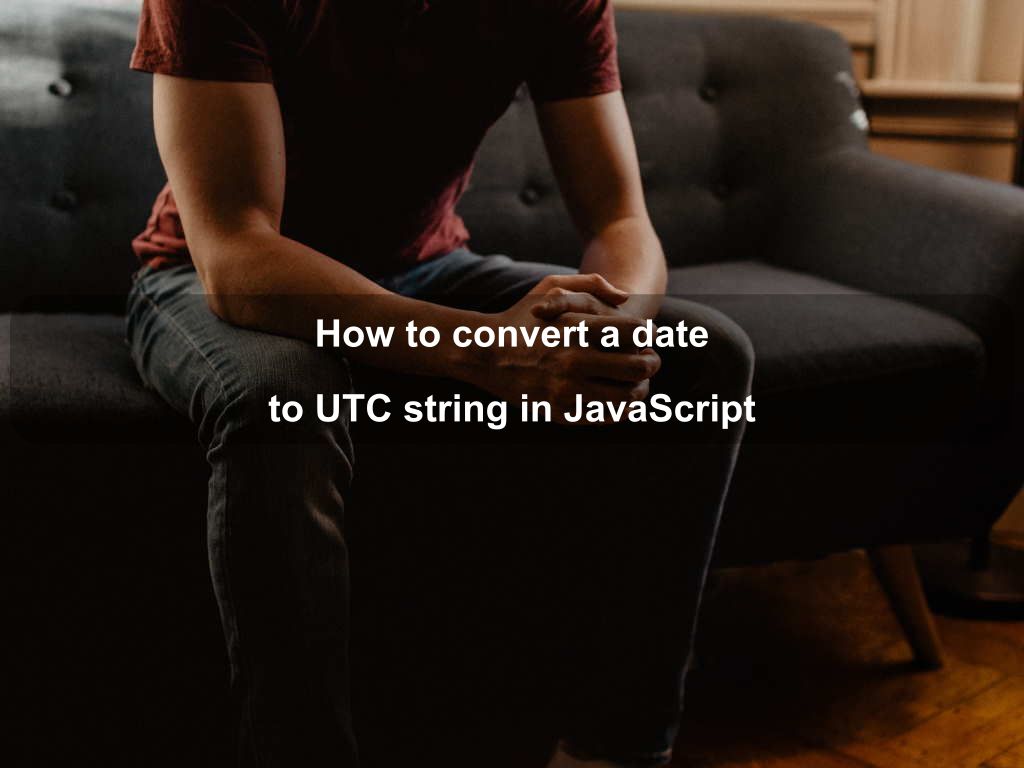
Are we missing something? Help us improve this article. Reach out to us.
How to convert a date to UTC string in JavaScript
To convert a JavaScript date object to a UTC string:
- Use the
toUTCString()
method of theDate
object. - The
toUTCString()
method converts a date to a string using the universal time zone.
const date = new Date()
// Date in the local time zone
console.log(date.toString())
// Sun Jun 20 2021 16:36:21 GMT+0500 (Pakistan Standard Time)
// Date in UTC time zone
console.log(date.toUTCString())
// Sun, 20 Jun 2021 11:36:56 GMT
Alternatively, you could use the Date.UTC()
method to create a new Date
object directly in the UTC time zone. By default, the Date.UTC()
method returns the number of milliseconds since January 1, 1970, 00:00:00 UTC.
In the following example, we use new Date()
to convert the milliseconds into a JavaScript Date
object.
const date = new Date(Date.UTC(2021, 5, 20, 12, 44, 20))
const utc = date.toUTCString()
console.log(utc)
// Sun, 20 Jun 2021 12:44:20 GMT
If you need to have the returned UTC string in ISO-8601 format, use the toISOString()
method instead:
console.log(new Date().toISOString())
// 2021-06-20T11:40:39.937Z
The toISOString()
method returns a string in ISO-8601 format (YYYY-MM-DDTHH:mm:ss.sssZ
). The time zone is always UTC, as denoted by the suffix Z
.
Read Next: How to get current time zone in JavaScript
Are you looking for other code tips?
JS Nooby
Javascript connoisseur