published: 09 Aug 2022
2 min read
How to add days to a date in JavaScript
To add days to a date in JavaScript:
- Use the
getDate()
method to get the day of the month for the given date. - Use the
setDate()
method, passing it the result of callinggetDate()
plus the number of days you want to add. - The
setDate()
method will add the specified number of days to theDate
object.
For example, to add one day to the current date, use the following code:
const today = new Date();
const tomorrow = new Date()
// Add 1 Day
tomorrow.setDate(today.getDate() + 1)
To update an existing JavaScript Date
object, you can do the following:
const date = new Date();
date.setDate(date.getDate() + 1)
console.log(date)
To add seven days to a Date
object, use the following code:
const date = new Date();
// Add 7 Days
date.setDate(date.getDate() + 7)
console.log(date)
You could even add a new method to the Date
's prototype and call it directly whenever you want to manipulate days like below:
Date.prototype.addDays = function (days) {
const date = new Date(this.valueOf())
date.setDate(date.getDate() + days)
return date
}
// Add 7 Days
const date = new Date('2020-12-02')
console.log(date.addDays(7))
// 2020-12-09T00:00:00.000Z
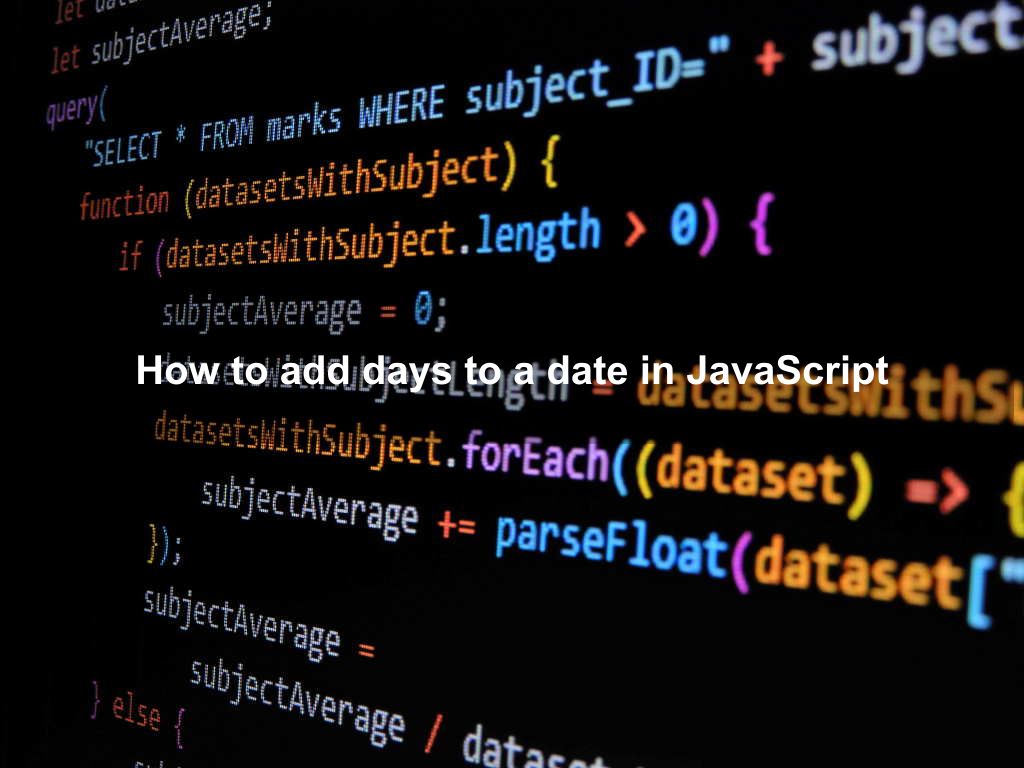
Are we missing something? Help us improve this article. Reach out to us.
How to add days to a date in JavaScript
To add days to a date in JavaScript:
- Use the
getDate()
method to get the day of the month for the given date. - Use the
setDate()
method, passing it the result of callinggetDate()
plus the number of days you want to add. - The
setDate()
method will add the specified number of days to theDate
object.
For example, to add one day to the current date, use the following code:
const today = new Date();
const tomorrow = new Date()
// Add 1 Day
tomorrow.setDate(today.getDate() + 1)
To update an existing JavaScript Date
object, you can do the following:
const date = new Date();
date.setDate(date.getDate() + 1)
console.log(date)
To add seven days to a Date
object, use the following code:
const date = new Date();
// Add 7 Days
date.setDate(date.getDate() + 7)
console.log(date)
You could even add a new method to the Date
's prototype and call it directly whenever you want to manipulate days like below:
Date.prototype.addDays = function (days) {
const date = new Date(this.valueOf())
date.setDate(date.getDate() + days)
return date
}
// Add 7 Days
const date = new Date('2020-12-02')
console.log(date.addDays(7))
// 2020-12-09T00:00:00.000Z
Are you looking for other code tips?
JS Nooby
Javascript connoisseur