published: 23 May 2022
2 min read
How to check if a variable is a number in JavaScript
To check if a variable is a number in JavaScript:
- Use the
Number.isFinite()
method to determine whether the passed value is a number. - The
Number.isFinite()
method returnstrue
if the passed value is not positiveInfinity
, negativeInfinity
, orNaN
.
Number.isFinite(34) // true
Number.isFinite(2e64) // true
Number.isFinite('0') // false
Number.isFinite(null) // false
Number.isFinite({}) // false
Number.isFinite(Infinity) // false
Number.isFinite(NaN) // false
Number.isFinite(-Infinity) // false
The Number.isFinite()
method was introduced in ES6 to check if the given value is a finite number. It returns true
if the passed value is a number, otherwise false
.
JavaScript also provides a global isFinite()
function that converts the parameter to a Number
and returns true
if the resulting value is not positive or negative Infinity
, NaN
, or undefined
.
This means, with Number.isFinite()
, only values of the type number and are finite return true
, and non-numbers always return false
:
isFinite('2') // true
Number.isFinite('2') // false
isFinite(null) // true
Number.isFinite(null) // false
Alternatively, you can use the Number.isNaN()
method to check if the passed value is a Number
and NaN
(Not a Number). This method is a more robust replacement of the global isNaN()
function in JavaScript.
Number.isNaN(34) // false <- 34 is a valid number
Number.isNaN(2e64) // false
Number.isNaN('0') // false <- 0 is a valid number
Number.isNaN(null) // false (false positive?)
Number.isNaN({}) // false
Number.isNaN(Infinity) // false
Number.isNaN(NaN) // true <- NaN is 'Not a Number'
Number.isNaN(-Infinity) // false
Unlike the global isNaN()
function, Number.isNaN()
does not convert the argument to a Number
; it returns true
when the argument is a Number
and is NaN
.
Finally, the typeof
operator can also be used to check if the variable type is a number
in JavaScript:
typeof 3 === 'number' // true
typeof '0' === 'number' // false ('string' type)
typeof null === 'number' // false
typeof {} === 'number' // false
typeof undefined === 'number' // false
typeof NaN === 'number' // true (false positive?)
typeof Infinity === 'number' // true
The typeof
operator is more robust than Number.isNaN()
and it correctly identifies null
and undefined
are not numbers.
However, it returns true
for Infinity
and NaN
because these are special number values in JavaScript.
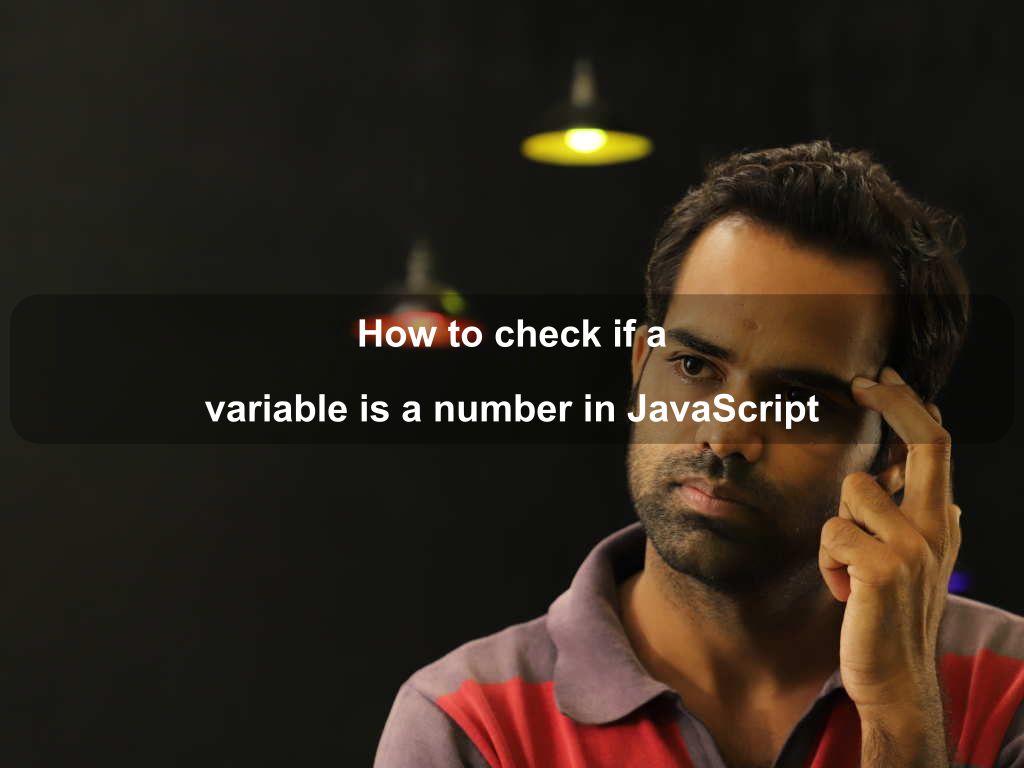
Are we missing something? Help us improve this article. Reach out to us.
How to check if a variable is a number in JavaScript
To check if a variable is a number in JavaScript:
- Use the
Number.isFinite()
method to determine whether the passed value is a number. - The
Number.isFinite()
method returnstrue
if the passed value is not positiveInfinity
, negativeInfinity
, orNaN
.
Number.isFinite(34) // true
Number.isFinite(2e64) // true
Number.isFinite('0') // false
Number.isFinite(null) // false
Number.isFinite({}) // false
Number.isFinite(Infinity) // false
Number.isFinite(NaN) // false
Number.isFinite(-Infinity) // false
The Number.isFinite()
method was introduced in ES6 to check if the given value is a finite number. It returns true
if the passed value is a number, otherwise false
.
JavaScript also provides a global isFinite()
function that converts the parameter to a Number
and returns true
if the resulting value is not positive or negative Infinity
, NaN
, or undefined
.
This means, with Number.isFinite()
, only values of the type number and are finite return true
, and non-numbers always return false
:
isFinite('2') // true
Number.isFinite('2') // false
isFinite(null) // true
Number.isFinite(null) // false
Alternatively, you can use the Number.isNaN()
method to check if the passed value is a Number
and NaN
(Not a Number). This method is a more robust replacement of the global isNaN()
function in JavaScript.
Number.isNaN(34) // false <- 34 is a valid number
Number.isNaN(2e64) // false
Number.isNaN('0') // false <- 0 is a valid number
Number.isNaN(null) // false (false positive?)
Number.isNaN({}) // false
Number.isNaN(Infinity) // false
Number.isNaN(NaN) // true <- NaN is 'Not a Number'
Number.isNaN(-Infinity) // false
Unlike the global isNaN()
function, Number.isNaN()
does not convert the argument to a Number
; it returns true
when the argument is a Number
and is NaN
.
Finally, the typeof
operator can also be used to check if the variable type is a number
in JavaScript:
typeof 3 === 'number' // true
typeof '0' === 'number' // false ('string' type)
typeof null === 'number' // false
typeof {} === 'number' // false
typeof undefined === 'number' // false
typeof NaN === 'number' // true (false positive?)
typeof Infinity === 'number' // true
The typeof
operator is more robust than Number.isNaN()
and it correctly identifies null
and undefined
are not numbers.
However, it returns true
for Infinity
and NaN
because these are special number values in JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur