published: 22 May 2022
2 min read
How to check if an attribute exists using JavaScript
To check if an HTML element has a specific attribute, you can use the hasAttribute()
method. This method returns true
if the specified attribute exists, otherwise it returns false
.
Let us say you have the following HTML element:
<a href='http://example.com' title='Example' data-role='self'>Example Page</a>
The following example checks if the title
attribute of the anchor element exists:
const anchor = document.querySelector('a');
const isTitle = anchor.hasAttribute('title');
console.log(isTitle); // true
The hasAttribute()
method also works for the HTML5 data-*
attributes.
The following example demonstrates how you can use the hasAttribute()
method to check if the anchor element has the data-role
attribute:
const anchor = document.querySelector('a');
const isRole = anchor.hasAttribute('data-role');
console.log(isRole); // true
To check if an HTML element has any attributes, JavaScript provides the hasAttributes()
method:
console.log(anchor.hasAttributes()); // true
The hasAttribute()
method works in all modern browsers, and IE9 and above.
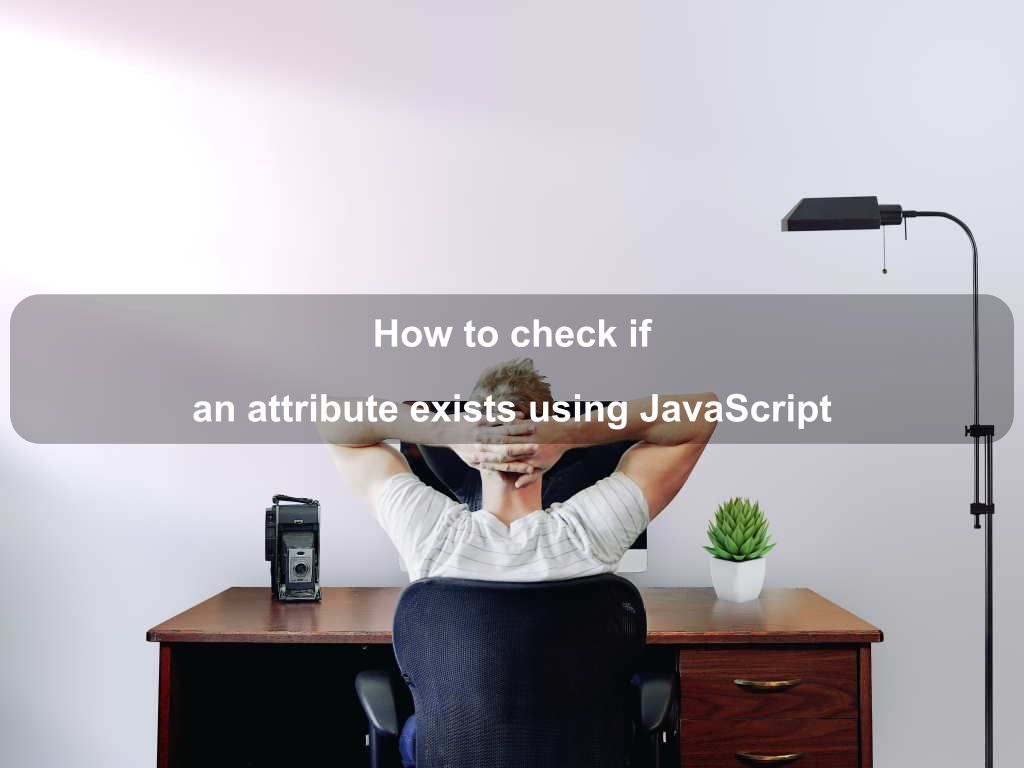
Are we missing something? Help us improve this article. Reach out to us.
How to check if an attribute exists using JavaScript
To check if an HTML element has a specific attribute, you can use the hasAttribute()
method. This method returns true
if the specified attribute exists, otherwise it returns false
.
Let us say you have the following HTML element:
<a href='http://example.com' title='Example' data-role='self'>Example Page</a>
The following example checks if the title
attribute of the anchor element exists:
const anchor = document.querySelector('a');
const isTitle = anchor.hasAttribute('title');
console.log(isTitle); // true
The hasAttribute()
method also works for the HTML5 data-*
attributes.
The following example demonstrates how you can use the hasAttribute()
method to check if the anchor element has the data-role
attribute:
const anchor = document.querySelector('a');
const isRole = anchor.hasAttribute('data-role');
console.log(isRole); // true
To check if an HTML element has any attributes, JavaScript provides the hasAttributes()
method:
console.log(anchor.hasAttributes()); // true
The hasAttribute()
method works in all modern browsers, and IE9 and above.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur