published: 05 May 2022
2 min read
How to check if a variable is a string in JavaScript
To check if a variable is a string in JavaScript, use the typeof
operator, e.g. typeof a === 'string'
. If the typeof
operator returns 'string'
, then the variable is a string. For all other values, the variable is not a string.
let name = 'Atta'
if (typeof name === 'string') {
console.log('Variable is a string')
} else {
console.log('Variable is NOT a string')
}
// Output => Variable is a string
In the above example, we used the typeof
operator to check if a variable is a string.
The typeof
operator returns a string indicating the type of the given value. Here are a few more examples:
console.log(typeof 'john') // 'string'
console.log(typeof '4') // 'string'
console.log(typeof true) // 'boolean'
console.log(typeof []) // 'object'
console.log(typeof {}) // 'object'
console.log(typeof 5) // 'number'
console.log(typeof null) // 'object'
console.log(typeof (() => {})) // 'function'
Unlike the other operators, the typeof
operator does not throw a ReferenceError
exception when used with a variable that is not declared:
if (typeof price === 'string') {
console.log('Variable is a string')
} else {
console.log('Variable is NOT a string')
}
// Output => Variable is NOT a string
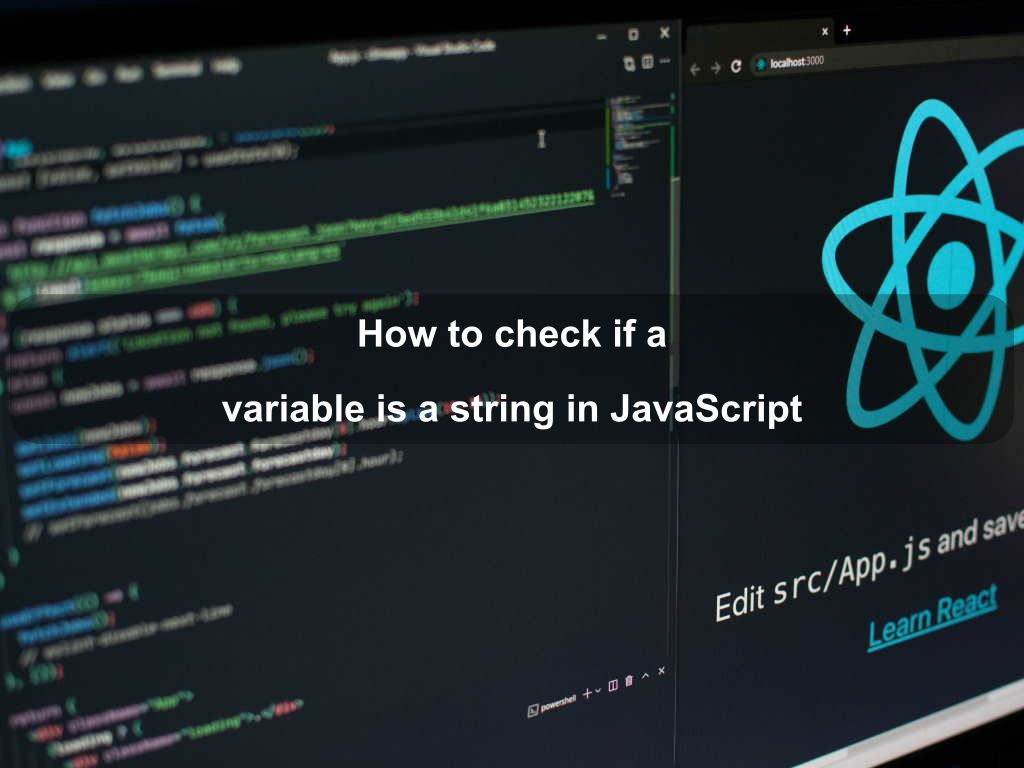
Are we missing something? Help us improve this article. Reach out to us.
How to check if a variable is a string in JavaScript
To check if a variable is a string in JavaScript, use the typeof
operator, e.g. typeof a === 'string'
. If the typeof
operator returns 'string'
, then the variable is a string. For all other values, the variable is not a string.
let name = 'Atta'
if (typeof name === 'string') {
console.log('Variable is a string')
} else {
console.log('Variable is NOT a string')
}
// Output => Variable is a string
In the above example, we used the typeof
operator to check if a variable is a string.
The typeof
operator returns a string indicating the type of the given value. Here are a few more examples:
console.log(typeof 'john') // 'string'
console.log(typeof '4') // 'string'
console.log(typeof true) // 'boolean'
console.log(typeof []) // 'object'
console.log(typeof {}) // 'object'
console.log(typeof 5) // 'number'
console.log(typeof null) // 'object'
console.log(typeof (() => {})) // 'function'
Unlike the other operators, the typeof
operator does not throw a ReferenceError
exception when used with a variable that is not declared:
if (typeof price === 'string') {
console.log('Variable is a string')
} else {
console.log('Variable is NOT a string')
}
// Output => Variable is NOT a string
Are you looking for other code tips?
JS Nooby
Javascript connoisseur