published: 30 Jul 2022
2 min read
Difference between a NodeList and an Array in JavaScript
In an earlier article, we looked at the ES6's forEach()
method to iterate through the elements of a NodeList as well as arrays in JavaScript. In this quick guide, I'll talk about the difference between a NodeList
and an array, to understand how they work under the hood.
A NodeList
may look like an array, but in reality, they both are two completely different things. A NodeList
object is basically a collection of DOM nodes extracted from the HTML document. An array is a special data-type in JavaScript, that can store a collection of arbitrary elements.
To create a NodeList
object, you can use the querySelectorAll
method. The following example selects all <p>
elements in the document:
const paragraphs = document.querySelectorAll('p');
A JavaScript array can be created by either using the array literal syntax or the Array
constructor:
const foods = ['Pizza', 'Pasta', 'Burger', 'Cake'];
// OR
const foods = new Array('Pizza', 'Pasta', 'Burger', 'Cake');
Both arrays and NodeLists provide the length
property to get the total number of items stored:
console.log('NodeList items: ${paragraphs.length}');
console.log('Array items: ${foods.length}');
The elements of both NodeLists and arrays can be accessed by a numeric index:
const p = paragraphs[2]; // 3rd node
const f = foods[1]; // 2nd food name
Although you can access the NodeList
elements like an array and use the same length
property, there are still certain differences. You can not use the common array methods like push()
, pop()
, slice()
, and join()
directly on a NodeList
. You have to first convert a NodeList
to a normal array using the Array.from()
method.
Another difference between a NodeList
and an array is that a NodeList
can be a live collection. This means if any element is added or removed from the DOM, the changes are automatically applied to the collection.
Both querySelector()
and querySelectorAll()
methods return a static list, which doesn't update if you make any changes to the DOM. However, the properties like Node.childNodes
are live lists, which will update as soon as you modify the DOM.
Finally, the last thing that differentiates a NodeList
from an array is the fact that the querySelectorAll()
is not a JavaScript API, but a browser API. This is kinda confusing as hell because we are using these APIs in JavaScript to manipulate the DOM. It turns out, according to MDN, other languages can also access these APIs to interact with the DOM.
Here is a Python DOM manipulation example (copied from MDN):
# Python DOM example
import xml.dom.minidom as m
doc = m.parse(r'C:\Projects\Py\chap1.xml')
doc.nodeName # DOM property of document object
p_list = doc.getElementsByTagName('para')
The biggest takeaway from the NodeList vs. an array discussion: a NodeList
is a collection of nodes that can be used to access and manipulate DOM elements, while an array is a JavaScript object which can hold more than one value at a time.
Both NodeLists and arrays have their own prototypes, methods, and properties. You can easily convert a NodeList
to an array if you want to, but not the other way around.
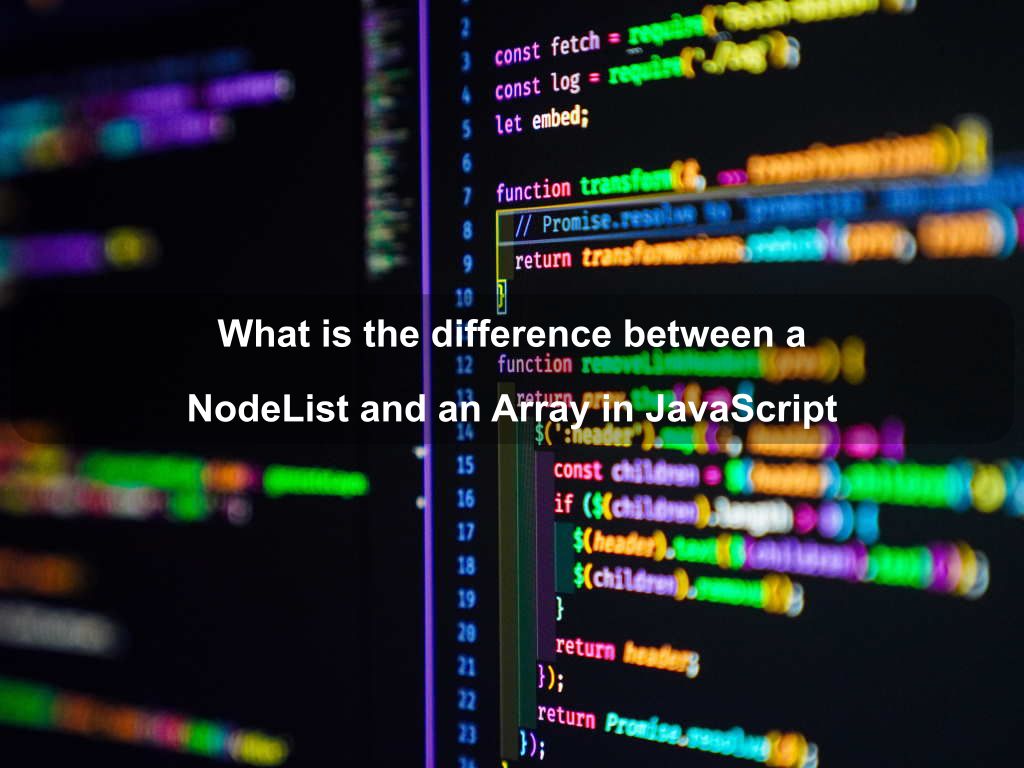
Are we missing something? Help us improve this article. Reach out to us.
Difference between a NodeList and an Array in JavaScript
In an earlier article, we looked at the ES6's forEach()
method to iterate through the elements of a NodeList as well as arrays in JavaScript. In this quick guide, I'll talk about the difference between a NodeList
and an array, to understand how they work under the hood.
A NodeList
may look like an array, but in reality, they both are two completely different things. A NodeList
object is basically a collection of DOM nodes extracted from the HTML document. An array is a special data-type in JavaScript, that can store a collection of arbitrary elements.
To create a NodeList
object, you can use the querySelectorAll
method. The following example selects all <p>
elements in the document:
const paragraphs = document.querySelectorAll('p');
A JavaScript array can be created by either using the array literal syntax or the Array
constructor:
const foods = ['Pizza', 'Pasta', 'Burger', 'Cake'];
// OR
const foods = new Array('Pizza', 'Pasta', 'Burger', 'Cake');
Both arrays and NodeLists provide the length
property to get the total number of items stored:
console.log('NodeList items: ${paragraphs.length}');
console.log('Array items: ${foods.length}');
The elements of both NodeLists and arrays can be accessed by a numeric index:
const p = paragraphs[2]; // 3rd node
const f = foods[1]; // 2nd food name
Although you can access the NodeList
elements like an array and use the same length
property, there are still certain differences. You can not use the common array methods like push()
, pop()
, slice()
, and join()
directly on a NodeList
. You have to first convert a NodeList
to a normal array using the Array.from()
method.
Another difference between a NodeList
and an array is that a NodeList
can be a live collection. This means if any element is added or removed from the DOM, the changes are automatically applied to the collection.
Both querySelector()
and querySelectorAll()
methods return a static list, which doesn't update if you make any changes to the DOM. However, the properties like Node.childNodes
are live lists, which will update as soon as you modify the DOM.
Finally, the last thing that differentiates a NodeList
from an array is the fact that the querySelectorAll()
is not a JavaScript API, but a browser API. This is kinda confusing as hell because we are using these APIs in JavaScript to manipulate the DOM. It turns out, according to MDN, other languages can also access these APIs to interact with the DOM.
Here is a Python DOM manipulation example (copied from MDN):
# Python DOM example
import xml.dom.minidom as m
doc = m.parse(r'C:\Projects\Py\chap1.xml')
doc.nodeName # DOM property of document object
p_list = doc.getElementsByTagName('para')
The biggest takeaway from the NodeList vs. an array discussion: a NodeList
is a collection of nodes that can be used to access and manipulate DOM elements, while an array is a JavaScript object which can hold more than one value at a time.
Both NodeLists and arrays have their own prototypes, methods, and properties. You can easily convert a NodeList
to an array if you want to, but not the other way around.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur