published: 03 Oct 2022
2 min read
Converting strings to uppercase and lowercase with JavaScript
In an earlier article, we looked at different ways to capitalize the first letter of a string to uppercase in JavaScript. In this article, you'll learn how to uppercase or lowercase a string completely in JavaScript.
JavaScript provides two built-in functions for converting strings to uppercase and lowercase. Let us look at them.
toUpperCase()
Method
The toUpperCase()
method transforms a string to uppercase letters in JavaScript. It doesn't change the original string and returns a new string equivalent to the string converted to uppercase.
Here is an example:
const str = 'JavaScript World!';
// convert string to uppercase
const upperStr = str.toUpperCase();
// print the new string
console.log(upperStr);
// output: JAVASCRIPT WORLD!
toLowerCase()
Method
The toLowerCase()
method does the opposite of the toUpperCase()
method. It converts all characters of the string to lowercase:
const str = 'JavaScript World!';
// convert string to lowercase
const lowerStr = str.toLowerCase();
// print the new string
console.log(lowerStr);
// output: javascript world!
Both these methods work in all modern browsers and are supported back to at least Internet Explorer 6.
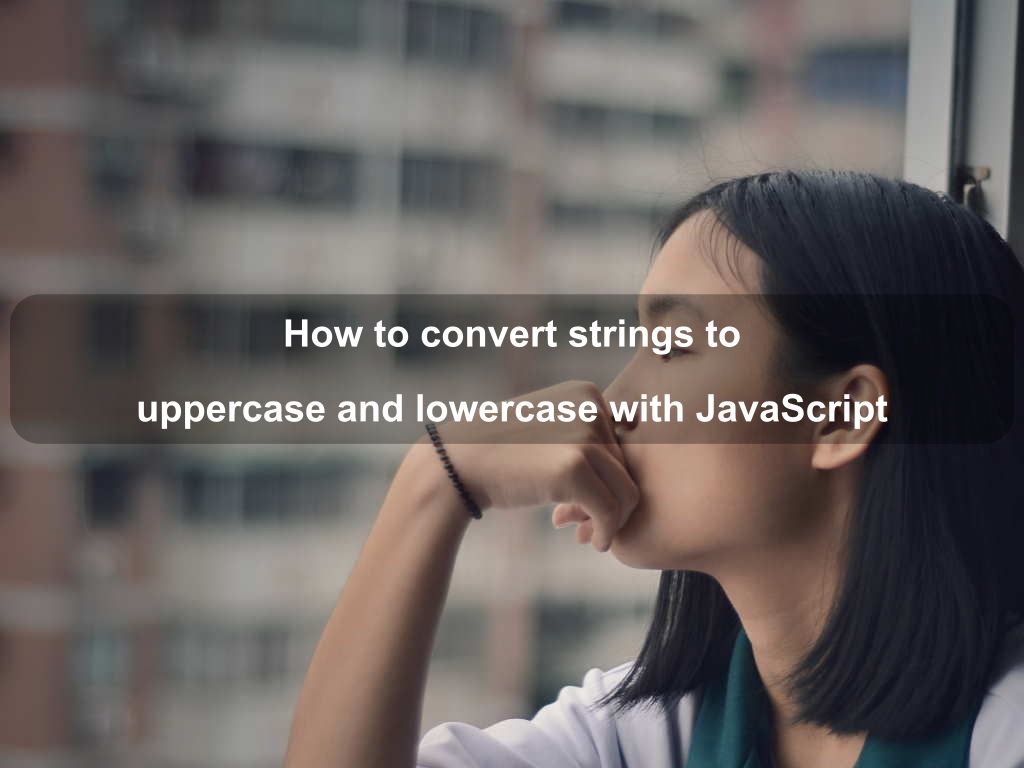
Are we missing something? Help us improve this article. Reach out to us.
Converting strings to uppercase and lowercase with JavaScript
In an earlier article, we looked at different ways to capitalize the first letter of a string to uppercase in JavaScript. In this article, you'll learn how to uppercase or lowercase a string completely in JavaScript.
JavaScript provides two built-in functions for converting strings to uppercase and lowercase. Let us look at them.
toUpperCase()
Method
The toUpperCase()
method transforms a string to uppercase letters in JavaScript. It doesn't change the original string and returns a new string equivalent to the string converted to uppercase.
Here is an example:
const str = 'JavaScript World!';
// convert string to uppercase
const upperStr = str.toUpperCase();
// print the new string
console.log(upperStr);
// output: JAVASCRIPT WORLD!
toLowerCase()
Method
The toLowerCase()
method does the opposite of the toUpperCase()
method. It converts all characters of the string to lowercase:
const str = 'JavaScript World!';
// convert string to lowercase
const lowerStr = str.toLowerCase();
// print the new string
console.log(lowerStr);
// output: javascript world!
Both these methods work in all modern browsers and are supported back to at least Internet Explorer 6.
Are you looking for other code tips?
JS Nooby
Javascript connoisseur