published: 04 Nov 2022
5 min read
How to Append one Array to Another in JavaScript.
The code is self-explanatory
1. Using the spread operator
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; const arr3 = [...arr1, ...arr2]; console.log(arr3); // 👉️ ['a', 'b', 'c', 'd']
2. Using the array concat
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; const arr3 = arr1.concat(arr2); console.log(arr3); // 👉️ ['a', 'b', 'c', 'd']
3. Using the array push
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; arr1.push(...arr2); console.log(arr1); // 👉️ ['a', 'b', 'c', 'd']
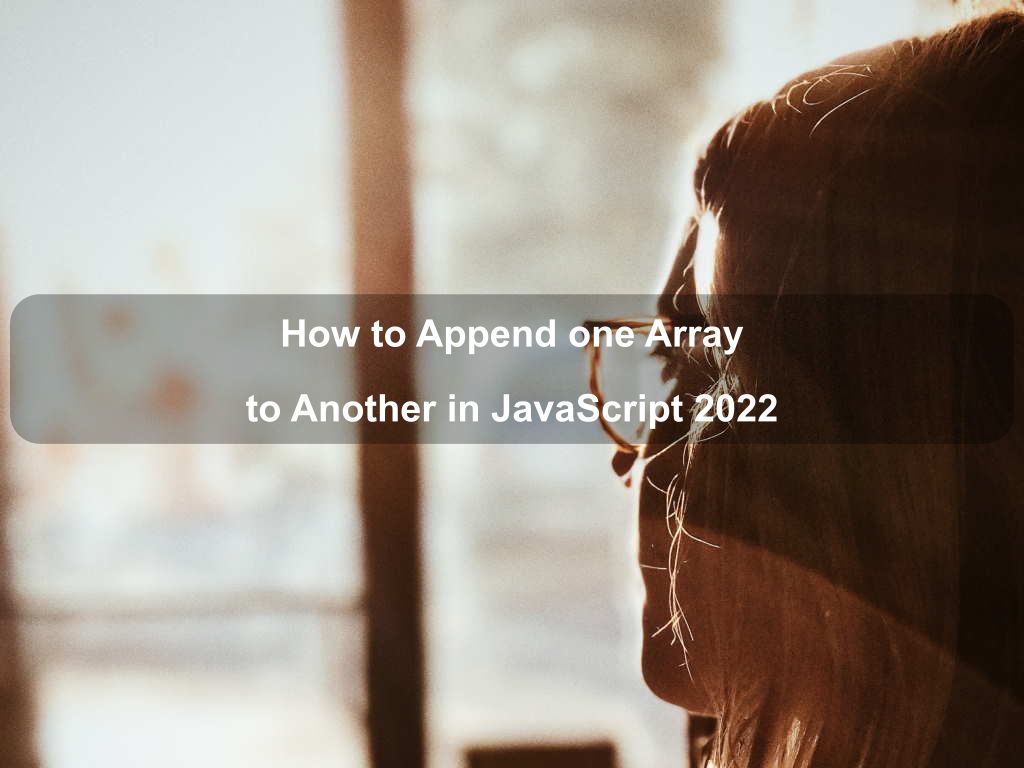
Are we missing something? Help us improve this article. Reach out to us.
How to Append one Array to Another in JavaScript.
The code is self-explanatory
1. Using the spread operator
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; const arr3 = [...arr1, ...arr2]; console.log(arr3); // 👉️ ['a', 'b', 'c', 'd']
2. Using the array concat
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; const arr3 = arr1.concat(arr2); console.log(arr3); // 👉️ ['a', 'b', 'c', 'd']
3. Using the array push
const arr1 = ['a', 'b']; const arr2 = ['c', 'd']; arr1.push(...arr2); console.log(arr1); // 👉️ ['a', 'b', 'c', 'd']
Are you looking for other code tips?
Check out what's on in the category: javascript, programming
Check out what's on in the tag: array, javascript
TipsAndTricksta
I love coding, I love coding tips & tricks, code snippets and javascript