published: 24 Sep 2022
2 min read
How to check if a date is today in JavaScript
From a JavaScript date instance, we can get the day, month and year values by using getDate()
, getMonth()
and getFullYear()
methods:
// month is zero-based (0-11)
const date = new Date(2022, 7, 7)
date.getDate() // 7
date.getMonth() // 7
date.getFullYear() // 2022
Now let's create a small function that takes a date as an argument and compares the above values to today's date values, and returns true if both are the same:
const isToday = date => {
const today = new Date()
return date.getDate() === today.getDate() &&
date.getMonth() === today.getMonth() &&
date.getFullYear() === today.getFullYear()
}
Here is how you can use it:
const date = new Date(2022, 7, 7)
console.log(isToday(date)) // true
Alternatively, you can extend the date object by adding the above function directly to object prototype like below:
Date.prototype.isToday = function () {
const today = new Date()
return this.getDate() === today.getDate() &&
this.getMonth() === today.getMonth() &&
this.getFullYear() === today.getFullYear()
}
Now just call the isToday()
method on any date object to compare it with today's date:
const date = new Date(2022, 7, 7)
console.log(date.isToday())
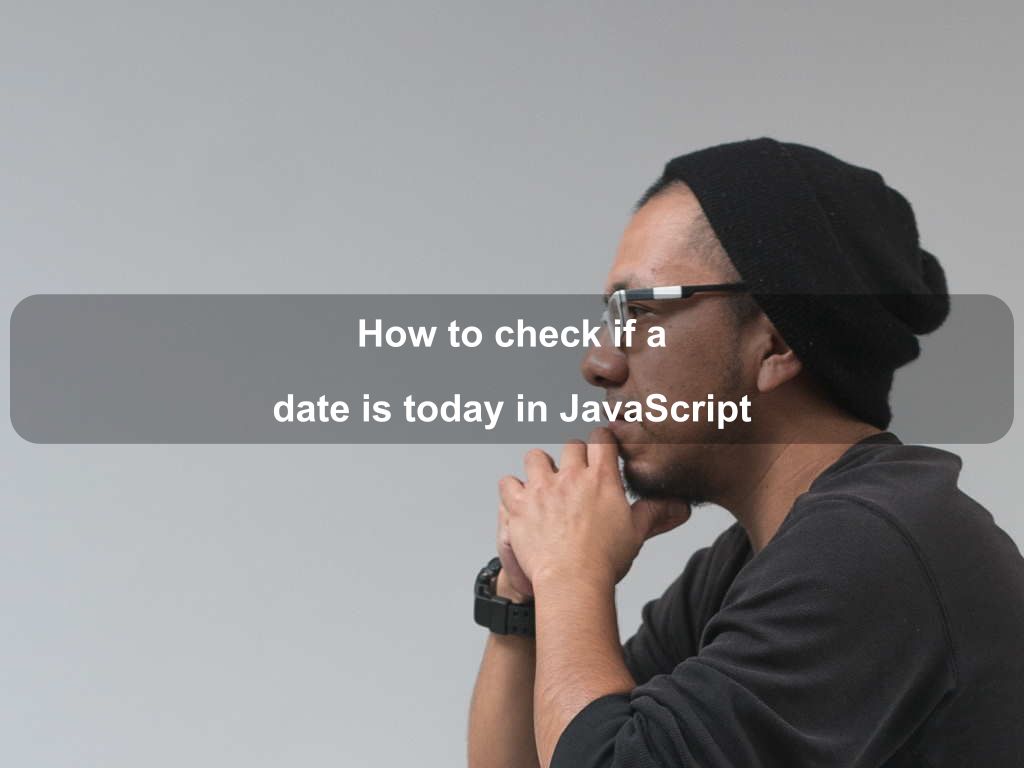
Are we missing something? Help us improve this article. Reach out to us.
How to check if a date is today in JavaScript
From a JavaScript date instance, we can get the day, month and year values by using getDate()
, getMonth()
and getFullYear()
methods:
// month is zero-based (0-11)
const date = new Date(2022, 7, 7)
date.getDate() // 7
date.getMonth() // 7
date.getFullYear() // 2022
Now let's create a small function that takes a date as an argument and compares the above values to today's date values, and returns true if both are the same:
const isToday = date => {
const today = new Date()
return date.getDate() === today.getDate() &&
date.getMonth() === today.getMonth() &&
date.getFullYear() === today.getFullYear()
}
Here is how you can use it:
const date = new Date(2022, 7, 7)
console.log(isToday(date)) // true
Alternatively, you can extend the date object by adding the above function directly to object prototype like below:
Date.prototype.isToday = function () {
const today = new Date()
return this.getDate() === today.getDate() &&
this.getMonth() === today.getMonth() &&
this.getFullYear() === today.getFullYear()
}
Now just call the isToday()
method on any date object to compare it with today's date:
const date = new Date(2022, 7, 7)
console.log(date.isToday())
Are you looking for other code tips?
JS Nooby
Javascript connoisseur