published: 10 May 2022
2 min read
Hide and show DOM elements using a CSS class in JavaScript
A few days ago, I wrote an article on how to hide and show DOM elements using inline styles in JavaScript. Today, you'll learn to do hide and show elements using CSS classes in JavaScript.
Let us first declare a simple CSS class that hides the element, if applied, by setting display: none
:
.hidden {
display: none;
}
Next, say we have the following <button>
element:
<button>Click Me</button>
Now if you want to hide the above button from the DOM, just add the hidden
class by using the add()
method provided by the classList object, like below:
// grab button element
const button = document.querySelector('.btn');
// hide it
button.classList.add('hidden');
To show the element again, simply removes the hidden
class by using the classList.remove()
method:
button.classList.remove('hidden');
While inline styles work perfectly to toggle the element visibility, the CSS class gives you more flexibility to control the behavior like this.
Creating hide()
& Show()
Methods
The classList
object provides a bunch of methods to add, remove, and toggle CSS classes from an element in vanilla JavaScript.
Let us use classList
to create our own jQuery-like hide()
, show()
, and toggle()
methods in pure JavaScript:
// hide an element
const hide = (elem) => {
elem.classList.add('hidden');
}
// show an element
const show = (elem) => {
elem.classList.remove('hidden');
}
// toggle the element visibility
const toggle = (elem) => {
elem.classList.toggle('hidden');
}
Now, if you want to hide or show any DOM element, just call the above method:
// hide
hide(document.querySelector('.btn'));
// show
show(document.querySelector('.btn'));
// toggle
toggle(document.querySelector('.btn'));
Take a look at this guide to learn more about handling CSS classes in vanilla JavaScript.
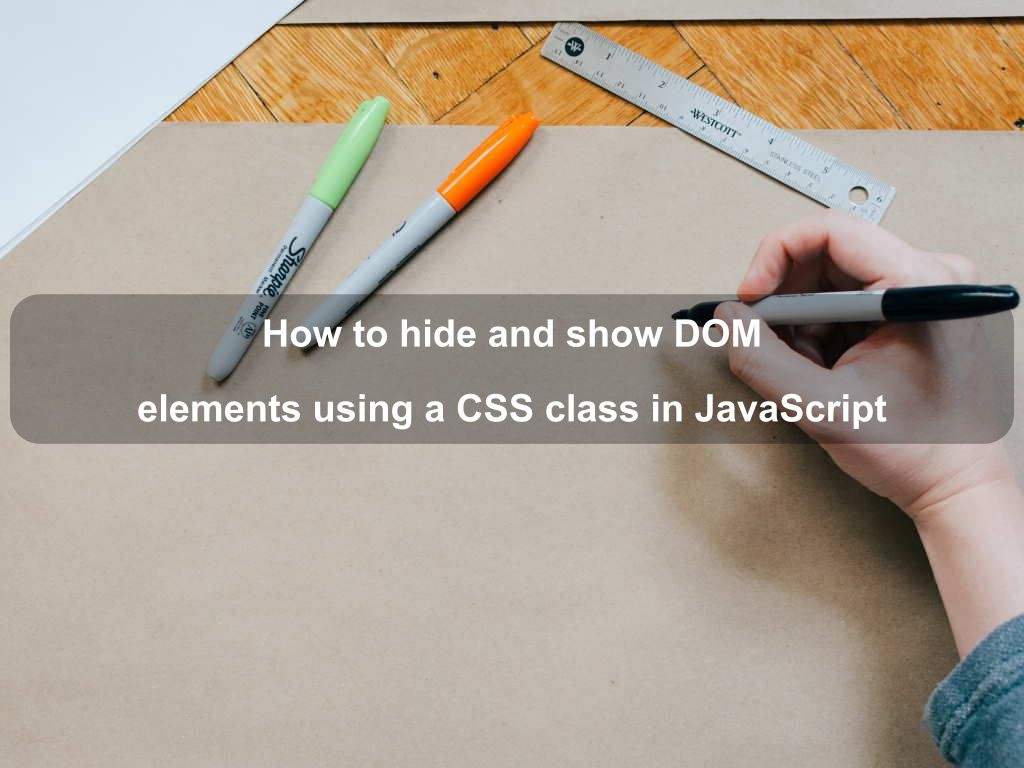
Are we missing something? Help us improve this article. Reach out to us.
Hide and show DOM elements using a CSS class in JavaScript
A few days ago, I wrote an article on how to hide and show DOM elements using inline styles in JavaScript. Today, you'll learn to do hide and show elements using CSS classes in JavaScript.
Let us first declare a simple CSS class that hides the element, if applied, by setting display: none
:
.hidden {
display: none;
}
Next, say we have the following <button>
element:
<button>Click Me</button>
Now if you want to hide the above button from the DOM, just add the hidden
class by using the add()
method provided by the classList object, like below:
// grab button element
const button = document.querySelector('.btn');
// hide it
button.classList.add('hidden');
To show the element again, simply removes the hidden
class by using the classList.remove()
method:
button.classList.remove('hidden');
While inline styles work perfectly to toggle the element visibility, the CSS class gives you more flexibility to control the behavior like this.
Creating hide()
& Show()
Methods
The classList
object provides a bunch of methods to add, remove, and toggle CSS classes from an element in vanilla JavaScript.
Let us use classList
to create our own jQuery-like hide()
, show()
, and toggle()
methods in pure JavaScript:
// hide an element
const hide = (elem) => {
elem.classList.add('hidden');
}
// show an element
const show = (elem) => {
elem.classList.remove('hidden');
}
// toggle the element visibility
const toggle = (elem) => {
elem.classList.toggle('hidden');
}
Now, if you want to hide or show any DOM element, just call the above method:
// hide
hide(document.querySelector('.btn'));
// show
show(document.querySelector('.btn'));
// toggle
toggle(document.querySelector('.btn'));
Take a look at this guide to learn more about handling CSS classes in vanilla JavaScript.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur