published: 24 Aug 2022
2 min read
How to check if a variable is undefined or NULL in JavaScript
To check if a variable is undefined
or null
in JavaScript, either use the equality operator ==
or strict equality operator ===
.
In JavaScript, a variable is considered undefined
if it is declared but not assigned a value. Whereas the null
is a special assignment value that signifies 'no value' or nonexistence of any value:
let name
let age = null
console.log(name) // undefined
console.log(age) // null
The equality operator ==
(also called loose equality operator) provides a more concise way to check whether the variable is undefined
or null
:
let name // undefined variable
if (name == null) {
console.log('Name is either undefined or null')
} else {
console.log('Name is NOT undefined or null')
}
// Output => Name is either undefined or null
The condition name == null
evaluates to true
because the equality operator treats both undefined
and null
as the same:
console.log(null == undefined) // true
Since the strict equality operator ===
(also called identity operator) does not treat undefined
and null
as the same, we have to use the ||
(or) operator to check if either of the two conditions is met:
let name
if (name === undefined || name === null) {
console.log('Name is either undefined or null')
} else {
console.log('Name is NOT undefined or null')
}
// Output => Name is either undefined or null
The strict equality operator ===
is more explicit but less concise as compared to the loose equality operator ==
for checking if the variable is undefined
or null
in JavaScript.
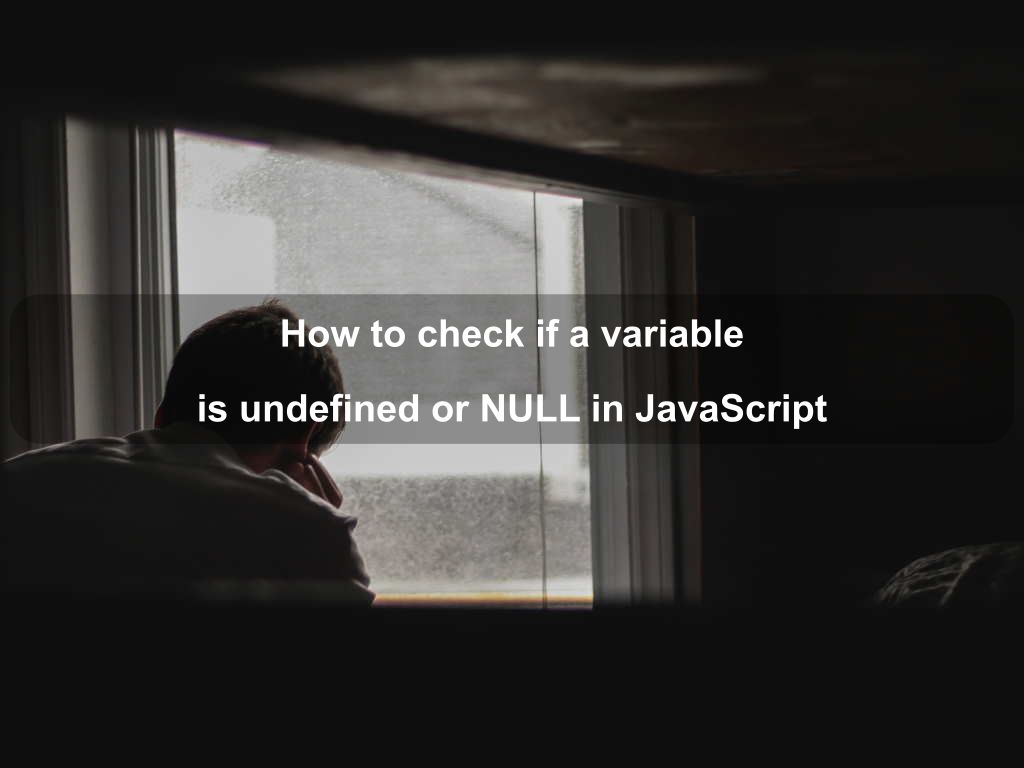
Are we missing something? Help us improve this article. Reach out to us.
How to check if a variable is undefined or NULL in JavaScript
To check if a variable is undefined
or null
in JavaScript, either use the equality operator ==
or strict equality operator ===
.
In JavaScript, a variable is considered undefined
if it is declared but not assigned a value. Whereas the null
is a special assignment value that signifies 'no value' or nonexistence of any value:
let name
let age = null
console.log(name) // undefined
console.log(age) // null
The equality operator ==
(also called loose equality operator) provides a more concise way to check whether the variable is undefined
or null
:
let name // undefined variable
if (name == null) {
console.log('Name is either undefined or null')
} else {
console.log('Name is NOT undefined or null')
}
// Output => Name is either undefined or null
The condition name == null
evaluates to true
because the equality operator treats both undefined
and null
as the same:
console.log(null == undefined) // true
Since the strict equality operator ===
(also called identity operator) does not treat undefined
and null
as the same, we have to use the ||
(or) operator to check if either of the two conditions is met:
let name
if (name === undefined || name === null) {
console.log('Name is either undefined or null')
} else {
console.log('Name is NOT undefined or null')
}
// Output => Name is either undefined or null
The strict equality operator ===
is more explicit but less concise as compared to the loose equality operator ==
for checking if the variable is undefined
or null
in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur