published: 20 Sep 2022
2 min read
How to check if a variable is an integer in JavaScript
To check if a variable is an integer in JavaScript, use the Number.isInteger()
method. It returns true
if the given value is an integer. Otherwise, false
.
Number.isInteger(34) // true
Number.isInteger(2e64) // true
Number.isInteger('0') // false
Number.isInteger(Math.PI) // false
Number.isInteger(null) // false
Number.isInteger({}) // false
Number.isInteger(Infinity) // false
Number.isInteger(NaN) // false
Number.isInteger(-Infinity) // false
The Number.isInteger()
method was introduced in ES6 to determine whether the passed value is an integer.
If the given value is an integer, it returns true
. For non-numeric values, NaN
, positive or negative Infinity
, or even for an instance of theNumber
class, it returns false
.
The Number.isInteger()
method will also return true
for floating point numbers that can be represented as an integer:
Number.isInteger(2.0) // true
Number.isInteger(2.3) // false
Number.isInteger(new Number(2)) // false
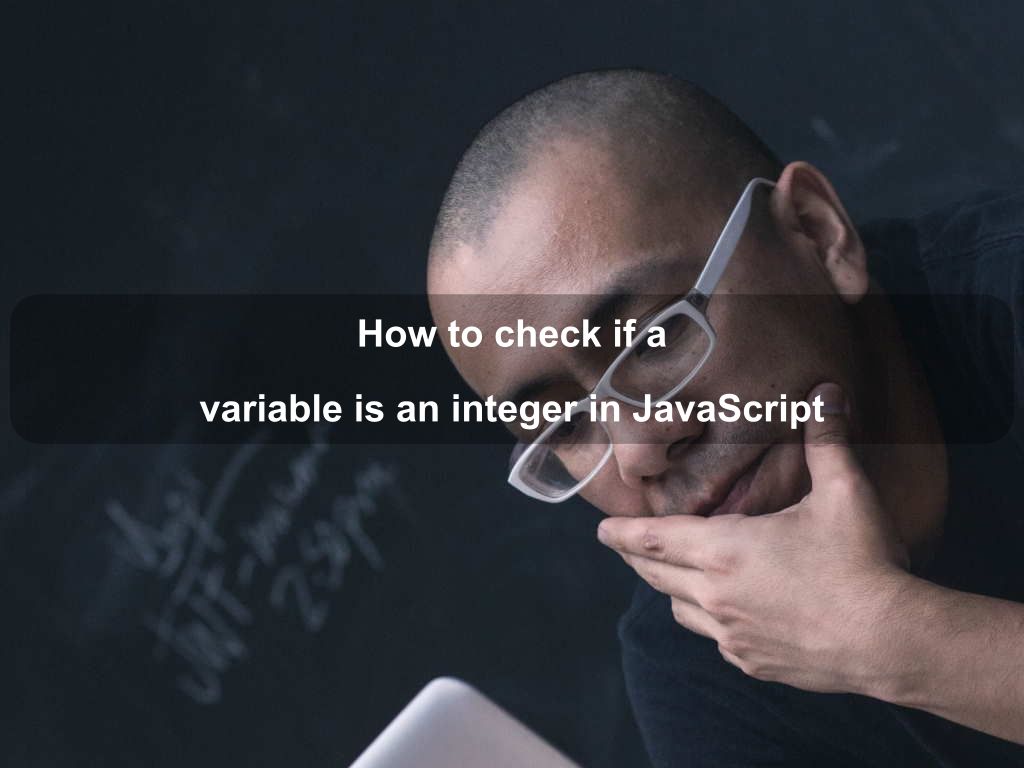
Are we missing something? Help us improve this article. Reach out to us.
How to check if a variable is an integer in JavaScript
To check if a variable is an integer in JavaScript, use the Number.isInteger()
method. It returns true
if the given value is an integer. Otherwise, false
.
Number.isInteger(34) // true
Number.isInteger(2e64) // true
Number.isInteger('0') // false
Number.isInteger(Math.PI) // false
Number.isInteger(null) // false
Number.isInteger({}) // false
Number.isInteger(Infinity) // false
Number.isInteger(NaN) // false
Number.isInteger(-Infinity) // false
The Number.isInteger()
method was introduced in ES6 to determine whether the passed value is an integer.
If the given value is an integer, it returns true
. For non-numeric values, NaN
, positive or negative Infinity
, or even for an instance of theNumber
class, it returns false
.
The Number.isInteger()
method will also return true
for floating point numbers that can be represented as an integer:
Number.isInteger(2.0) // true
Number.isInteger(2.3) // false
Number.isInteger(new Number(2)) // false
Are you looking for other code tips?
JS Nooby
Javascript connoisseur