published: 14 May 2022
2 min read
How to catch exceptions in setTimeout and setInterval
In JavaScript, we use the try...catch
statement to capture unexpected errors and exceptions.
How does try...catch
work?
try {
// block of code
} catch (error) {
// handle the error
} finally {
// execute in all cases
}
- The
try
clause is executed first. - If no exception is thrown, the
catch
clause is ignored, and the execution of thetry
statement is completed. - If an exception is thrown during the execution of the
try
clause, the rest of this clause is ignored. Thecatch
clause is executed, and what comes after thetry
statement is executed. - The
finally
clause is optional and executes after both clauses, regardless of whether an exception is thrown or not.
Capturing exceptions in setTimeout
and setInterval
Both setTimeout
and setInterval
functions call or evaluate an expression after a specified number of milliseconds. If we put these methods inside the try
clause and an exception is thrown, the catch
clause will not catch any of them:
try {
setTimeout(() => {
throw new Error('An exception is thrown')
}, 500)
} catch (error) {
console.error({ error })
}
This is because the try...catch
statement works synchronously, and the function in question is executed asynchronously after a certain period of time.
To resolve this issue, we have to put the try...catch
block inside the function:
setTimeout(() => {
try {
throw new Error('An exception is thrown')
} catch (error) {
console.error({ error })
}
}, 500)
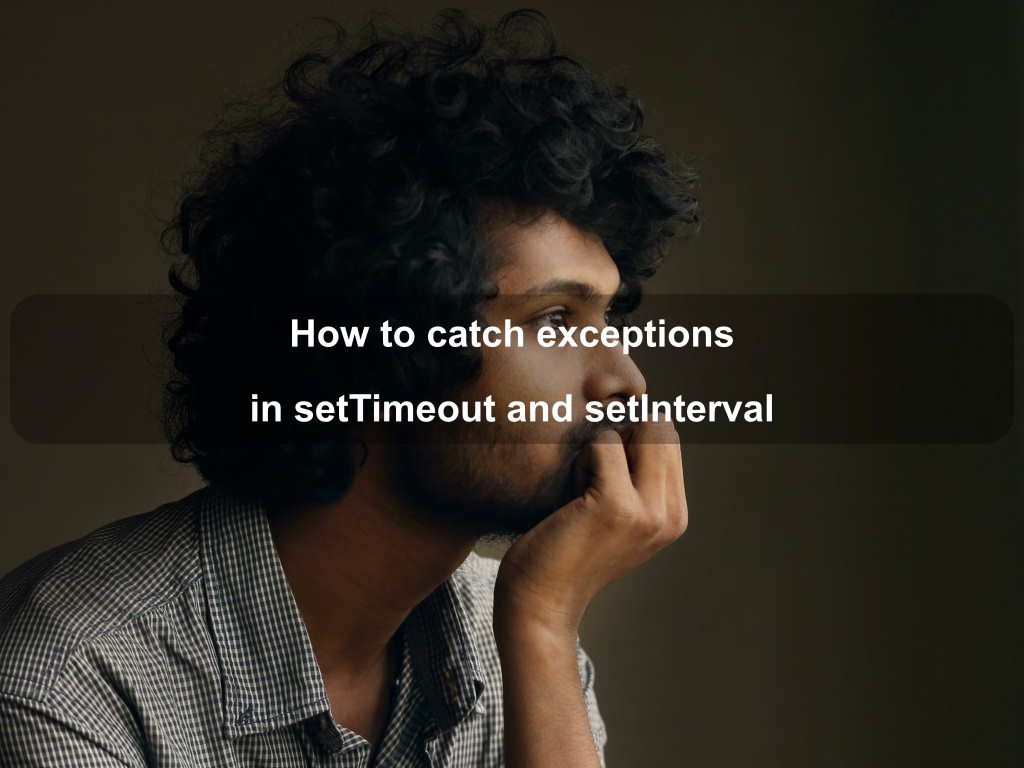
Are we missing something? Help us improve this article. Reach out to us.
How to catch exceptions in setTimeout and setInterval
In JavaScript, we use the try...catch
statement to capture unexpected errors and exceptions.
How does try...catch
work?
try {
// block of code
} catch (error) {
// handle the error
} finally {
// execute in all cases
}
- The
try
clause is executed first. - If no exception is thrown, the
catch
clause is ignored, and the execution of thetry
statement is completed. - If an exception is thrown during the execution of the
try
clause, the rest of this clause is ignored. Thecatch
clause is executed, and what comes after thetry
statement is executed. - The
finally
clause is optional and executes after both clauses, regardless of whether an exception is thrown or not.
Capturing exceptions in setTimeout
and setInterval
Both setTimeout
and setInterval
functions call or evaluate an expression after a specified number of milliseconds. If we put these methods inside the try
clause and an exception is thrown, the catch
clause will not catch any of them:
try {
setTimeout(() => {
throw new Error('An exception is thrown')
}, 500)
} catch (error) {
console.error({ error })
}
This is because the try...catch
statement works synchronously, and the function in question is executed asynchronously after a certain period of time.
To resolve this issue, we have to put the try...catch
block inside the function:
setTimeout(() => {
try {
throw new Error('An exception is thrown')
} catch (error) {
console.error({ error })
}
}, 500)
Are you looking for other code tips?
JS Nooby
Javascript connoisseur